Overcoming Outsider Blockers: Team Dependency Solutions
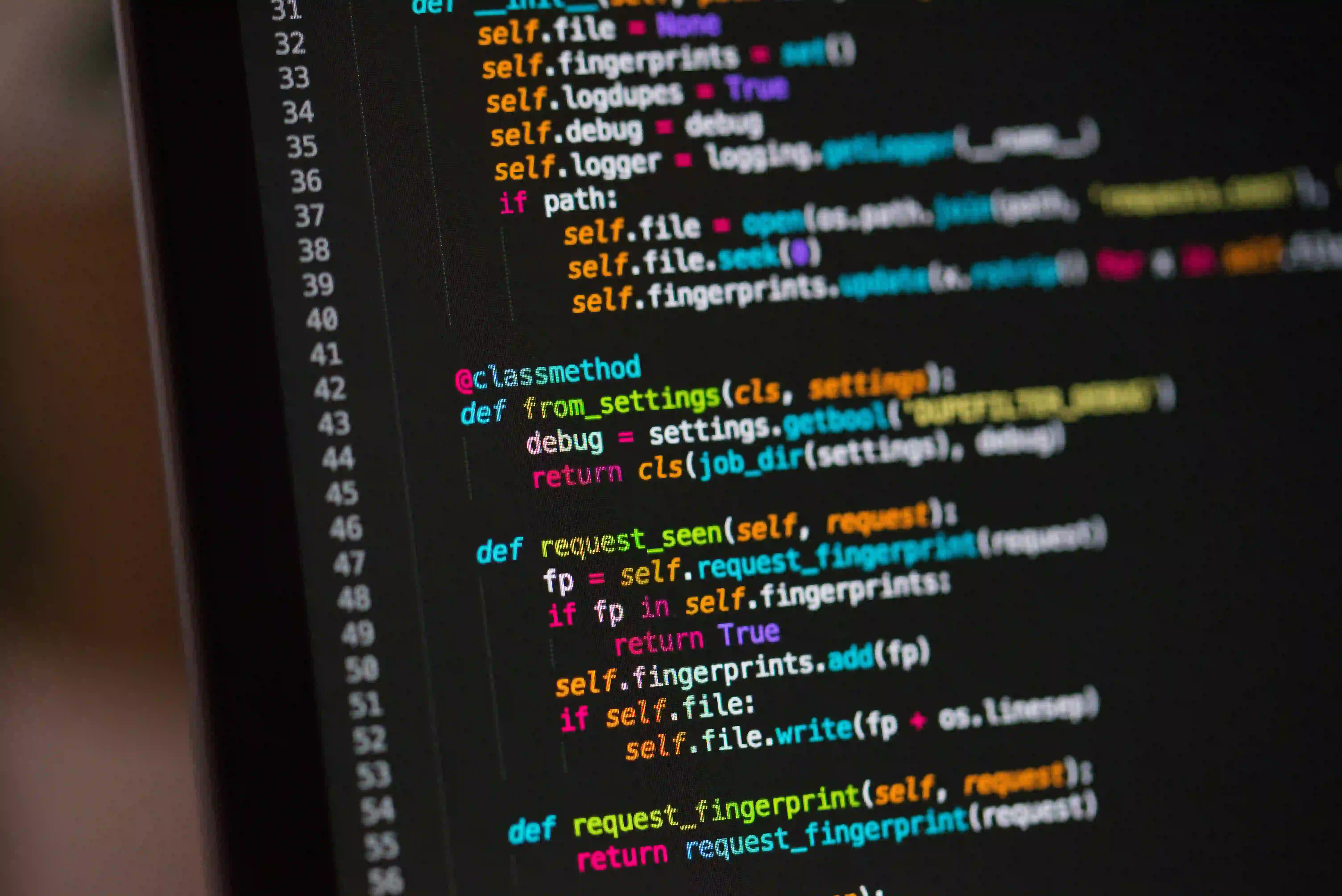
Overcoming Outsider Blockers: Team Dependency Solutions
In Java development, managing dependencies is a critical aspect of building robust and efficient applications. As projects become more complex, managing dependencies and dealing with outsider blockers become a common challenge. This blog post aims to provide insights into overcoming outsider blockers and managing team dependencies in Java development by implementing effective solutions.
Understanding Outsider Blockers
Outsider blockers occur when a team member's progress is hindered due to external factors, such as dependencies on other team members' work, third-party APIs, or external services. These blockers can significantly slow down the development process and hinder overall productivity.
Effective Dependency Management
Effective dependency management is crucial for addressing outsider blockers and maintaining project momentum. Tools like Maven and Gradle provide powerful dependency management capabilities in Java projects. By defining project dependencies in a central configuration file, such as pom.xml
for Maven or build.gradle
for Gradle, teams can ensure consistent and reliable dependency resolution.
Maven Example
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>example-library</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
In this example, the pom.xml
file specifies a dependency on an external library with a specific version. This declarative approach to dependency management enables seamless sharing and integration of dependencies across team members and projects.
Embracing Modularization
Modularization is a key strategy for reducing the impact of outsider blockers. By breaking down a large monolithic codebase into smaller, decoupled modules, teams can work on independent components without being overly reliant on each other's work.
Java 9 Modules
With the introduction of modules in Java 9, developers can define clear boundaries and interdependencies between various parts of their applications. This promotes encapsulation and allows teams to work on specific modules without being obstructed by dependencies on unrelated code.
module com.example.application {
requires com.example.module1;
requires com.example.module2;
...
}
By explicitly declaring module dependencies, teams can isolate their work and reduce the impact of outsider blockers on individual modules.
Continuous Integration and Delivery
Implementing a robust continuous integration and delivery (CI/CD) pipeline can help mitigate the impact of outsider blockers. By automating build, test, and deployment processes, teams can identify and address dependency-related issues early in the development lifecycle.
Jenkins Pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
stage('Deploy') {
steps {
sh 'mvn deploy'
}
}
}
}
By incorporating dependency resolution, compilation, testing, and deployment steps into a CI/CD pipeline, teams can minimize the impact of outsider blockers and ensure consistent progress across the development cycle.
Dealing with External Services and APIs
External services and APIs often introduce a level of uncertainty and potential for outsider blockers. It's crucial to implement robust error handling, fallback mechanisms, and service mocks to prevent external dependencies from halting development progress.
Circuit Breaker Pattern
The circuit breaker pattern, popularized by libraries like Netflix Hystrix, provides a mechanism for dealing with external service failures. By wrapping calls to external dependencies with a circuit breaker, teams can gracefully handle failures and prevent cascading issues caused by unreliable services.
CircuitBreaker circuitBreaker = new CircuitBreaker()
.onOpen(() -> log.error("Circuit open - fallback enabled"))
.onClose(() -> log.info("Circuit closed - normal operation"))
.onHalfOpen(() -> log.warn("Circuit half-open - testing the waters"))
.fallback(() -> performFallbackOperation());
By incorporating resilient patterns like the circuit breaker, teams can minimize the impact of outsider blockers caused by external dependencies.
To Wrap Things Up
Managing team dependencies and overcoming outsider blockers in Java development requires a combination of effective tools, modularization, continuous integration, and resilient patterns. By embracing these solutions, teams can minimize the impact of external factors and maintain consistent progress towards delivering high-quality software.
Additional Resources
For further insights into Java dependency management and modularization, refer to the following resources:
By implementing effective solutions to manage team dependencies and address outsider blockers, Java development teams can ensure a smoother progression towards project completion. With a strong foundation in dependency management and modularization, the impact of external factors can be significantly mitigated, allowing for a more efficient and productive development process.