Mastering Spring: Avoid Common Pitfalls in Custom Annotations
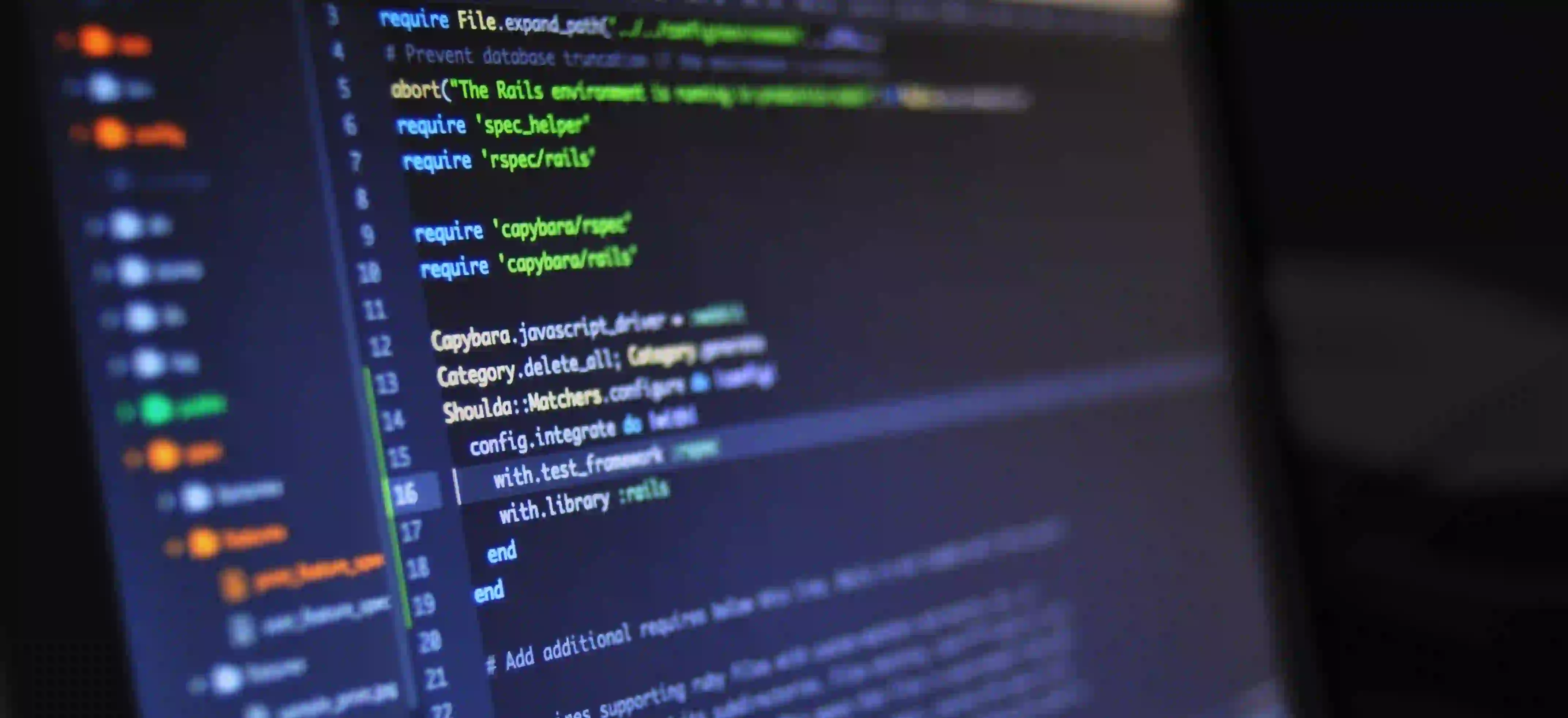
Mastering Spring: Avoid Common Pitfalls in Custom Annotations
Custom annotations in Java are a powerful tool for adding metadata to your code. When working with the Spring Framework, they can be used to simplify configuration, enhance readability, and promote reusability. However, creating custom annotations in Spring can lead to potential pitfalls if not implemented correctly. In this post, we will explore some common pitfalls when working with custom annotations in Spring and how to avoid them.
Pitfall #1: Missing meta-annotation for custom annotations
One common mistake when creating custom annotations in Spring is forgetting to add the meta-annotation @Retention
and @Target
to specify how and where the custom annotation can be used.
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface CustomAnnotation {
// Annotation attributes and logic here
}
Why: Adding @Retention(RetentionPolicy.RUNTIME)
ensures that the annotation is available at runtime, which is particularly important when using reflection in Spring. Meanwhile, @Target(ElementType.METHOD)
specifies that the annotation can only be used on methods.
Pitfall #2: Not defining annotation attributes
Another common pitfall is creating custom annotations without defining any attributes. Attributes are essential for custom annotations to carry additional information or configuration.
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface CustomAnnotation {
String value(); // Define at least one attribute
}
Why: Defining attributes allows for flexibility and customization when using the custom annotation. For example, the @RequestMapping
annotation in Spring defines attributes such as method
, value
, and produces
to configure request mappings.
Pitfall #3: Not using meta-annotations effectively
Meta-annotations are annotations that can be used as building blocks for creating new annotations. A common mistake is not leveraging meta-annotations effectively when creating custom annotations in Spring.
@GetMapping("/example")
public void handleExampleRequest() {
// Method logic here
}
Why: By using meta-annotations such as @RequestMapping
, @GetMapping
, or @PostMapping
, you can simplify the creation of custom annotations by combining existing annotations with consistent configurations.
Pitfall #4: Inconsistent usage of custom annotations
Inconsistency in using custom annotations can lead to confusion and maintenance challenges. It is essential to establish clear guidelines for when and how custom annotations should be used within a codebase.
@CustomAnnotation
public void methodA() {
// Method logic here
}
@CustomAnnotation
public void methodB() {
// Method logic here
}
Why: Establishing consistent usage patterns for custom annotations promotes code readability, maintainability, and reduces the risk of errors or misunderstandings.
Pitfall #5: Overcomplicating custom annotations
Custom annotations should aim to simplify and enhance code, not overcomplicate it. Avoid the temptation to create overly complex custom annotations with convoluted logic or excessive attributes.
@CustomAnnotation
public void methodWithOvercomplicatedLogic() {
// Method logic here
}
Why: Overcomplicated custom annotations can make code harder to understand and maintain. It's essential to strike a balance between using custom annotations to streamline code and keeping them simple and intuitive.
A Final Look
Custom annotations in Spring can be a valuable tool for improving code readability and maintainability. By avoiding common pitfalls such as missing meta-annotations, not defining attributes, not using meta-annotations effectively, inconsistent usage, and overcomplicating custom annotations, you can harness the full potential of custom annotations in your Spring projects.
Remember to leverage meta-annotations, define clear guidelines for usage, and keep custom annotations simple yet effective. By mastering the creation and usage of custom annotations in Spring, you can elevate the quality and maintainability of your codebase.
For more in-depth insights into custom annotations and Spring, you can explore the official Spring documentation and Baeldung's tutorials.