Unlocking Solutions: 5 Strategies to Solve Code Deadlocks
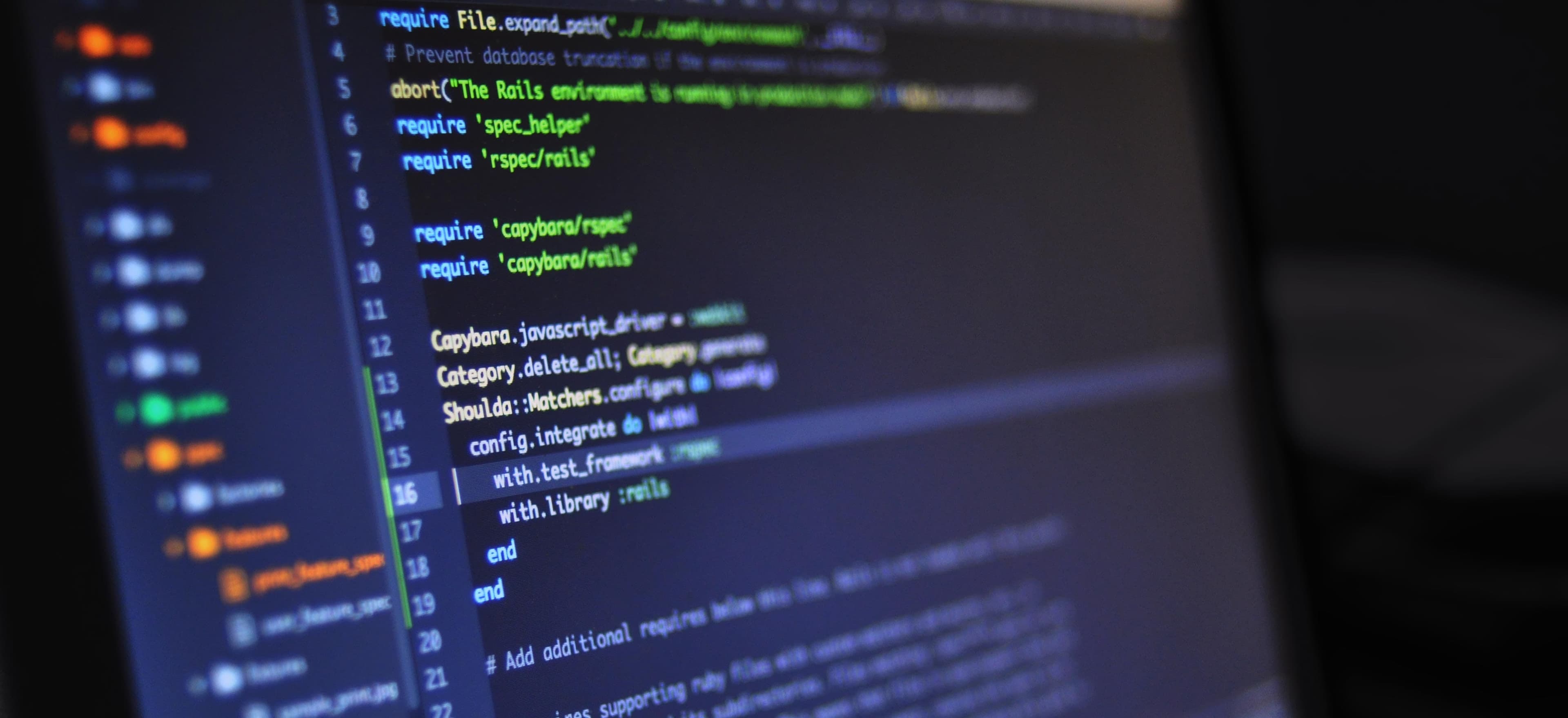
- Published on
Unlocking Solutions: 5 Strategies to Solve Code Deadlocks
Deadlocks in Java can be a developer's worst nightmare. They occur when two or more threads are blocked forever, each waiting for the other to release a resource. Identifying and solving deadlocks is crucial for maintaining a stable and responsive application. In this post, we will explore five effective strategies to tackle and prevent deadlocks in Java.
Understanding Deadlocks
Before delving into solutions, let's understand the nature of deadlocks. Deadlocks occur when four conditions are simultaneously met:
- Mutual Exclusion: At least one resource is non-shareable, meaning only one process can use it at a time.
- Hold and Wait: Processes already holding resources may request new ones without releasing the current ones.
- No Preemption: Resources cannot be forcibly taken from the process holding them; they can only be released voluntarily.
- Circular Wait: There exists a circular waiting chain, where Process A is waiting for a resource held by Process B, which in turn is waiting for a resource held by Process A.
Strategy 1: Avoid Nested Locks
One common cause of deadlocks is nested locks. When a thread holds one lock and then tries to acquire another while still holding the first, it can lead to a deadlock situation. To avoid this, always acquire locks in the same order across all threads. By following this practice, you reduce the likelihood of circular wait conditions, thereby preventing deadlocks.
synchronized void method1() {
// Critical section
synchronized (lock2) {
// Nested lock - can lead to deadlock
}
}
synchronized void method2() {
// Critical section
synchronized (lock2) {
// Acquiring locks in the same order
}
}
Strategy 2: Timeout Mechanism
Introducing a timeout mechanism while acquiring locks can help mitigate deadlocks. By specifying a maximum time to wait for a lock, you allow the system to recover from potential deadlock situations. If a lock cannot be acquired within the specified time, a thread can release all locks and reattempt the operation, preventing it from being stuck indefinitely.
synchronized void method1() {
try {
if (lock2.tryLock(10, TimeUnit.SECONDS)) {
// Critical section
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
} finally {
lock2.unlock();
}
}
Strategy 3: Resource Ordering
Another effective approach is to define a global order for acquiring locks on multiple resources. By ensuring that all threads acquire locks in the same order, you eliminate the possibility of circular wait conditions. This strategy requires a thorough understanding of the application's resource dependencies and meticulous planning to establish a consistent lock acquisition order.
Strategy 4: Deadlock Detection and Recovery
Implementing deadlock detection algorithms can help identify and recover from deadlock scenarios. One such approach is to periodically check for circular wait conditions and force release of resources to break the deadlock. While this strategy can incur additional overhead, it provides a safety net for handling complex deadlock scenarios.
if (Thread.holdsLock(lock1) && Thread.holdsLock(lock2)) {
// Detected deadlock - release resources
lock1.unlock();
lock2.unlock();
// Recovery mechanism
}
Strategy 5: Using ReentrantLock and Condition
Java provides the ReentrantLock
class, which offers more flexibility and control over locks compared to synchronized blocks. Along with Condition
objects, ReentrantLock
allows for finer-grained control of lock acquisition and release, enabling advanced deadlock prevention strategies such as timed waiting and signal-based notifications.
ReentrantLock lock = new ReentrantLock();
Condition condition = lock.newCondition();
lock.lock();
try {
while (conditionNotMet) {
condition.await(10, TimeUnit.SECONDS);
}
// Critical section
} finally {
lock.unlock();
}
The Bottom Line
Deadlocks are a critical issue in multi-threaded applications, but with the right strategies, they can be effectively prevented and resolved. By understanding the fundamental conditions that lead to deadlocks and applying proactive measures such as avoiding nested locks, implementing timeout mechanisms, defining resource ordering, employing deadlock detection and recovery, and using advanced locking mechanisms provided by Java, developers can ensure the stability and reliability of their applications.
Incorporating these strategies not only enhances the robustness of code but also fosters a deeper understanding of concurrency and resource management, paving the way for more resilient and efficient Java applications.
Remember, prevention is key, but being equipped with the knowledge to tackle deadlocks when they occur is equally crucial in the world of Java programming.
Learning how to effectively solve and prevent code deadlocks can save you invaluable time and effort, and ensure the smooth functioning of your Java applications.
Checkout our other articles