Refactor Smarter: Moving Static Methods to a New Class!
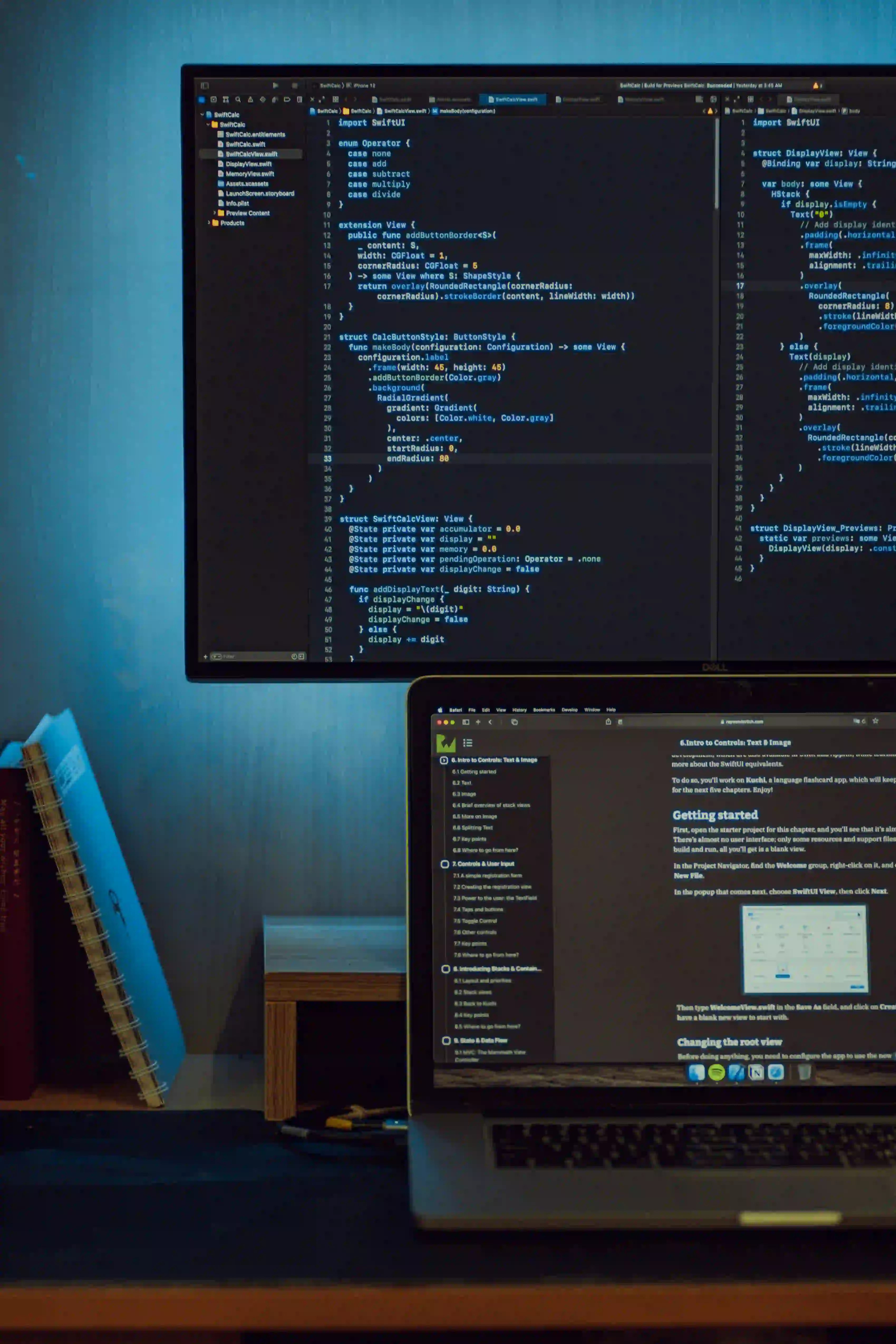
Refactor Smarter: Moving Static Methods to a New Class!
Refactoring is an essential part of software development. It allows you to improve the design, structure, and readability of your code without changing its functionality. In this blog post, we will delve into the practice of moving static methods to a new class for a smarter and more organized codebase.
What are Static Methods?
Static methods in Java belong to the class and not to the instances of the class. They can be invoked without the need for creating an instance of the class. These methods are commonly used for utility functions or operations that do not require object state.
Here's an example of a static method:
public class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
The add
method in the MathUtils
class is a static method that adds two integers without the need to instantiate an object of the MathUtils
class.
When to Consider Moving Static Methods to a New Class
As a codebase grows, classes tend to accumulate a multitude of static methods, leading to clutter and decreased maintainability. When you notice a group of related static methods within a class, it's a telltale sign that they could be extracted to a new class.
By moving these static methods to a new class, you can improve code organization, promote reusability, and enhance testability. This not only adheres to the core principles of object-oriented programming but also sets the stage for more modular and cohesive code.
The Steps to Refactor
Let's walk through the steps to refactor and move static methods to a new class in Java.
Step 1: Identify Related Static Methods
Identify a cohesive set of static methods within a class that can be grouped together based on their functionality. For example, if you have static methods related to file operations, they can be candidates for extraction.
Step 2: Create a New Class
Create a new class that captures the essence of the functionality represented by the identified static methods.
public class FileUtils {
public static void copyFile(String source, String destination) {
// Implementation
}
public static void deleteFile(String file) {
// Implementation
}
}
Step 3: Modify Client Code
Update the client code to invoke the static methods from the new class.
Before:
FileUtils.copyFile("source.txt", "destination.txt");
After:
FileUtils.copyFile("source.txt", "destination.txt");
Step 4: Test and Refactor
Ensure that the refactored code works as expected by running tests and validating the functionality. Additionally, utilize this opportunity to further refactor the code for improved readability and maintainability.
The Benefits of Moving Static Methods to a New Class
Improved Code Organization
By segregating related static methods into their own class, you create a clear and focused environment, making it easier for developers to locate and comprehend the code.
Enhanced Reusability
With static methods encapsulated within a dedicated class, they become more easily reusable across different parts of the codebase, promoting the Don't Repeat Yourself (DRY) principle.
Simplified Testing
Isolating static methods into their own class facilitates easier testing as they can be individually tested without needing to involve the complexities of the parent class.
Closing Remarks
Refactoring static methods to a new class is a pragmatic approach towards maintaining a clean, cohesive, and maintainable codebase. By following the outlined steps and leveraging the benefits, you can streamline your code and pave the way for a more efficient and scalable software architecture.
So, the next time you encounter a cluster of static methods within a class, consider refactoring them to a new class for a smarter and more organized codebase!
Continue to refine your Java skills and learn more about best practices by checking out Oracle's official Java documentation and Baeldung's Java tutorials.
Happy coding!