Unlocking Kannel Gateway: Master Java API for SMS Success
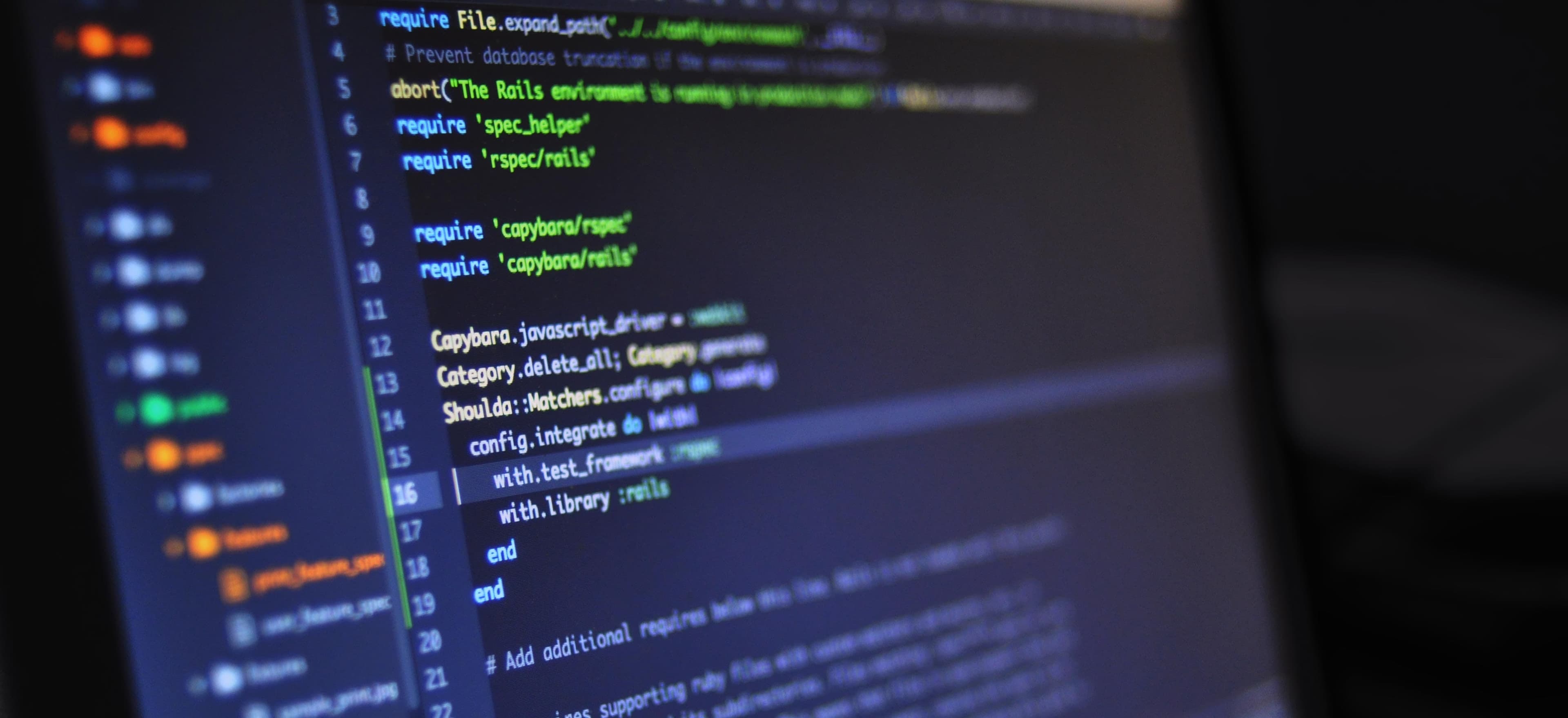
- Published on
Unlocking Kannel Gateway: Master Java API for SMS Success
Are you seeking a robust solution for integrating SMS capabilities into your Java application? Look no further than Kannel Gateway. In this post, we will explore how to leverage the Kannel Gateway Java API to seamlessly incorporate SMS functionality into your Java applications. We will cover the key features of Kannel Gateway, the benefits of using the Java API, and provide practical examples to demonstrate its usage.
What is Kannel Gateway?
Kannel is an open-source WAP and SMS gateway, designed to provide a flexible platform for SMS and WAP push service. It is widely used for building SMS-based applications, such as SMS gateways, bulk SMS services, and SMS notification systems. Kannel supports various protocols, including SMPP, HTTP, and GSM modems, making it a versatile tool for SMS communication.
Why Use the Java API for Kannel Gateway?
The Java API for Kannel Gateway offers a convenient and powerful way to interact with the Kannel SMS gateway. By using the Java API, developers can seamlessly integrate SMS functionality into their Java applications, leveraging the full capabilities of the Kannel Gateway. The Java API abstracts the complexities of communication protocols and provides a straightforward interface for sending and receiving SMS messages.
Getting Started with the Kannel Gateway Java API
To get started with the Kannel Gateway Java API, you need to ensure that Kannel Gateway is properly configured and running. Once the Kannel Gateway is set up, you can begin using the Java API to interact with the gateway programmatically.
Let's look at a simple example of sending an SMS message using the Kannel Gateway Java API:
import org.kannel.api.KannelSender;
public class SMSSender {
public static void main(String[] args) {
KannelSender sender = new KannelSender("http://kannel-server:port", "username", "password");
String recipient = "+1234567890";
String message = "Hello, this is a test message from Kannel Gateway!";
sender.sendMessage(recipient, message);
}
}
In this example, we create a KannelSender
instance by providing the URL of the Kannel server, along with the username and password for authentication. We then use the sendMessage
method to send an SMS message to a recipient with the specified message content.
The Kannel Gateway Java API simplifies the process of sending SMS messages, allowing developers to focus on the core functionality of their application.
Key Features of the Kannel Gateway Java API
The Kannel Gateway Java API provides a range of features to facilitate seamless integration of SMS functionality into Java applications:
- Simplified Communication: The Java API abstracts the complexities of communication protocols, making it easier to send and receive SMS messages.
- Authentication Support: The API supports authentication mechanisms, ensuring secure communication with the Kannel Gateway.
- Error Handling: Robust error handling capabilities are integrated into the API, allowing developers to handle communication issues gracefully.
- Asynchronous Communication: The API supports asynchronous communication, enabling non-blocking interactions with the Kannel Gateway.
By leveraging these features, developers can create reliable and efficient SMS-enabled applications with ease.
Example: Handling SMS Delivery Reports
In addition to sending SMS messages, the Kannel Gateway Java API allows for handling delivery reports to track the status of sent messages. Let's consider an example of how to handle SMS delivery reports using the Java API:
import org.kannel.api.KannelReceiver;
import org.kannel.api.SMSDeliveryReport;
public class SMSDeliveryReportHandler {
public static void main(String[] args) {
KannelReceiver receiver = new KannelReceiver("http://kannel-server:port", "username", "password");
SMSDeliveryReport[] deliveryReports = receiver.getDeliveryReports();
for (SMSDeliveryReport report : deliveryReports) {
System.out.println("Message ID: " + report.getMessageId());
System.out.println("Recipient: " + report.getRecipient());
System.out.println("Status: " + report.getStatus());
System.out.println("-----------------------");
}
}
}
In this example, we create a KannelReceiver
instance to retrieve delivery reports from the Kannel Gateway. We use the getDeliveryReports
method to fetch the delivery reports, and then iterate through the reports to display the message ID, recipient, and status of each report.
By incorporating delivery report handling into your Java application, you can gain insights into the status of sent SMS messages and ensure reliable message delivery.
A Final Look
In conclusion, the Kannel Gateway Java API offers a powerful and convenient way to integrate SMS functionality into Java applications. Whether you are building a notification system, a two-factor authentication solution, or a bulk SMS service, the Kannel Gateway Java API provides the tools you need to achieve SMS success.
By leveraging the features of the Java API, developers can streamline the integration of SMS capabilities, while benefiting from robust error handling, authentication support, and seamless communication with the Kannel Gateway.
If you're interested in diving deeper into Kannel Gateway and its Java API, be sure to check out the official documentation and explore the possibilities of incorporating SMS into your Java applications.
Unlock the potential of SMS communication in your Java applications with the Kannel Gateway Java API!
Checkout our other articles