Simplify Java: Reduce Boilerplate with Project Lombok!
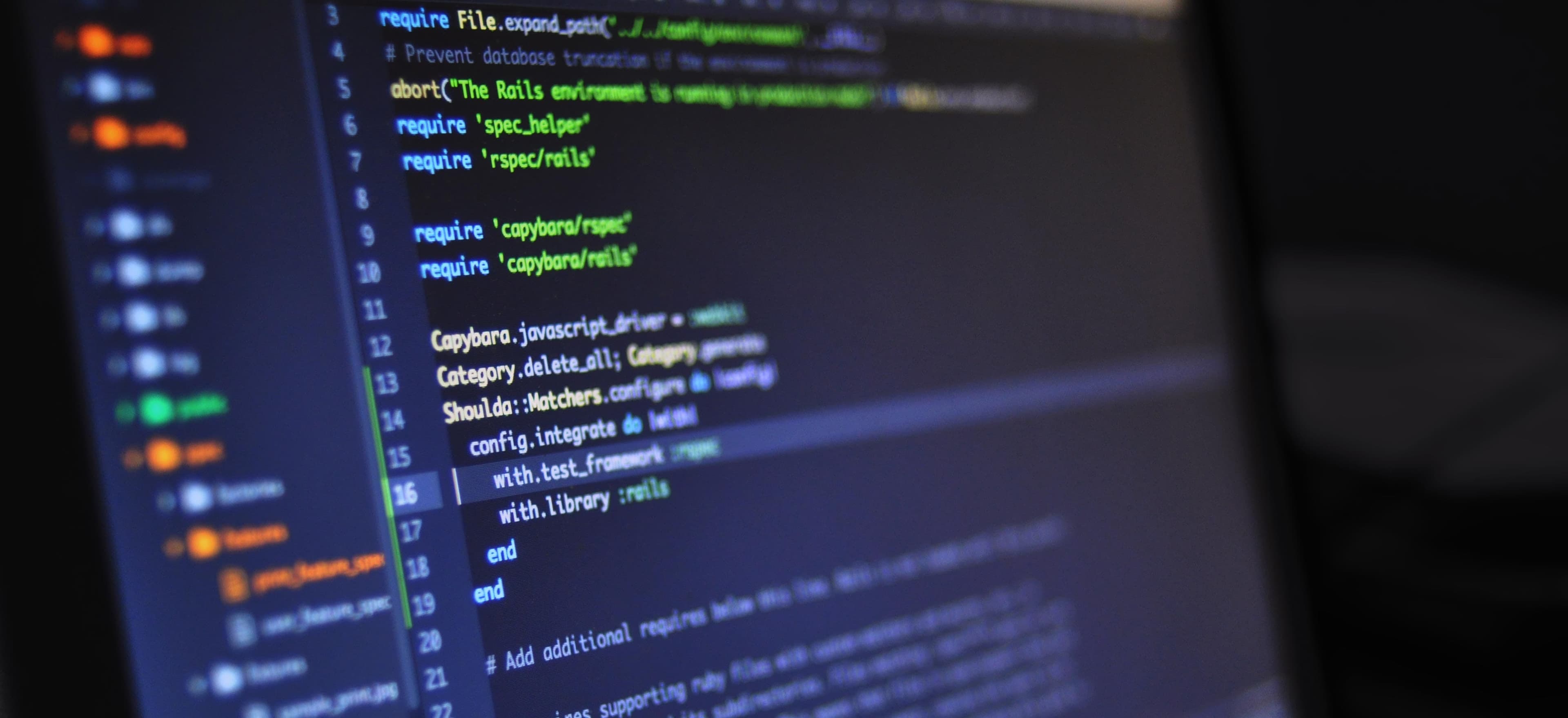
- Published on
Simplify Java: Reduce Boilerplate with Project Lombok
Are you tired of writing the same repetitive Java code over and over again? Do you wish there was a way to reduce boilerplate code and focus more on the essential aspects of your application? Look no further! In this post, we will explore how Project Lombok can help streamline your Java development by effectively eliminating repetitive code patterns, allowing you to write cleaner, more concise code without sacrificing readability or maintainability.
What is Project Lombok?
Project Lombok is a popular library that aims to reduce Java verbosity by automatically generating common code, such as getters, setters, constructors, and more. It achieves this by leveraging a set of annotations that trigger the generation of these mundane tasks at compile time, effectively eliminating the need for developers to write them manually.
Getting Started with Project Lombok
To integrate Project Lombok into your Java project, you need to add the Lombok dependency to your build tool. If you are using Maven, you can do this by adding the following dependency to your pom.xml
file:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
<scope>provided</scope>
</dependency>
Once you have added the dependency, you need to enable annotation processing in your IDE. For example, in IntelliJ IDEA, you can enable annotation processing by going to Settings > Build, Execution, Deployment > Compiler > Annotation Processors
and checking the box for "Enable annotation processing."
Reducing Boilerplate with Lombok Annotations
Let's dive into some of the most commonly used Lombok annotations and see how they can effectively reduce boilerplate code in your Java classes.
@Data
The @Data
annotation combines the features of @ToString
, @EqualsAndHashCode
, @Getter
, and @Setter
, effectively generating all the boilerplate code typically associated with these methods. By annotating a class with @Data
, Lombok takes care of generating the toString
, equals
, hashCode
, and the respective getter and setter methods for all fields in the class.
@Data
public class User {
private Long id;
private String username;
private String email;
}
With just the @Data
annotation, we have effectively eliminated the need to write boilerplate methods suchjsonmamaea;a Getters,Setters, toString,equals and hashCode.of what you consider important, such as visibility and even thread-safety.
@NonNull
The @NonNull
annotation is used to automatically generate null-checks for constructor parameters or methods arguments, effectively eliminating the need for explicit null-checks within the method body. If a null value is passed to a method or constructor parameter annotated with @NonNull
, Lombok will automatically generate a null-check for that parameter.
public class UserService {
public void createUser(@NonNull String username, @NonNull String email) {
// Automatically generates null-checks for username and email
// Business logic to create a user
}
}
By annotating the method parameters with @NonNull
, Lombok ensures that null values are checked at the entry point of the method, promoting robustness and reliability in your code.
@Builder
The @Builder
annotation generates a builder pattern for your class, allowing for easier and more readable instantiation of objects with many fields. It eliminates the need to write complex and error-prone telescoping constructors or numerous overloaded constructors to accommodate different instantiation scenarios.
@Builder
public class User {
private Long id;
private String username;
private String email;
}
// Instantiation using the builder pattern
User user = User.builder()
.id(1L)
.username("john_doe")
.email("john@example.com")
.build();
By annotating the class with @Builder
, Lombok gives you a fluent and expressive way to construct objects, enhancing the readability of your code while minimizing potential errors associated with large numbers of constructor parameters.
@Cleanup
The @Cleanup
annotation automatically manages resources that require cleanup, such as closing streams or releasing locks. It ensures that the resource is properly closed after the block of code, effectively eliminating the need for explicit try-with-resources
blocks and manual resource cleanup.
public void processFile(String filePath) {
@Cleanup FileInputStream fileInputStream = new FileInputStream(filePath);
// Read and process the file
}
With @Cleanup
, Lombok takes care of closing the FileInputStream
after the block of code, promoting cleaner and more readable resource management in your Java applications.
Final Considerations
By leveraging the power of Project Lombok, you can significantly reduce the boilerplate code in your Java applications, leading to cleaner, more readable code while maintaining the robustness and reliability of your software. Whether it's automatic generation of common methods, null-checks, builder patterns, or resource cleanup, Lombok empowers you to focus on the core logic of your application without getting bogged down by repetitive, mundane tasks.
So why wait? Streamline your Java development with Project Lombok and experience the joy of writing elegant, concise code without the burden of unnecessary verbosity. Embrace the power of automation and let Lombok supercharge your productivity!
Are you ready to simplify your Java development with Project Lombok? Share your thoughts and experiences with us!
Checkout our other articles