Mastering Microservices: Tackling Tough Code Patterns
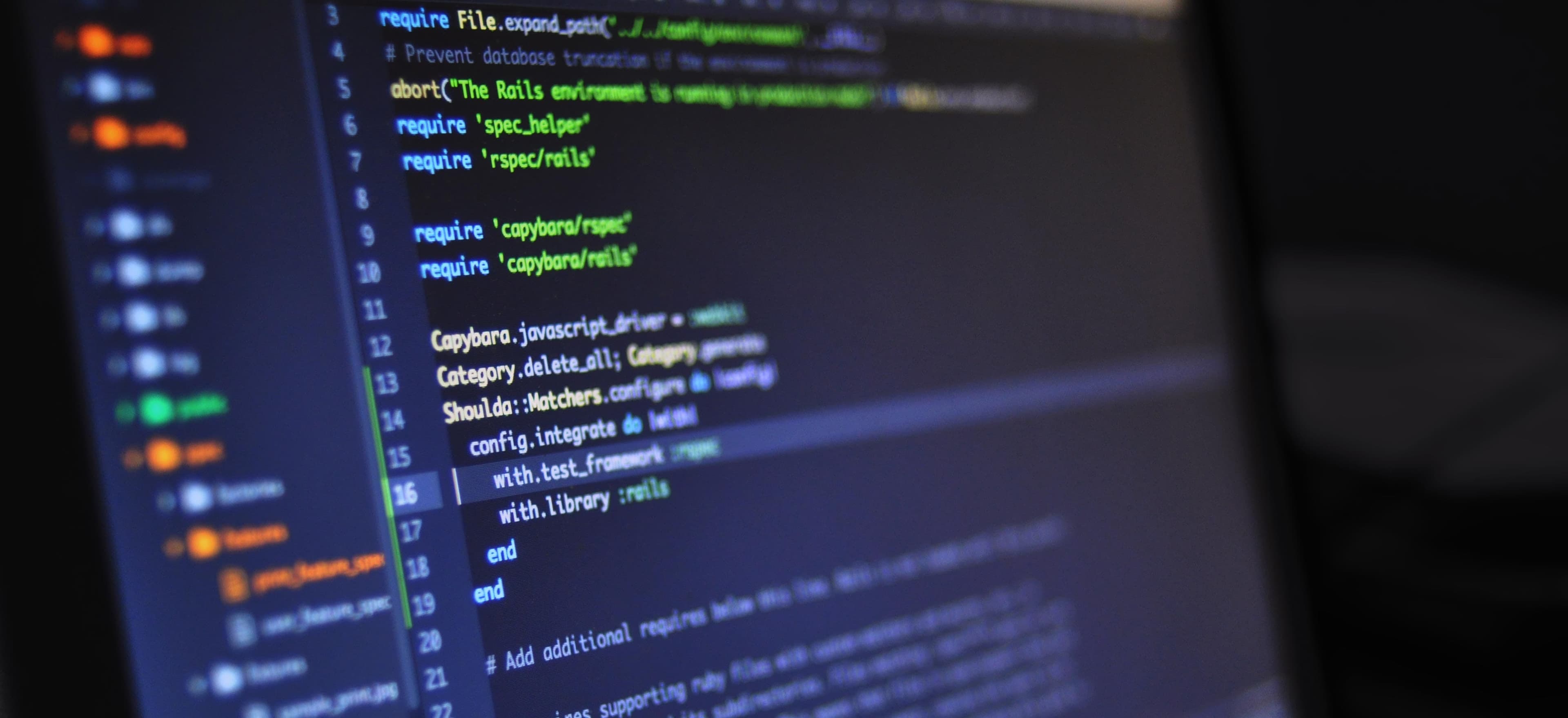
- Published on
Mastering Microservices: Tackling Tough Code Patterns
In the realm of modern software development, microservices architecture has gained significant traction due to its ability to build scalable and flexible applications. Microservices, with their independent deployability and scalability, offer a plethora of advantages, but with these advantages come the challenges of managing complex code patterns. This blog post aims to delve into some tough code patterns encountered in microservices development and explore strategies to effectively tackle them.
The Challenge of Distributed Systems
Microservices, by nature, are distributed systems. This distributed nature brings along a set of complexities, such as network latency, fault tolerance, and eventual consistency. Managing these complexities within the codebase is crucial for developing robust microservices.
Dealing with Network Latency
Handling network latency is paramount in a microservices architecture. When making inter-service calls, network latency can significantly impact the overall system performance. Utilizing asynchronous communication, such as message queues or reactive programming, can mitigate the impact of network latency by allowing services to continue processing other tasks while waiting for the response.
// Using CompletableFuture to perform asynchronous tasks in Java
CompletableFuture<Void> futureTask = CompletableFuture.runAsync(() -> {
// Asynchronous task logic
});
Utilizing libraries like Java's CompletableFuture can facilitate the implementation of asynchronous tasks, reducing the waiting time for network responses and improving system responsiveness.
Ensuring Fault Tolerance
Fault tolerance is another critical aspect of microservices. Services within a microservices architecture should be resilient to failures, cascading failures, and transient network issues. Implementing circuit breakers, where services can fail fast and fallback on alternative logic, helps in maintaining system stability.
// Implementing circuit breaker pattern using Hystrix
@HystrixCommand(fallbackMethod = "fallbackMethod")
public ResponseObject serviceMethod() {
// Service logic
}
Frameworks like Hystrix provide support for implementing the circuit-breaker pattern in Java, allowing services to handle failures gracefully.
Data Management Challenges
Microservices often deal with distributed data management, which introduces a new set of challenges. Ensuring data consistency, managing transactions, and orchestrating data across multiple services are some of the prominent data management challenges in a microservices architecture.
Ensuring Data Consistency
Maintaining data consistency in a distributed environment is a non-trivial task. Implementing the Saga pattern, where a series of local transactions are coordinated to maintain data consistency across services, can be an effective approach.
// Using the Saga pattern for managing distributed transactions
@Transactional
public void performComplexTransaction() {
// Transaction logic
}
Frameworks like Atomikos and Bitronix provide support for implementing distributed transactions using the Saga pattern in Java, aiding in maintaining data consistency across microservices.
Orchestration of Data Across Services
Orchestrating data across multiple services poses a considerable challenge. Implementing event-driven architecture, where services communicate through asynchronous events, can facilitate the orchestration of data across services without creating tight coupling.
// Using event sourcing for orchestrating data across services
public void handleEvent(EventObject event) {
// Event handling logic
}
Frameworks like Axon provide support for event sourcing and event-driven architecture in Java, enabling seamless orchestration of data across microservices.
Microservices Security Concerns
Security is a paramount aspect of microservices development. With a distributed architecture, ensuring data privacy, authentication, and authorization across services is of utmost importance.
Authenticating and Authorizing Requests
Implementing a robust authentication and authorization mechanism is crucial for securing microservices. Leveraging JWT (JSON Web Tokens) for authentication and role-based access control for authorization can bolster the security of microservices.
// Using JWT for authentication in Java
String token = Jwts.builder().setSubject("username").signWith(SignatureAlgorithm.HS256, "secretKey").compact();
Libraries like JJWT provide support for implementing JWT-based authentication and authorization in Java, enhancing the security posture of microservices.
Securing Communication Between Services
Securing communication channels between services is pivotal. Implementing mutual TLS (Transport Layer Security) or leveraging service mesh solutions like Istio can ensure secure communication and data privacy between microservices.
// Implementing mutual TLS for securing communication in Java
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, trustManagerFactory.getTrustManagers(), null);
Frameworks like Java's javax.net.ssl
package facilitate the implementation of mutual TLS for securing communication between microservices.
Testing and Monitoring Challenges
Testing and monitoring microservices present unique challenges. With the distributed nature of microservices, ensuring comprehensive testing and effective monitoring becomes imperative.
Implementing Contract Testing
Contract testing ensures that interactions between services are consistent and reliable. Tools like Pact enable contract testing by defining and verifying service contracts, ensuring compatibility and reliability in a distributed environment.
// Using Pact for contract testing in Java
public void verifyServiceContracts() {
MockProviderConfig config = new MockProviderConfig.http(8080);
MockProviderRule mockProviderRule = new MockProviderRule(config, this);
}
Pact-JVM provides support for contract testing in Java, allowing developers to validate service contracts and interactions effectively.
Microservices Monitoring and Tracing
Effective monitoring and tracing of microservices are essential for identifying performance bottlenecks and debugging issues. Implementing distributed tracing using tools like Zipkin or Jaeger helps in visualizing and understanding the flow of requests across microservices.
// Instrumenting microservices for distributed tracing using Zipkin
Tracing tracing = Tracing.newBuilder().localServiceName("my-service").sampler(Sampler.ALWAYS_SAMPLE).build();
Libraries like Brave facilitate the instrumentation of microservices for distributed tracing in Java, enabling comprehensive monitoring and tracing capabilities.
A Final Look
Mastering tough code patterns in microservices is crucial for building robust and resilient systems. By addressing challenges related to distributed systems, data management, security, testing, and monitoring, developers can effectively navigate the complexities of microservices development. Leveraging appropriate patterns, tools, and frameworks in Java empowers developers to conquer the intricacies of microservices and build scalable, reliable, and secure applications.
Embracing the intricacies of microservices development equips developers with the skills to architect resilient systems, paving the way for innovation and scalability in modern software development.
Remember, mastering microservices entails not only understanding the intricacies of code patterns but also embracing the paradigm shift towards distributed, resilient, and scalable architectures.
Happy coding!
Disclaimer: The code snippets provided are exemplary and may require adaptation to specific use cases and best practices within individual projects.
References:
- CompletableFuture in Java: https://www.baeldung.com/java-completablefuture
- Hystrix Circuit Breaker: https://github.com/Netflix/Hystrix/wiki
- Atomikos Transactions: http://www.atomikos.com/
- Axon Framework: https://axoniq.io/
- JWT in Java: https://github.com/jwtk/jjwt
- Istio Service Mesh: https://istio.io/
- Pact-JVM: https://github.com/DiUS/pact-jvm
- Zipkin Distributed Tracing: https://zipkin.io/
- Brave Distributed Tracing: https://github.com/openzipkin/brave
Checkout our other articles