The Pitfalls of Deploying Java Apps on AWS Lambda
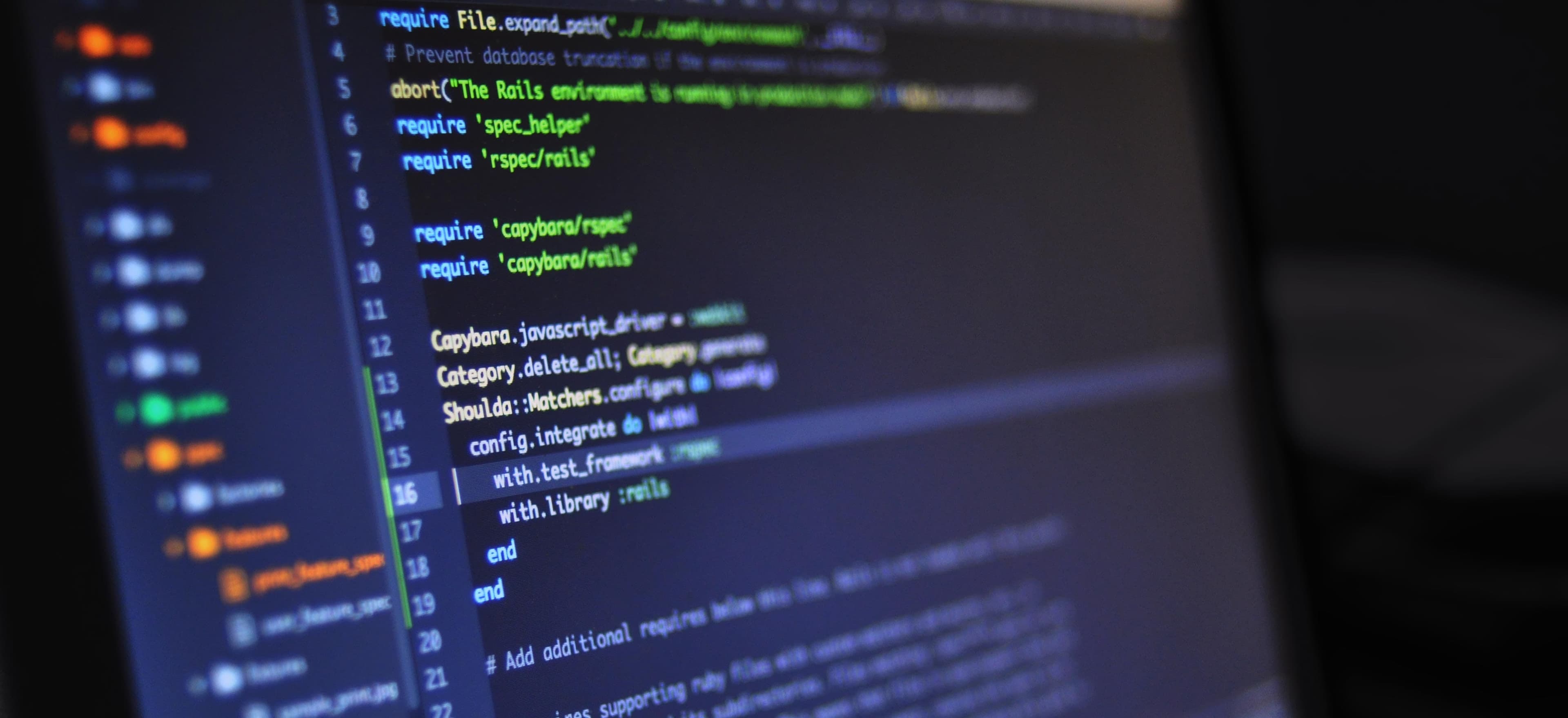
- Published on
Essentials at a Glance
When it comes to deploying Java applications on AWS Lambda, there are certain pitfalls that developers should be aware of. While AWS Lambda provides a powerful serverless computing platform, Java developers often encounter challenges when deploying their applications. In this article, we'll explore some common pitfalls and how to overcome them when deploying Java apps on AWS Lambda.
Cold Start Performance
One of the most significant challenges when deploying Java applications on AWS Lambda is the cold start performance. When a Lambda function is invoked for the first time or after a period of inactivity, AWS must initialize the Java Virtual Machine (JVM) and load the application, resulting in longer invocation times.
To mitigate this issue, developers can employ several strategies such as using lightweight Java frameworks like Micronaut or Quarkus, optimizing the application's startup code, and employing techniques to keep the JVM warm, such as using scheduled pings or leveraging Lambda Provisioned Concurrency.
Example of Optimizing Startup Code
public class Handler implements RequestHandler<Request, Response> {
static {
// Perform any expensive initialization during class loading
}
public Response handleRequest(Request request, Context context) {
// Handler logic
}
}
In this example, the static block can be used to perform any costly initialization during class loading, thereby reducing startup time during Lambda invocation.
Memory Allocation
Another common pitfall when deploying Java apps on AWS Lambda is inefficient memory allocation. Since AWS Lambda allocates memory in 64 MB increments, Java developers must optimize memory usage to ensure efficient and cost-effective execution of their functions.
Developers can use Java memory profiling tools like VisualVM or YourKit to identify memory-intensive operations and optimize memory allocation. Additionally, employing efficient data structures and minimizing object creation can help reduce memory overhead.
Example of Optimizing Memory Allocation
public class MemoryOptimizedHandler implements RequestHandler<Request, Response> {
public Response handleRequest(Request request, Context context) {
// Use primitive data types instead of object wrappers to reduce memory usage
int result = 0;
for (int i = 0; i < 1000; i++) {
result += i;
}
// Handler logic
}
}
In this example, the use of primitive data types instead of object wrappers reduces memory overhead and improves the efficiency of the Lambda function.
Jar Size Limitations
AWS Lambda has a limitation on the size of the deployment package, with a maximum uncompressed size of 250 MB. Java applications with large dependencies or resources may exceed this limit, causing deployment failures.
To overcome this limitation, developers can employ techniques such as using lightweight dependencies, minimizing resource files, and employing tools like ProGuard to remove unused code and optimize the size of the deployment package.
Example of Optimizing Jar Size
<build>
<plugins>
<plugin>
<groupId>com.github.wvengen</groupId>
<artifactId>proguard-maven-plugin</artifactId>
<version>2.0.14</version>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>proguard</goal>
</goals>
</execution>
</executions>
<configuration>
<injar>${project.build.finalName}.jar</injar>
<outjar>${project.build.finalName}-min.jar</outjar>
<proguardVersion>6.2.2</proguardVersion>
<options>
<option>-allowaccessmodification</option>
<option>-dontoptimize</option>
<option>-keep class * {
public static void main(java.lang.String[]);
}
</option>
</options>
</configuration>
</plugin>
</plugins>
</build>
In this example, the ProGuard Maven plugin is used to remove unused code and optimize the size of the deployment package, ensuring it fits within the AWS Lambda size limitations.
Closing the Chapter
Deploying Java applications on AWS Lambda offers the benefits of serverless computing, but it comes with its own set of challenges. By being mindful of cold start performance, memory allocation, and jar size limitations, developers can successfully navigate the pitfalls and optimize their Java apps for efficient execution on AWS Lambda.
In conclusion, while AWS Lambda provides a powerful platform for serverless Java applications, it's critical to be aware of these pitfalls and employ best practices to ensure smooth deployment and optimal performance.
For further reading on Java optimization on AWS Lambda, I recommend checking out AWS Lambda's official documentation on best practices for Java and the AWS Java SDK. These resources provide valuable insights into optimizing Java applications for AWS Lambda.
Checkout our other articles