Choosing Between @RestController and @Controller in Spring MVC
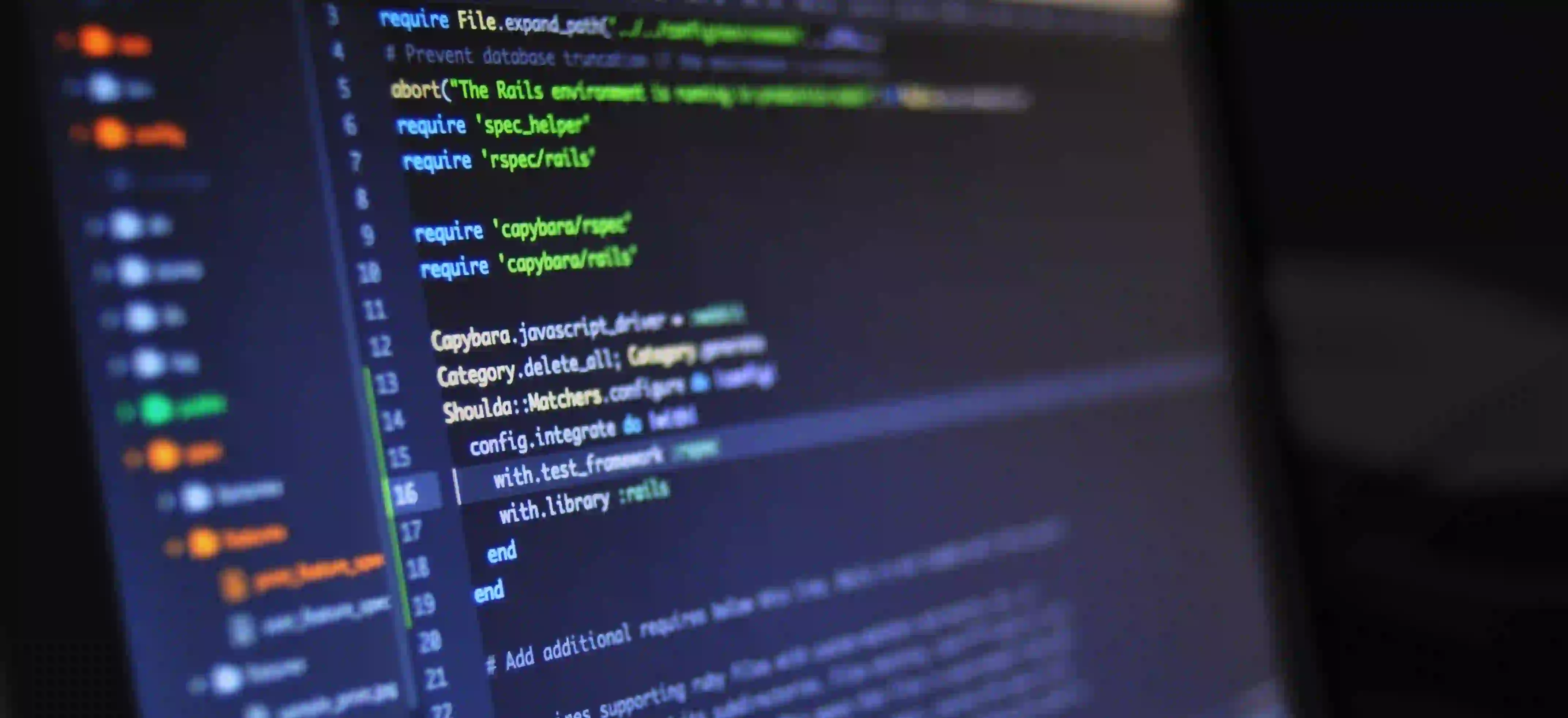
Understanding the Difference Between @RestController and @Controller in Spring MVC
When working with Spring MVC, it's essential to understand the distinction between the @RestController
and @Controller
annotations. Both play a critical role in handling HTTP requests and generating responses, but their use cases differ significantly.
In this article, we'll delve into the nuances of @RestController
and @Controller
, discussing their purposes, use cases, and the best scenarios to utilize each of them.
What is @RestController?
The @RestController
annotation in Spring MVC is a specialized version of the @Controller
annotation. It was introduced in Spring 4.0 to simplify the creation of RESTful web services. When a class is annotated with @RestController
, it's capable of handling HTTP requests and returning the response as JSON or XML data, without the need for explicit Jackson or JAXB annotations.
What is @Controller?
On the other hand, the @Controller
annotation is a fundamental component of the Spring MVC framework. It indicates that a particular class serves the role of a controller. When a method within a @Controller
class is invoked, the return value is resolved as a logical view name.
Use Cases
@RestController
The @RestController
annotation is ideal for scenarios where the primary goal is to build RESTful web services. It's suitable for exposing a collection of resources through a consistent API interface. Typically, the return value of the methods annotated with @RestController
is the actual response body, serialized into JSON or XML format.
@Controller
Conversely, the @Controller
annotation is more suitable for traditional web applications, where the focus is on rendering server-side HTML or processing form submissions. Methods within a @Controller
class often return the name of a view, which is resolved by a view resolver to generate the appropriate HTML response.
When to Use @RestController vs. @Controller
Use @RestController When:
- Building a RESTful web service
- Exposing JSON or XML responses
- Developing a stateless, client-agnostic API
Use @Controller When:
- Rendering server-side HTML views
- Handling form submissions
- Managing session state and server-side rendering
Example Code
Let's examine a simple example to demonstrate the differences between using @RestController
and @Controller
.
Consider a case where we want to handle a GET request for fetching a user's information.
Using @RestController
@RestController
@RequestMapping("/users")
public class UserRestController {
@GetMapping("/{userId}")
public User getUser(@PathVariable Long userId) {
// Retrieve user information from the database
return userService.getUserById(userId);
}
}
In this example, the UserRestController
annotated with @RestController
returns the User
object directly, which will be serialized into JSON for the client.
Using @Controller
@Controller
@RequestMapping("/users")
public class UserController {
@GetMapping("/{userId}")
public String getUser(@PathVariable Long userId, Model model) {
// Retrieve user information from the database
User user = userService.getUserById(userId);
model.addAttribute("user", user);
return "user-profile";
}
}
In this case, the UserController
annotated with @Controller
returns the logical view name "user-profile", and the server generates the corresponding HTML response.
Bringing It All Together
In summary, the decision to use @RestController
or @Controller
should align with the specific requirements of the application. While @RestController
is tailored for building RESTful web services and returning data in JSON or XML format, @Controller
is well-suited for traditional server-side rendering of web applications.
Understanding the distinction between the two annotations is crucial for effectively implementing the appropriate design pattern and ensuring the desired functionality of your Spring MVC application.
For further insights into Spring MVC and web application development, check out the official Spring documentation and Baeldung's comprehensive guide on Spring MVC.