Boost Your Dev Game: Faster Web Actors Unveiled!
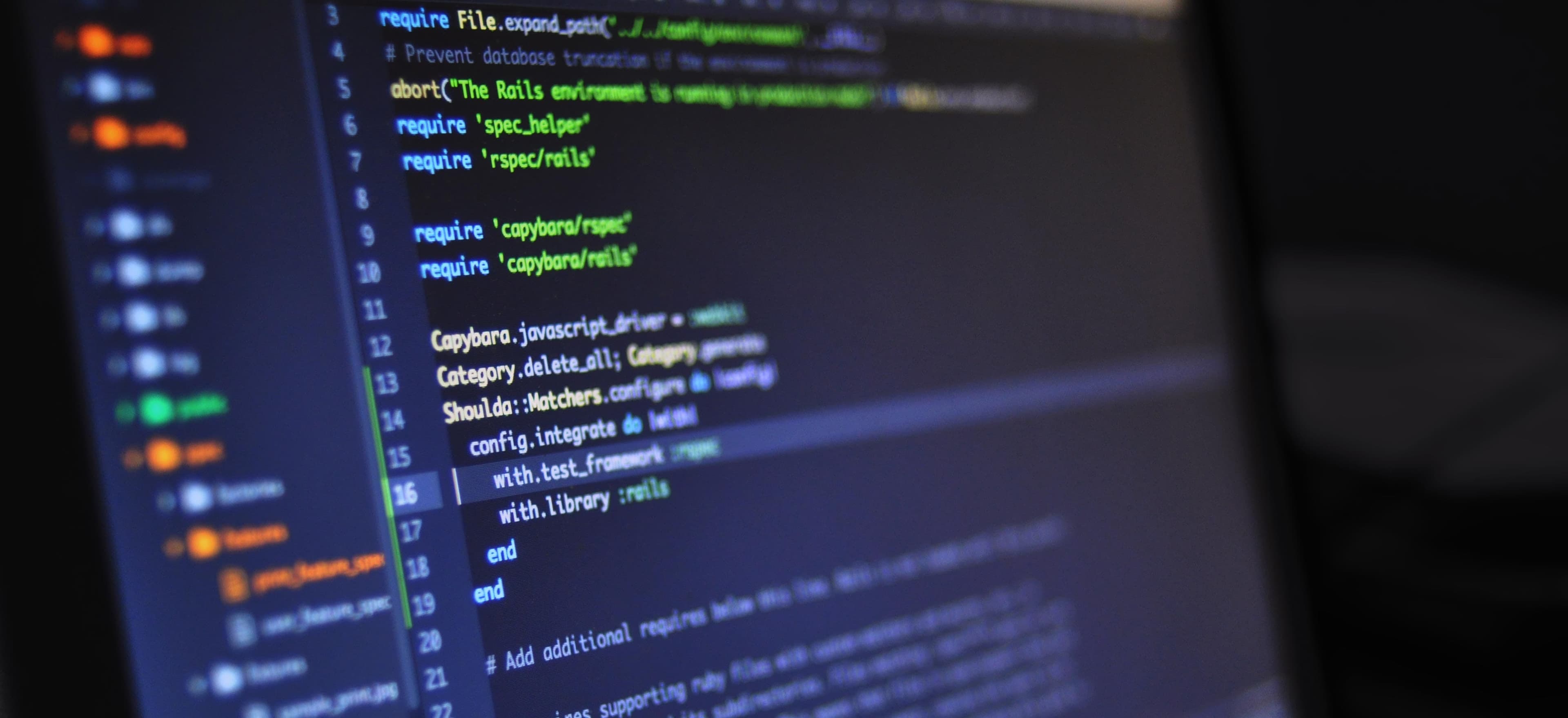
- Published on
Boost Your Dev Game: Faster Web Actors Unveiled!
As a Java developer, you’re no stranger to the ever-evolving world of web development. Your toolkit likely includes a host of Java libraries, frameworks, and design patterns to build robust and efficient web applications. And when it comes to managing concurrent, asynchronous tasks in your web applications, leveraging the actor model can be a game-changer.
In this post, we will delve into the world of web actors in Java, exploring how they can supercharge the performance of your web applications. We will discuss the basics of the actor model, showcase an example of web actors in action, and explore the benefits they offer. So, buckle up and get ready to take your web development expertise to the next level!
Understanding the Actor Model
The actor model is a powerful paradigm for building concurrent and distributed systems. At its core, the actor model revolves around the idea of actors - independent entities that encapsulate state, behavior, and communication. Actors communicate with each other by sending and receiving messages, allowing for seamless concurrency and parallelism without the need for locks or explicit synchronization mechanisms.
In the context of web development, web actors can revolutionize the way you handle concurrent operations within your applications. By allowing you to model individual components as independent actors, you can achieve high levels of concurrency and responsiveness, leading to more efficient and scalable web applications.
Getting Started with Web Actors in Java
Now that we’ve laid the groundwork, let’s dive into a practical example of implementing web actors in Java. To get started, you’ll need to leverage a powerful library that provides support for implementing the actor model. One such library is Akka, a toolkit for building highly concurrent, distributed, and resilient message-driven applications on the JVM.
First, ensure you have the Akka library added to your project's dependencies. You can do this by adding the following Maven dependency to your pom.xml
file:
<dependency>
<groupId>com.typesafe.akka</groupId>
<artifactId>akka-actor_2.12</artifactId>
<version>2.6.16</version>
</dependency>
Next, let’s create a simple web actor that simulates processing concurrent requests. Below is an example of a web actor that processes incoming messages:
import akka.actor.AbstractActor;
public class WebActor extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, message -> {
// Simulate processing
System.out.println("Processing message: " + message);
})
.build();
}
}
In this example, the WebActor
extends AbstractActor
and overrides the createReceive
method to define its message-handling behavior. When the actor receives a String
message, it simply logs the received message to simulate processing.
Leveraging the Power of Web Actors
Now that we have our web actor in place, let’s explore how we can utilize its power within a web application. Consider a scenario where you need to handle concurrent HTTP requests by dispatching them to web actors for processing. This can be achieved by integrating web actors with a lightweight web framework, such as Spark Java.
import static spark.Spark.*;
public class WebApplication {
public static void main(String[] args) {
// Create web actor instances
final ActorSystem actorSystem = ActorSystem.create("web-actors-system");
final ActorRef webActor1 = actorSystem.actorOf(Props.create(WebActor.class));
final ActorRef webActor2 = actorSystem.actorOf(Props.create(WebActor.class));
// Define HTTP routes
get("/process/request1", (req, res) -> {
webActor1.tell("Request 1 received", ActorRef.noSender());
return "Request 1 processed";
});
get("/process/request2", (req, res) -> {
webActor2.tell("Request 2 received", ActorRef.noSender());
return "Request 2 processed";
});
}
}
In this example, we create instances of the WebActor
within our web application and define HTTP routes that dispatch incoming requests to the respective web actors for processing. This setup allows for efficient handling of concurrent requests, as each request is processed independently by a dedicated web actor.
The Benefits of Web Actors in Java
By incorporating web actors into your Java web applications, you can unlock a myriad of benefits:
-
Concurrency: Web actors enable seamless concurrency, allowing your web application to efficiently handle multiple concurrent tasks without the complexity of traditional synchronization mechanisms.
-
Scalability: With web actors, you can easily scale your application to handle increased traffic by adding more actor instances, ensuring that each request is processed in isolation without impacting others.
-
Resilience: The actor model promotes fault isolation, making it easier to recover from failures within your web application without disrupting the entire system.
-
Simplicity: Web actors offer a clear and intuitive model for managing concurrent operations, leading to simpler code and easier maintenance.
To Wrap Things Up
In conclusion, web actors are a powerful tool in the arsenal of Java web developers, offering a scalable, resilient, and efficient approach to handling concurrent operations. By embracing the actor model, you can elevate the performance and responsiveness of your web applications, paving the way for a seamless user experience.
So, the next time you find yourself grappling with the complexities of concurrent tasks in your web applications, consider harnessing the incredible power of web actors. With the right tools and techniques at your disposal, you can take your web development prowess to new heights and deliver exceptional web experiences.
Ready to dive deeper into web actors? Check out the official Akka documentation for comprehensive insights into leveraging the actor model for building high-performance web applications. Happy coding!