Overcoming JNDI Database Pool Challenges in Tomcat & Spring
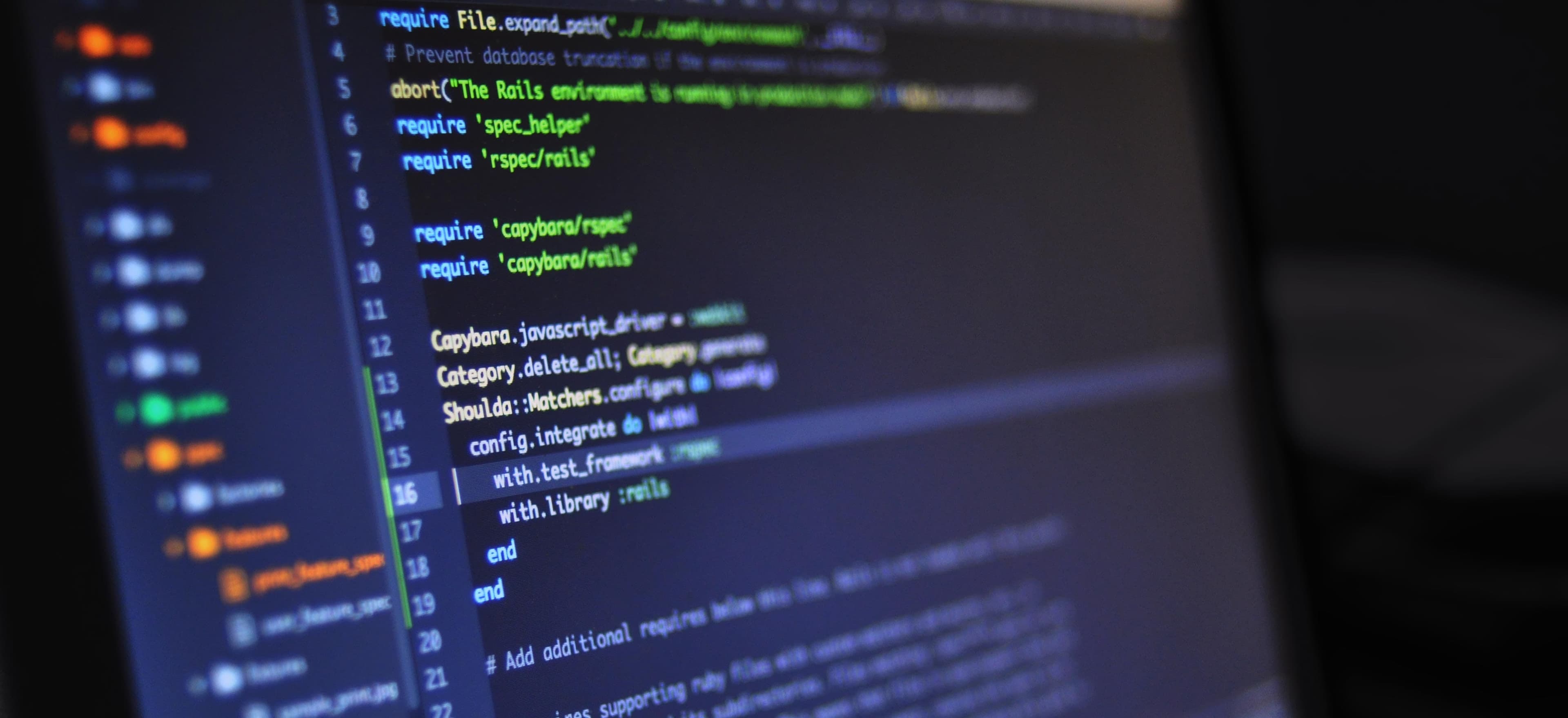
- Published on
Overcoming JNDI Database Pool Challenges in Tomcat & Spring
In Java web development, setting up and managing database connections is crucial for application performance and reliability. One widely-used technique for achieving this is to configure a database connection pool using JNDI (Java Naming and Directory Interface) in Tomcat, and accessing it in a Spring application.
In this article, we will explore the challenges developers may face when setting up a JNDI database pool in Tomcat and using it in a Spring application, and discuss best practices for overcoming these challenges.
Understanding JNDI and Database Connection Pools
JNDI provides a naming and directory service that allows Java objects to be stored and looked up. When used in the context of database connection pools, JNDI allows us to create a pool of pre-configured database connections that can be accessed by various components of an application.
Tomcat, a popular Java application server, provides a built-in JNDI mechanism for managing resources such as database connections. Spring, on the other hand, is a powerful and widely used framework for building Java applications, including web applications.
Configuring a JNDI Database Pool in Tomcat
To configure a JNDI database pool in Tomcat, you need to define a Resource
element in Tomcat's context configuration file (context.xml
). This Resource
element specifies the details of the database connection pool, such as the JDBC URL, username, password, and other configuration parameters.
Here's an example of configuring a JNDI database pool for a MySQL database in Tomcat's context.xml
:
<Context>
<Resource name="jdbc/mydb" auth="Container" type="javax.sql.DataSource"
maxTotal="100" maxIdle="30" maxWaitMillis="10000"
username="dbuser" password="dbpassword"
driverClassName="com.mysql.cj.jdbc.Driver"
url="jdbc:mysql://localhost:3306/mydb"/>
</Context>
In this example, we define a javax.sql.DataSource
named jdbc/mydb
with various configuration attributes such as maximum total connections, maximum idle connections, and the database connection URL.
Accessing the JNDI Database Pool in a Spring Application
Once the JNDI database pool is configured in Tomcat, we can access it in a Spring application using the JndiObjectFactoryBean
provided by Spring. This bean is used to lookup the JNDI resource and obtain a reference to the database connection pool.
Here's an example of accessing the JNDI database pool in a Spring application context configuration file:
<bean id="dataSource" class="org.springframework.jndi.JndiObjectFactoryBean">
<property name="jndiName" value="java:comp/env/jdbc/mydb"/>
</bean>
In this example, we define a dataSource
bean using JndiObjectFactoryBean
and specify the JNDI name of the database pool (jdbc/mydb
).
Challenges and Best Practices
Challenge 1: Handling Connection Leaks
One common challenge when using database connection pools is handling connection leaks. If a database connection is not properly closed after its use, it can lead to a connection leak, eventually exhausting the available connections in the pool.
Best Practice: Use try-with-resources or try-finally
When obtaining a database connection from the pool, always ensure that it is properly closed after its use. In Java 7 and later, you can use the try-with-resources statement to automatically close the connection after the code block is executed. Prior to Java 7, you can use a try-finally block to ensure proper closing of the connection.
Challenge 2: Managing Connection Pool Configuration
Configuring the connection pool parameters such as maximum total connections, maximum idle connections, and timeout values is critical for optimal performance and resource utilization.
Best Practice: Continuously Monitor and Adjust Configuration
Regularly monitor the application's database connection pool usage and performance metrics to determine if the configuration parameters need adjustment. Tools like HikariCP provide insightful metrics and monitoring capabilities for fine-tuning the connection pool configuration.
Final Thoughts
In this article, we explored the process of setting up a JNDI database pool in Tomcat and accessing it in a Spring application, discussed common challenges developers face when working with JNDI database pools, and recommended best practices for overcoming these challenges.
By following best practices such as proper connection handling and continuous monitoring and adjustment of connection pool configuration, developers can ensure the efficient and reliable operation of database connection pools in their Java web applications.
In conclusion, configuring and utilizing a JNDI database pool in Tomcat with a Spring application can be a powerful and efficient way to manage database connections, provided that the developers are aware of common challenges and best practices for addressing them.
For further reading on JNDI, Tomcat, and Spring, we recommend the official documentation for Tomcat JNDI Resources and Spring Framework.