Unlocking Concurrency: Atomic Operations in Java
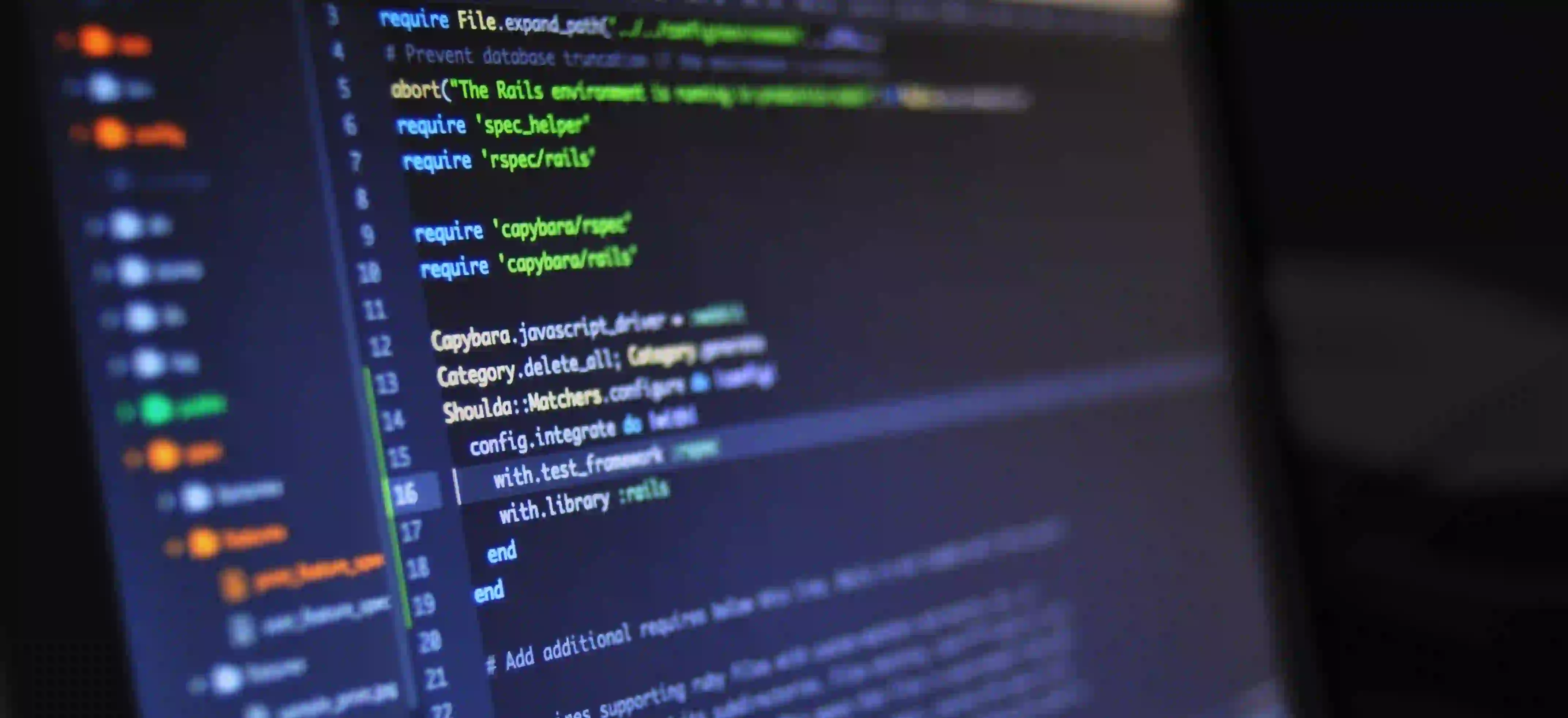
Unlocking Concurrency: Atomic Operations in Java
Concurrency in Java is a powerful feature that allows multiple tasks to execute simultaneously, improving performance and responsiveness. However, it can also introduce complexities such as data consistency issues when multiple threads access and modify shared data concurrently. This is where atomic operations play a crucial role in maintaining data integrity and avoiding race conditions. In this article, we'll explore the concept of atomic operations in Java, understand their importance, and learn how to leverage them effectively.
Understanding Atomic Operations
In the context of concurrent programming, an operation is said to be atomic if it is guaranteed to be executed as a single, indivisible unit. This means that when multiple threads are simultaneously performing the operation, it will appear as if the operations are executed in isolation, without any interference from other threads.
In Java, the java.util.concurrent.atomic
package provides various classes that support atomic operations, such as:
AtomicInteger
AtomicLong
AtomicReference
AtomicBoolean
These classes offer atomic versions of respective primitive types and references, allowing thread-safe operations without the need for explicit synchronization.
Why Atomic Operations Matter
Consider a scenario where multiple threads are incrementing a shared integer variable. Without synchronization or atomic operations, the following code snippet could lead to unexpected results due to data races:
class SharedResource {
private int counter;
public void increment() {
counter++;
}
}
In this example, if multiple threads concurrently call the increment
method, the shared counter
variable could end up with an incorrect value due to race conditions. This is where atomic operations shine, as they provide a safe and efficient way to perform such operations without the risk of data corruption.
Leveraging Atomic Operations in Java
Let's rewrite the previous example using AtomicInteger
to ensure atomic increments:
import java.util.concurrent.atomic.AtomicInteger;
class SharedResource {
private AtomicInteger counter = new AtomicInteger(0);
public void increment() {
counter.incrementAndGet();
}
}
In this refactored code, the incrementAndGet
method in AtomicInteger
ensures that the increment operation is atomic, allowing multiple threads to safely modify the counter
variable without the need for explicit locking or synchronization.
Atomic Operations and Compare-and-Swap (CAS)
Under the hood, atomic classes in Java often utilize Compare-and-Swap (CAS) operations to achieve atomicity. CAS is a low-level concurrency control mechanism widely used in multi-threaded environments. It involves comparing the current value of a variable with an expected value and, if they match, replacing the variable's value with a new value. This process is done atomically and provides a way to avoid traditional locks and their associated problems like deadlock and contention.
The AtomicInteger
class, for instance, uses CAS operations for atomic increments and updates. This allows for efficient and non-blocking concurrency control, making it a powerful tool for building high-performance concurrent applications.
Handling Complex Operations Atomically
In addition to simple operations like increments and updates, atomic operations can also be used to compose complex operations atomically. For example, consider the need to update a set of related variables in a thread-safe manner. This is where atomic compound actions, such as those provided by AtomicReference
, come into play.
import java.util.concurrent.atomic.AtomicReference;
class ComplexResource {
private AtomicReference<String> data = new AtomicReference<>("initial");
public void updateData(String newValue) {
String existingValue;
String combinedValue;
do {
existingValue = data.get();
combinedValue = existingValue + newValue;
} while (!data.compareAndSet(existingValue, combinedValue));
}
}
In this example, the updateData
method atomically updates the data
by appending a new value to the existing value. The compareAndSet
method ensures that the update is performed atomically, preventing interference from other threads.
When to Use Atomic Operations
Atomic operations are valuable in scenarios where traditional synchronization techniques like locks or synchronized
blocks are either too coarse-grained or too performance-intensive. They are particularly beneficial for fine-grained operations on shared variables, where the overhead of locking mechanisms can impact scalability and responsiveness.
It's important to note that while atomic operations provide a lightweight alternative to traditional locking, they are not a one-size-fits-all solution. Understanding the specific concurrency requirements of your application is crucial in determining whether atomic operations are the most appropriate choice.
The Closing Argument
In the world of concurrent programming, maintaining data consistency and avoiding race conditions are essential for building robust and scalable applications. Atomic operations in Java provide a concise and efficient way to achieve thread-safe, lock-free operations on shared variables. By leveraging atomic classes and their underlying mechanisms like CAS, developers can harness the power of concurrency without sacrificing data integrity or performance.
To delve deeper into the topic, I recommend exploring the official Java documentation on java.util.concurrent.atomic for a comprehensive overview of atomic classes and their usage.
In conclusion, mastering atomic operations equips you with a valuable toolset for conquering concurrency challenges and elevating the performance of your Java applications. Happy coding!