Unlocking GraalVM Power: A Beginners Guide to Faster Java
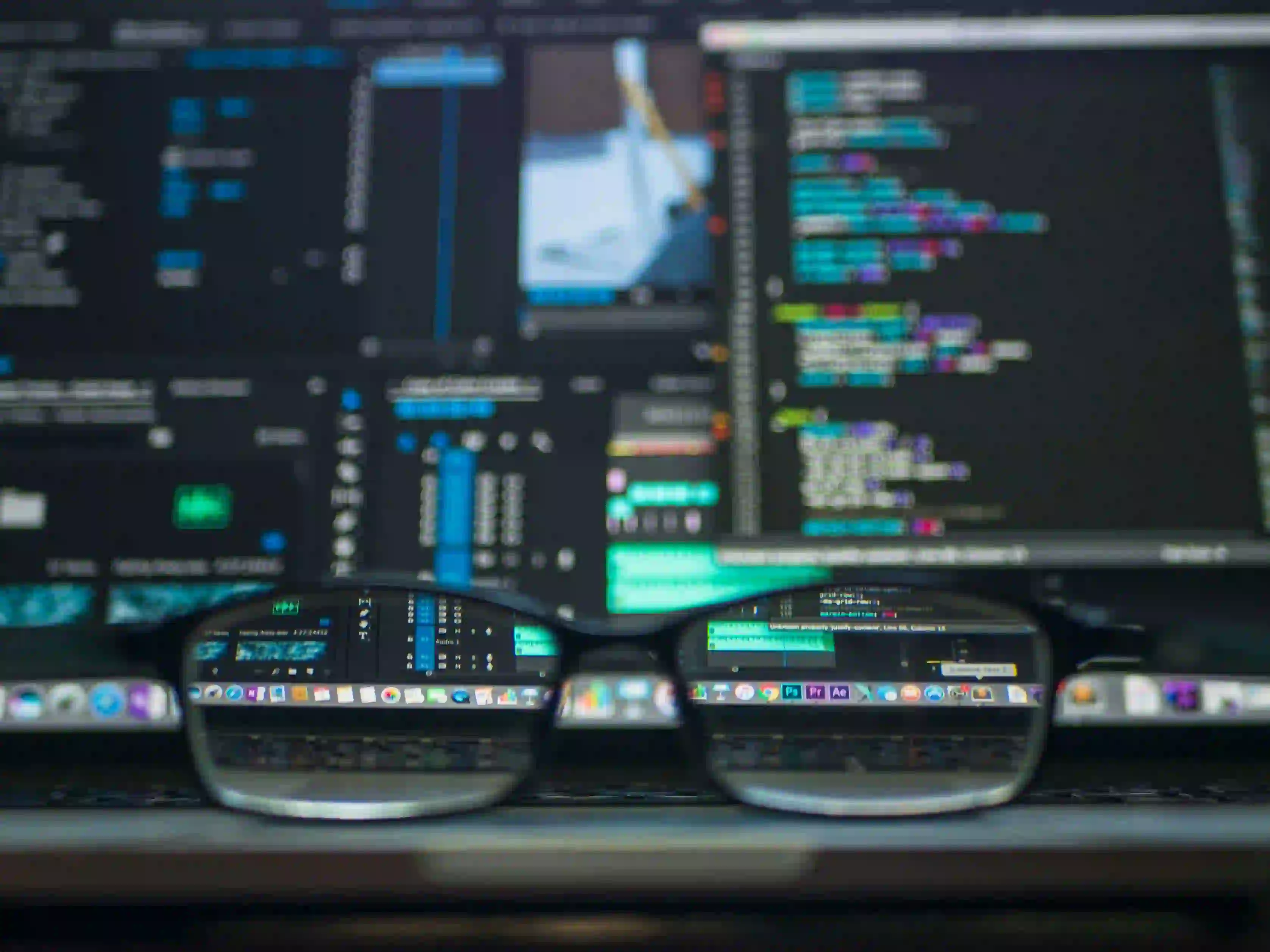
Unlocking GraalVM Power: A Beginners Guide to Faster Java
Are you looking to turbocharge your Java applications? Look no further than GraalVM – a high-performance runtime that offers impressive speed, reduced memory consumption, and better polyglot support. In this post, we’ll dive into the exciting world of GraalVM and demonstrate how it can elevate the performance of your Java programs. Whether you're a seasoned Java developer or just starting your journey, this guide will provide you with a solid understanding of GraalVM and how to leverage its capabilities to optimize your Java applications.
What is GraalVM?
GraalVM is a universal virtual machine that supports multiple languages and execution modes, including Just-In-Time (JIT) compilation, Ahead-Of-Time (AOT) compilation, and a polyglot environment that enables seamless integration of languages such as Java, JavaScript, Python, Ruby, and R. Its ability to run on various platforms, including macOS, Linux, and Windows, makes it a versatile tool for developers across different ecosystems.
With GraalVM, developers can benefit from its advanced optimizing compiler, which is built using the GraalVM compiler toolkit. This compiler can increase the performance of both Just-In-Time and Ahead-Of-Time compilation, resulting in faster execution of applications and reduced memory footprint.
Getting Started with GraalVM
Before we can explore the benefits of GraalVM for Java, let's set it up and run a simple Java program using GraalVM's Just-In-Time compilation.
Step 1: Installing GraalVM
You can download GraalVM from the official website here. Choose the version appropriate for your operating system and follow the installation instructions provided.
Step 2: Running a Java Program with GraalVM
Now that GraalVM is installed, let's create a simple Java program and run it using GraalVM's JIT compilation.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, GraalVM!");
}
}
Save the above code in a file named HelloWorld.java
and execute the following commands in your terminal:
javac HelloWorld.java
java HelloWorld
By default, the Java program is executed using the system's default JVM. Now, let's use GraalVM to run the program with JIT compilation:
native-image -cp . HelloWorld
./helloworld
The native-image
command compiles the Java program into a native executable using GraalVM's Ahead-Of-Time compilation, resulting in improved startup time and reduced memory overhead.
Leveraging GraalVM for Faster Java Programs
Now that we have seen how to run a simple Java program using GraalVM, let's explore some techniques to harness the power of GraalVM for optimizing Java applications.
1. Ahead-Of-Time Compilation
One of the key features of GraalVM is its ability to perform Ahead-Of-Time compilation, which translates Java bytecode into a standalone native executable. This eliminates the need for a separate JVM during execution, resulting in faster startup time and reduced memory consumption. To utilize AOT compilation with GraalVM, you can use the native-image
command as demonstrated in the previous section.
2. Just-In-Time Compilation
GraalVM's advanced optimizing compiler enhances the performance of Just-In-Time compilation by applying aggressive optimizations to the Java bytecode. As a result, Java applications running on GraalVM exhibit improved runtime performance and reduced memory footprint compared to traditional JVMs.
3. Polyglot Capabilities
GraalVM's polyglot environment allows seamless interoperability between different programming languages. This means that you can leverage the strengths of multiple languages within the same application, whether it's integrating existing Python or JavaScript code with your Java application or implementing performance-critical components in languages like C or C++.
4. Native Image Configuration
When using GraalVM's native-image utility, you can fine-tune various configurations to optimize the generated native executable for your specific application. This includes specifying resource configurations, reflection usage, and image build parameters to achieve the best performance for your Java application.
Best Practices for Optimizing Java Applications with GraalVM
To ensure that you are making the most of GraalVM's capabilities for your Java applications, consider the following best practices:
1. Profile-guided Optimization
By analyzing the execution profile of your Java application, you can identify hotspots and performance bottlenecks. Leveraging GraalVM's profile-guided optimization techniques allows you to tailor the AOT and JIT compilation strategies to optimize the critical parts of your application.
2. Choose the Right Compilation Mode
Depending on the nature of your Java application, you can choose to use GraalVM's AOT compilation for standalone native executables or rely on JIT compilation for Just-In-Time performance improvements. Understanding the trade-offs between these compilation modes is essential for maximizing the performance benefits of GraalVM.
3. Explore Polyglot Integration
Take advantage of GraalVM's polyglot capabilities to integrate existing code written in other languages seamlessly with your Java application. This can be particularly beneficial for scenarios where specific tasks are better suited for a language other than Java, such as data manipulation in Python or complex UI logic in JavaScript.
4. Continuous Performance Monitoring
Regularly monitor the performance of your Java application running on GraalVM to identify any regressions or opportunities for further optimization. This can involve using profiling tools, benchmarking suites, or performance monitoring utilities provided by GraalVM to gain insights into the runtime behavior of your applications.
The Last Word
GraalVM is a game-changer for Java developers seeking to unlock higher performance and improved efficiency in their applications. By harnessing the power of GraalVM's advanced compilation techniques, polyglot support, and performance optimizations, you can elevate the speed and resource utilization of your Java programs. Whether you are building microservices, serverless applications, or performance-critical systems, GraalVM is a formidable ally in your quest for faster, more efficient Java applications.
In this guide, we've covered the fundamentals of GraalVM, set up a basic Java program with GraalVM, and explored best practices for optimizing Java applications using GraalVM's capabilities. By following these principles and leveraging the full potential of GraalVM, you can take your Java applications to new heights of performance and efficiency.
So, what are you waiting for? Dive into GraalVM and unleash the full potential of your Java applications!
Now that you’ve finished this guide, check out this detailed comparison between GraalVM and OpenJDK to further enrich your understanding of GraalVM and its impact on Java development.