Revolutionize Testing: JUnit's Secret to Loop-Free Repeats
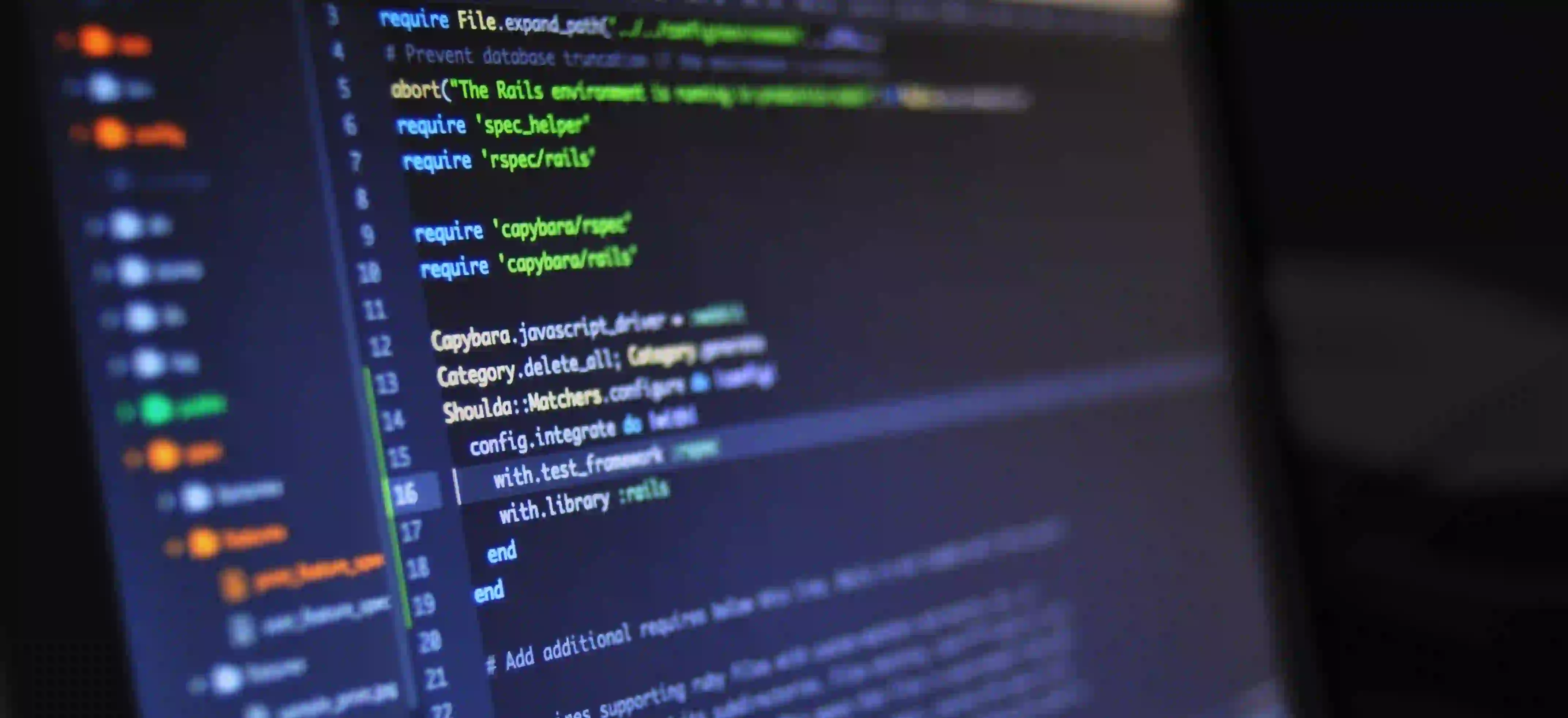
Revolutionize Testing: JUnit's Secret to Loop-Free Repeats
When it comes to Java and testing, JUnit stands tall as the go-to framework for developers worldwide. But what makes JUnit so powerful? This blog post delves into the lesser-known, yet incredibly potent feature of JUnit - parameterized tests. We will explore how parameterized tests can help in writing efficient, maintainable, and loop-free test code.
The Power of Parameterized Tests
JUnit's parameterized tests allow you to run the same test logic with multiple sets of parameters. This means you can create more thorough test cases without duplicating the test code. Let's consider an example where a method needs to be tested with different input parameters. Traditionally, one might create multiple test methods, each with a different set of input values. However, parameterized tests provide a smarter approach.
@RunWith(Parameterized.class)
public class MathUtilParameterizedTest {
private int input;
private boolean expected;
public MathUtilParameterizedTest(int input, boolean expected) {
this.input = input;
this.expected = expected;
}
@Parameterized.Parameters
public static Collection<Object[]> testConditions() {
return Arrays.asList(new Object[][] {
{0, true},
{2, true},
{3, false},
{6, true}
});
}
@Test
public void testIsEven() {
MathUtil mathUtil = new MathUtil();
assertEquals(expected, mathUtil.isEven(input));
}
}
In this example, the MathUtilParameterizedTest
class uses JUnit's Parameterized
runner to specify the input parameters and the expected outcome. The testConditions
method provides a collection of parameter sets, and the testIsEven
method tests the isEven
method of MathUtil
with each set of parameters.
By using parameterized tests, you reduce code duplication, improve readability, and set the stage for easy maintenance.
Why Parameterized Tests are Game-Changing
Efficiency
Parameterized tests reduce the need for duplicate test logic. With a single test method handling multiple parameter sets, you can achieve comprehensive test coverage without bloating your test suite.
Readability
By consolidating related tests into a single parameterized test, the test class becomes leaner and more coherent. This makes it easier for developers to grasp the intent of the test suite and locate specific test cases.
Maintainability
When the need arises to modify the test logic, a parameterized test requires changes in only one place, as opposed to multiple test methods. This ensures consistent behavior across all test cases and simplifies the maintenance process.
Guidelines for Writing Parameterized Tests
Keep It Simple
When using parameterized tests, it's essential to keep the parameter generation logic straightforward. Avoid complex parameter generation code to maintain the clarity and intent of the test.
Choose Appropriate Data Types
Carefully select data types for parameterized tests to cover various scenarios. Ensure that the chosen data types effectively represent the input parameters, minimizing the risk of incorrect test cases.
Leverage External Data Sources
In real-world scenarios, input parameters for tests might come from external sources such as property files or databases. JUnit supports the use of external sources for parameterized tests, enabling flexibility and maintainability.
Use with Theories
JUnit's @Theory
annotation complements parameterized tests by allowing the use of logical conditions to define expected outcomes. This further enhances the expressiveness and flexibility of your test suite.
A Final Look
JUnit's parameterized tests offer a powerful mechanism to streamline your testing efforts by eliminating redundant test logic. By embracing parameterized tests, you can achieve comprehensive test coverage, maintainable test suites, and efficient testing practices.
In conclusion, parameterized tests in JUnit provide a succinct, scalable, and reusable approach to testing, and mastering this feature can revolutionize your testing strategies.
So, the next time you find yourself writing repetitive test methods, remember - parameterized tests are the secret to loop-free repeats!
To delve deeper into the world of JUnit and testing in Java, check out our comprehensive guide on JUnit.
Don't forget to share your thoughts and experiences with parameterized tests in the comments below. Happy testing!