Tackling Complexity in Spring Integration Java DSL
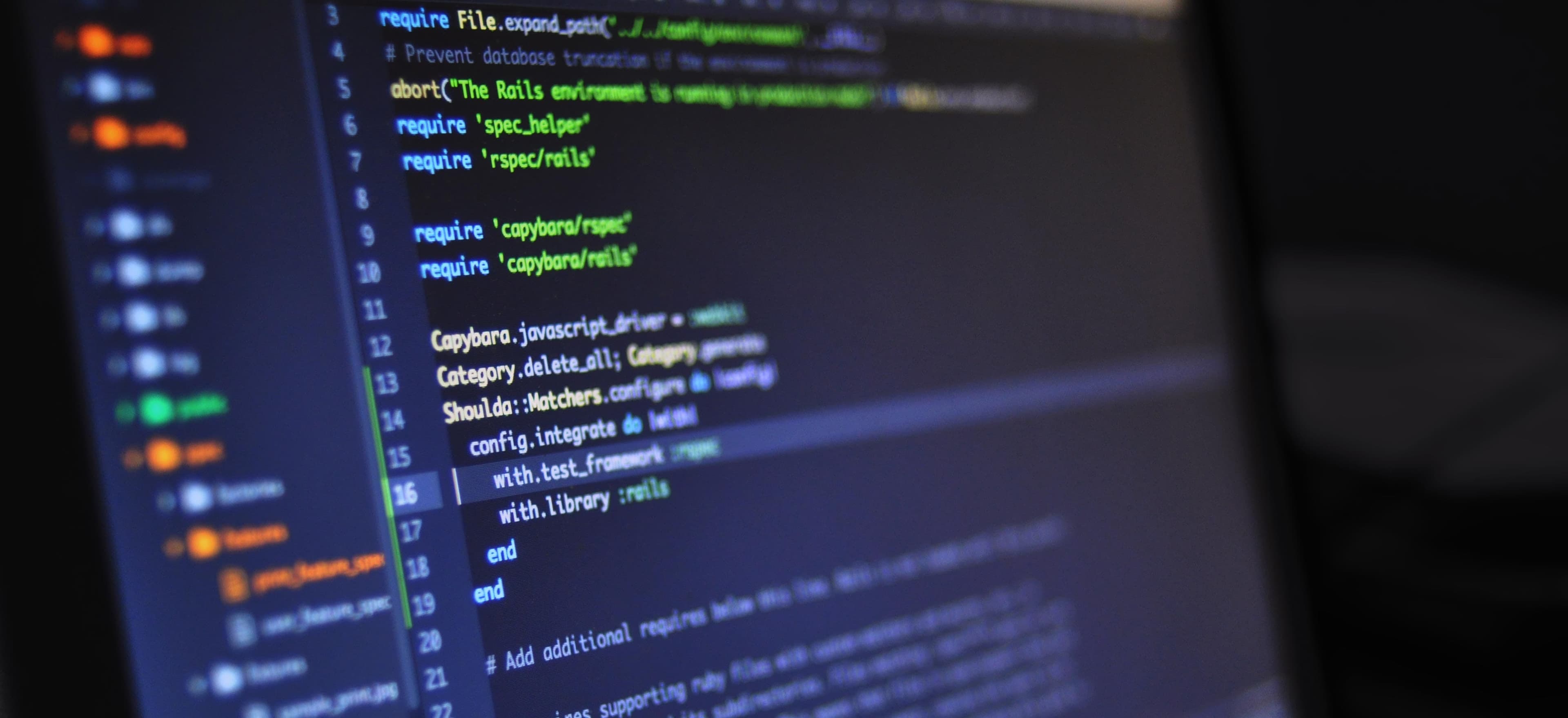
- Published on
Tackling Complexity in Spring Integration Java DSL
When it comes to modern Java application development, the Spring Framework is a powerhouse. It provides a comprehensive set of tools and libraries for building enterprise-grade applications. One of the essential components of the Spring Framework is Spring Integration, which facilitates the implementation of enterprise integration patterns (EIP) using a simple and lightweight messaging framework.
In this article, we'll delve into the complexities developers often encounter when working with Spring Integration and how the Java DSL (Domain Specific Language) can be used to simplify and streamline the integration process.
Understanding the Challenge
Traditional XML configuration for Spring Integration can sometimes result in verbose and hard-to-maintain code. While powerful, it can lead to a steep learning curve for developers trying to understand and modify the configuration. This issue becomes more pronounced as the complexity of the integration grows. Moreover, the XML configuration lacks the type safety and autocompletion provided by modern IDEs, making refactoring and debugging a cumbersome task.
In response to these challenges, Spring Integration offers a Java DSL, which allows developers to configure integration flows using a fluent, Java-based API. The Java DSL aims to address the limitations of XML configuration, providing a more straightforward and type-safe way to define integration components.
Simplifying Integration with Java DSL
Let's dive into an example to illustrate the simplicity and elegance of Spring Integration Java DSL. Suppose we have a requirement to build an integration flow that reads messages from a JMS queue, processes them, and then sends the processed messages to a file. Here's how we can achieve this using the Java DSL.
Configuring the Integration Flow
@Configuration
@EnableIntegration
public class IntegrationConfig {
@Bean
public IntegrationFlow jmsToFileFlow(JmsTemplate jmsTemplate, MessageChannel jmsInputChannel) {
return IntegrationFlows.from(jmsInputChannel)
.handle(Jms.outboundAdapter(jmsTemplate).destination("outputQueue"))
.get();
}
}
In this example, we create an IntegrationFlow
bean that represents the integration flow. We use IntegrationFlows
to define the flow, starting with an input channel (jmsInputChannel
) and then processing the messages using the handle
method.
Why Use Java DSL
By using the Java DSL, we benefit from the type safety and auto-completion provided by modern IDEs, making it easier to navigate and understand the integration flow. Additionally, the fluent API of the Java DSL enhances the readability of the code and reduces the verbosity typically associated with XML configuration.
Handling Errors and Retries
Error handling and retry mechanisms are critical aspects of any integration flow. With the Java DSL, we can seamlessly integrate error handling and retry logic into our integration flows, making them more robust and resilient.
Let's consider a scenario where we want to handle errors that occur during message processing and retry the processing multiple times before moving the message to a dead-letter queue.
Handling Errors and Retries
@Configuration
@EnableIntegration
public class IntegrationConfig {
@Bean
public IntegrationFlow jmsErrorHandlingFlow(JmsTemplate jmsTemplate, MessageChannel jmsInputChannel) {
return IntegrationFlows.from(jmsInputChannel)
.handle((GenericHandler<String>) (payload, headers) -> {
// Process the incoming message
// If an error occurs, throw an exception
}, e -> e.advice(retryAdvice()))
.channel(c -> c.errorHandler(errorHandler -> errorHandler.deadLetterChannel("deadLetterChannel")))
.get();
}
private RequestHandlerRetryAdvice retryAdvice() {
RequestHandlerRetryAdvice retryAdvice = new RequestHandlerRetryAdvice();
retryAdvice.setRetryTemplate(retryTemplate());
return retryAdvice;
}
private RetryTemplate retryTemplate() {
RetryTemplate retryTemplate = new RetryTemplate();
retryTemplate.setRetryPolicy(new SimpleRetryPolicy(3));
retryTemplate.setBackOffPolicy(new FixedBackOffPolicy());
return retryTemplate;
}
}
In this example, we use the handle
method to process the incoming messages and attach a retry advice to handle any exceptions that may occur during processing. We also configure an error handler to direct failed messages to a dead-letter queue for further analysis and processing.
Why Handle Errors and Retries
By incorporating error handling and retry logic within the integration flow, we ensure that the system can gracefully recover from transient failures and avoid message loss. The Java DSL allows us to define these error handling and retry mechanisms in a clear and concise manner, improving the robustness of our integration solution.
The Closing Argument
The Spring Integration Java DSL offers a powerful and expressive way to define integration flows in a more concise, type-safe, and readable manner. By leveraging the Java DSL, developers can simplify the complexity of integration configurations, enhance code maintainability, and incorporate robust error handling and retry mechanisms seamlessly.
In conclusion, the Java DSL is a valuable tool for tackling the complexities of Spring Integration, enabling developers to build scalable, resilient, and maintainable integration solutions with ease.
Incorporating the Java DSL into your Spring Integration projects can result in more efficient and manageable code, ultimately leading to a more robust and resilient integration solution.
To further enhance your understanding of Spring Integration Java DSL, check out the official Spring Integration Documentation and explore the wide range of capabilities it offers.
Start simplifying your Spring Integration configurations today with the intuitive and powerful Spring Integration Java DSL!