Overcome Common Pitfalls: Updating DynamoDB Items in Java
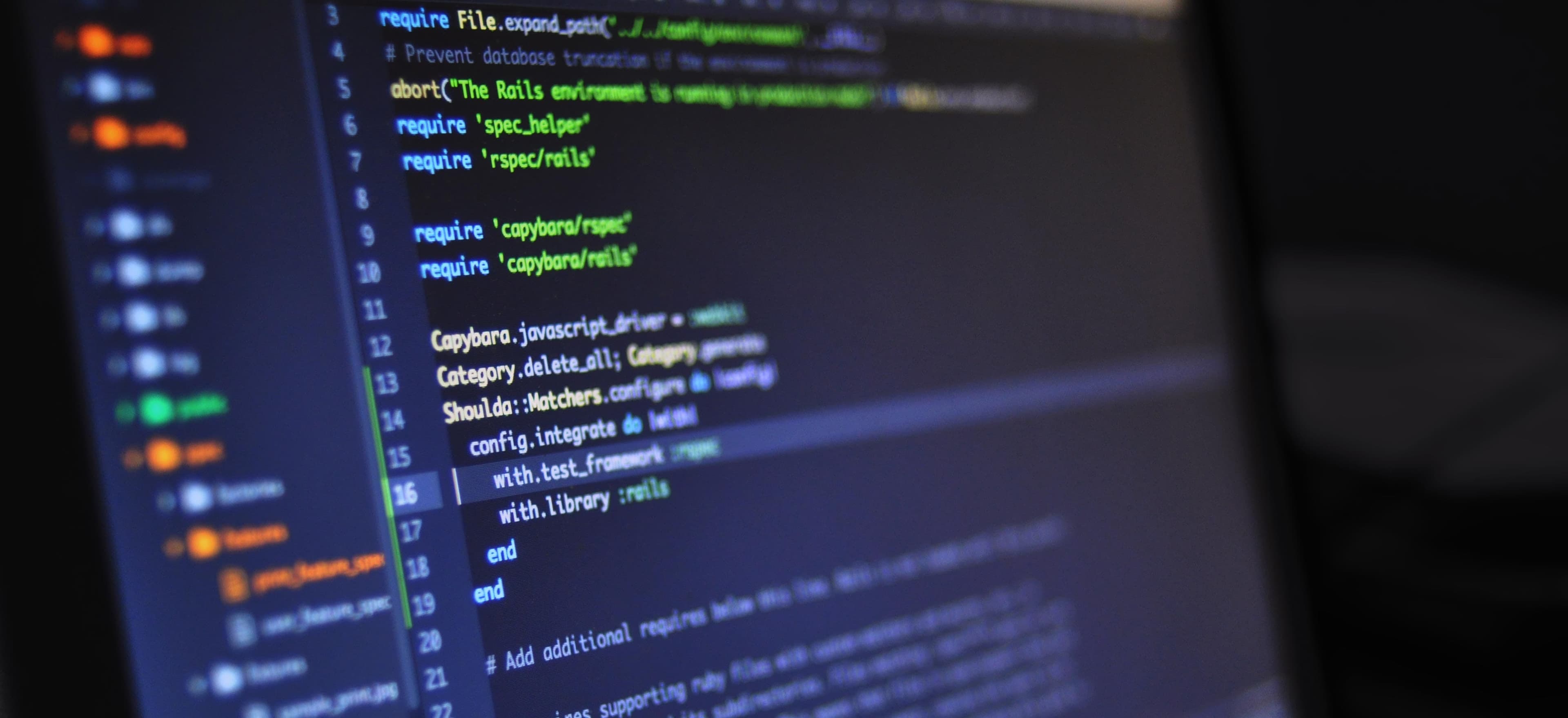
- Published on
Master the Art of Updating DynamoDB Items in Java
DynamoDB, a fully managed NoSQL database service provided by AWS, offers fast and flexible document data storage. However, updating items in DynamoDB, especially in the context of a Java application, comes with its own set of challenges and potential pitfalls. In this post, we'll delve into best practices and effective strategies for updating DynamoDB items in Java, ensuring smooth and efficient data manipulation while avoiding common stumbling blocks.
1. Understand the DynamoDB Data Model
Before delving into the code, it's crucial to have a solid understanding of the DynamoDB data model. DynamoDB employs key concepts such as tables, items, and attributes which differ from traditional SQL databases. For a comprehensive understanding, refer to the official Amazon DynamoDB developer guide.
2. Use the AWS SDK for Java
The AWS SDK for Java simplifies interaction with DynamoDB, providing a high-level API for seamless integration. Ensure that you have the AWS SDK for Java included in your project, either through Maven or Gradle.
For Maven, include the following dependency:
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>dynamodb</artifactId>
</dependency>
For Gradle, add the dependency:
implementation 'software.amazon.awssdk:dynamodb'
3. The UpdateItem API Call
The UpdateItem
API call is a powerful tool for modifying existing items in DynamoDB. This operation allows you to update specific attributes or add new ones while preserving the rest of the item.
Here's a basic example of using the UpdateItem
API call:
UpdateItemRequest updateItemRequest = UpdateItemRequest.builder()
.tableName("YourTableName")
.key(Map.of("primaryKey", AttributeValue.builder().s("yourPrimaryKeyValue").build()))
.updateExpression("SET #attrName = :attrValue")
.expressionAttributeNames(Map.of("#attrName", "yourAttributeName"))
.expressionAttributeValues(Map.of(":attrValue", AttributeValue.builder().s("newValue").build()))
.build();
dynamoDbClient.updateItem(updateItemRequest);
In this example, we are updating a specific attribute (yourAttributeName
) of an item in the DynamoDB table.
4. Utilize Conditional Expressions for Atomic Updates
In scenarios where you need to ensure that an update occurs only if certain conditions are met, DynamoDB's conditional expressions come into play. By using conditional expressions, you can achieve atomic updates, ensuring the integrity of your data.
Here's an example of utilizing conditional expressions in an update operation:
UpdateItemRequest updateItemRequest = UpdateItemRequest.builder()
.tableName("YourTableName")
.key(Map.of("primaryKey", AttributeValue.builder().s("yourPrimaryKeyValue").build()))
.updateExpression("SET #attrName = :attrValue")
.conditionExpression("attribute_exists(#attrName)")
.expressionAttributeNames(Map.of("#attrName", "yourAttributeName"))
.expressionAttributeValues(Map.of(":attrValue", AttributeValue.builder().s("newValue").build()))
.build();
dynamoDbClient.updateItem(updateItemRequest);
In this example, the update will only occur if the attribute yourAttributeName
exists in the item. If the condition isn't met, the update operation will be rejected.
5. Handle Concurrent Updates with Conditional Expressions
To handle concurrent updates effectively, DynamoDB provides a feature called optimistic locking. This prevents simultaneous updates from overwriting each other's changes. Conditional expressions play a pivotal role in implementing optimistic locking.
UpdateItemRequest updateItemRequest = UpdateItemRequest.builder()
.tableName("YourTableName")
.key(Map.of("primaryKey", AttributeValue.builder().s("yourPrimaryKeyValue").build()))
.updateExpression("SET #attrName = :attrValue")
.conditionExpression("#attrName = :currentValue")
.expressionAttributeNames(Map.of("#attrName", "yourAttributeName"))
.expressionAttributeValues(Map.of(
":attrValue", AttributeValue.builder().s("newValue").build(),
":currentValue", AttributeValue.builder().s("expectedValue").build()))
.build();
dynamoDbClient.updateItem(updateItemRequest);
In this scenario, the update will only proceed if the current value of yourAttributeName
matches the expected value (expectedValue
). If the values differ, the update request will be rejected, indicating that the item has been modified elsewhere.
6. Leverage Version Numbering for Optimistic Locking
An alternative approach to implementing optimistic locking involves the use of version numbers. By incorporating a version attribute in your DynamoDB items, you can compare and update this attribute to manage concurrent modifications.
UpdateItemRequest updateItemRequest = UpdateItemRequest.builder()
.tableName("YourTableName")
.key(Map.of("primaryKey", AttributeValue.builder().s("yourPrimaryKeyValue").build()))
.updateExpression("SET #attrName = :attrValue, #versionAttr = :newVersion")
.conditionExpression("#versionAttr = :currentVersion")
.expressionAttributeNames(Map.of("#attrName", "yourAttributeName", "#versionAttr", "version"))
.expressionAttributeValues(Map.of(
":attrValue", AttributeValue.builder().s("newValue").build(),
":newVersion", AttributeValue.builder().n("2").build(),
":currentVersion", AttributeValue.builder().n("1").build()))
.build();
dynamoDbClient.updateItem(updateItemRequest);
In this example, the version
attribute serves as a concurrency control mechanism. The update will only occur if the current version matches the expected version, preventing conflicting modifications.
7. Error Handling and Retries
When updating items in DynamoDB, it's essential to handle potential errors gracefully. Network issues, provisioned throughput exceeded errors, or conditional check failures are some of the scenarios that warrant robust error handling.
try {
// UpdateItemRequest setup
dynamoDbClient.updateItem(updateItemRequest);
} catch (ConditionalCheckFailedException e) {
// Handle conditional check failure
// Optionally, retry with updated data
} catch (DynamoDbException e) {
// Handle other DynamoDB related exceptions
// Perform retries if applicable
}
By catching specific exceptions such as ConditionalCheckFailedException
, you can tailor your error handling based on the type of error encountered, improving the robustness of your item update logic.
8. Embrace ORM Libraries for Enhanced Productivity
For complex applications with extensive DynamoDB interactions, utilizing Object-Relational Mapping (ORM) libraries like DynaORM or DynamoDBMapper can streamline your development process. ORM libraries abstract away the low-level DynamoDB operations, providing a more intuitive and object-oriented interface.
By leveraging a capable ORM library, you can focus on the business logic of your application, delegating the intricacies of database interaction to the library's functionality.
In Summary
Updating items in DynamoDB within a Java application demands a meticulous approach, considering factors such as data integrity, concurrency, and error resilience. By understanding DynamoDB's data model, harnessing the capabilities of the AWS SDK for Java, and employing strategies like conditional expressions and optimistic locking, you can navigate through the complexities of item updates with finesse.
Mastering the techniques outlined in this post will empower you to build robust, performant, and reliable applications that harness the power of DynamoDB while gracefully handling data manipulation.
Stay tuned for more insightful content on advanced DynamoDB interactions and Java best practices!