Why Most Algorithms Fail to Be Truly Efficient
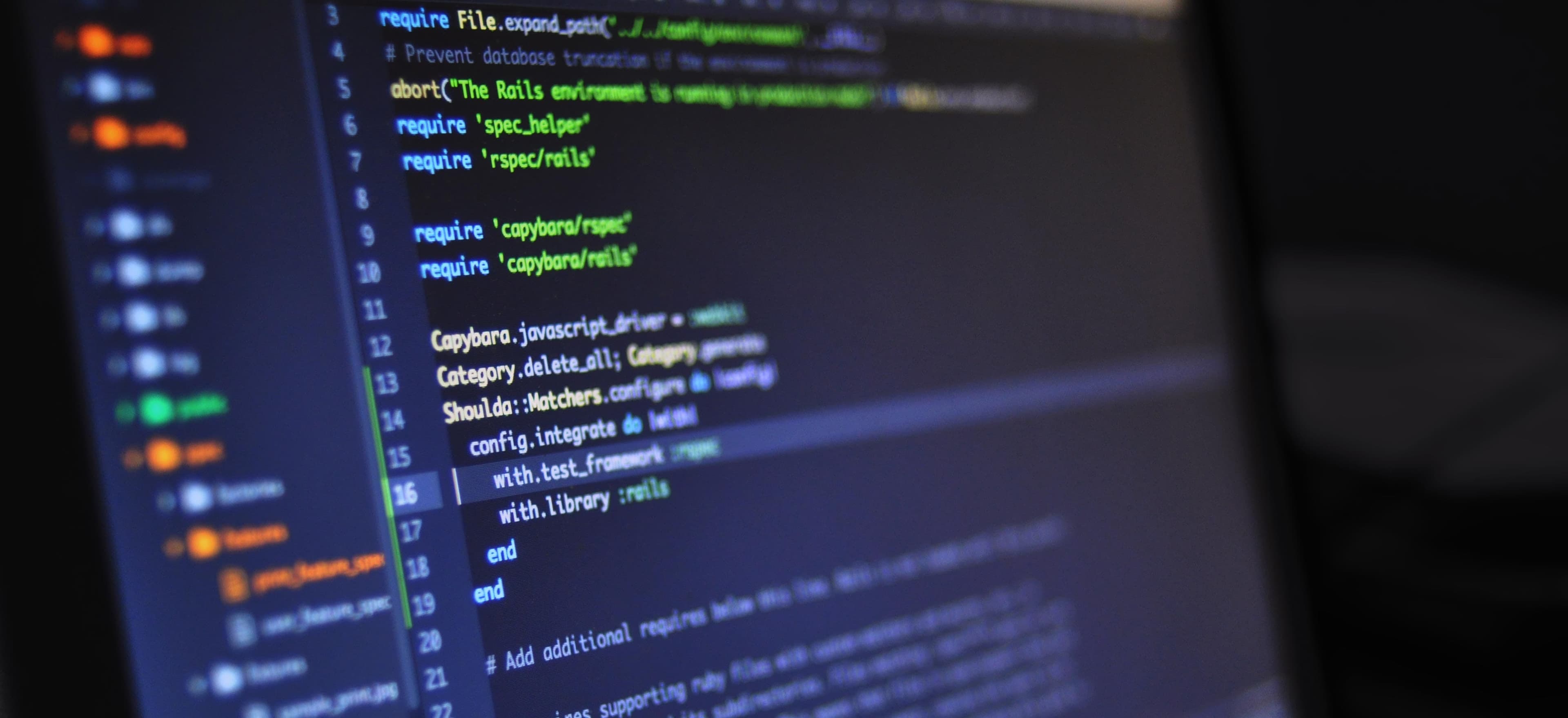
- Published on
Why Most Algorithms Fail to Be Truly Efficient
In the evolving world of computer science and programming, efficiency is often a critical concern. Algorithms, which are step-by-step procedures for calculations or data processing, are expected to run efficiently, utilizing minimal resources while providing accurate results. However, many algorithms fall short of these expectations. In this blog post, we will delve into the reasons why most algorithms fail to be truly efficient, common pitfalls, and best practices that can be followed to create more efficient algorithms.
Understanding Algorithm Efficiency
Algorithm efficiency is generally assessed by two primary factors: time complexity and space complexity.
- Time complexity measures how the runtime of an algorithm increases with the size of the input. It is usually expressed using Big O notation (for example, O(n), O(log n)).
- Space complexity evaluates the amount of memory an algorithm uses in relation to the input size.
An efficient algorithm should aim for both low time and space complexity. However, achieving this can be challenging due to various intrinsic limitations of algorithms and their implementations.
Common Reasons for Inefficiency
-
Suboptimal Algorithm Choice
One primary reason for inefficiency is the selection of algorithms that are not well-suited for the problem at hand. For instance, using a sorting algorithm with higher time complexity instead of a more efficient one can drastically increase runtime.
// Inserting the capability of bubble sort for small datasets. public void bubbleSort(int[] arr) { int n = arr.length; boolean swapped; for (int i = 0; i < n-1; i++) { swapped = false; for (int j = 0; j < n-i-1; j++) { if (arr[j] > arr[j+1]) { // Swap arr[j] and arr[j+1] int temp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = temp; swapped = true; } } if (!swapped) break; // No elements were swapped, the array is sorted. } }
In this example, Bubble Sort is simple but inefficient for large datasets. Instead, a more efficient sorting algorithm like Quick Sort or Merge Sort should be utilized.
-
Overly Complicated Logic
Another common issue is incorporating overly complicated logic that results in redundant computations. When algorithms perform unnecessary calculations, they generate excessive overhead. Code refactoring can help optimize performance.
Example of inefficient code:
public int factorial(int n) { if (n == 0) return 1; return n * factorial(n - 1); // Recursive call can lead to stack overflow for large n. }
A more efficient approach avoids deep recursion:
public int factorial(int n) { int result = 1; for (int i = 2; i <= n; i++) { result *= i; // Iterative approach to avoid stack overflow. } return result; }
-
Poor Data Structures
The choice of data structures can significantly influence the efficiency of an algorithm. Using data structures that provide inefficient operations (like linked lists for random access) can result in performance issues.
// Using an array for quick access int[] numbers = {1, 2, 3, 4, 5}; int idx = 2; System.out.println(numbers[idx]); // O(1) for retrieval // Using a LinkedList for quick access LinkedList<Integer> numberList = new LinkedList<>(); numberList.add(1); numberList.add(2); numberList.add(3); System.out.println(numberList.get(idx)); // O(n) for retrieval
Here, accessing an element in an array is constant time, O(1), whereas a LinkedList requires traversal, making it O(n).
-
Ignoring Edge Cases
An algorithm that does not account for edge cases can lead to performance issues or unexpected behavior. A robust algorithm should handle all scenarios efficiently.
public double divide(int a, int b) { if (b == 0) { throw new IllegalArgumentException("Division by zero is not allowed."); // Handle edge case } return (double) a / b; }
Ensure that your code gracefully manages unusual inputs to maintain efficiency under various conditions.
-
Neglecting Algorithmic Paradigms
There are various algorithmic paradigms, such as Greedy, Dynamic Programming, Divide and Conquer, etc. Depending on the problem, one may be more suitable than another. By neglecting to consider these paradigms, developers may end up with unoptimized solutions.
Consider calculating Fibonacci numbers using a Dynamic Programming approach versus a naive recursive solution.
// Naive recursive Fibonacci public int fibonacci(int n) { if (n <= 1) return n; return fibonacci(n - 1) + fibonacci(n - 2); // Exponential time complexity O(2^n) }
By using memoization or a DP approach, we can achieve linear time complexity.
public int fibonacci(int n) { int[] memo = new int[n + 1]; memo[0] = 0; memo[1] = 1; for (int i = 2; i <= n; i++) { memo[i] = memo[i - 1] + memo[i - 2]; // O(n) time complexity } return memo[n]; }
Best Practices for Efficient Algorithms
To circumvent the inefficiencies discussed above, consider the following best practices when developing algorithms:
-
Select the Right Algorithm: Familiarize yourself with various algorithms and data structures suitable for specific problem types, enhancing your chances of selecting the best one.
-
Keep It Simple: Avoid over-complicating your logic. Aim for scalability and sustainability in your design.
-
Profile Code: Utilize profiling tools to benchmark your algorithm and find performance bottlenecks. Optimizing based on actual data can lead to significant performance improvements.
-
Optimize Data Structures: When designing an algorithm, ensure that you're utilizing the most efficient data structure for your specific needs.
-
Handle Edge Cases: Always consider the corner cases where performance may degrade. Edge case analysis is crucial in defining robust algorithms.
-
Understand Algorithmic Paradigms: Knowing when to apply each of the algorithmic paradigms can be the key to solving a problem efficiently.
In Conclusion, Here is What Matters
While many algorithms may fail to deliver the efficiency that programmers seek, understanding the reasons behind this common phenomenon can enhance algorithmic design and implementation. By being mindful of algorithm choice, logical complexity, data structures, edge cases, and algorithmic paradigms, developers can craft efficient algorithms that meet the demands of their applications.
The journey toward efficient algorithms is continuous and evolving. By keeping our algorithms optimal and our code clean, we can minimize inefficiencies and pave the way for robust software solutions.
For further reading, consider diving deeper into the theory behind algorithms on GeeksforGeeks or exploring comprehensive resources on data structures on Java Tutorials.
Checkout our other articles