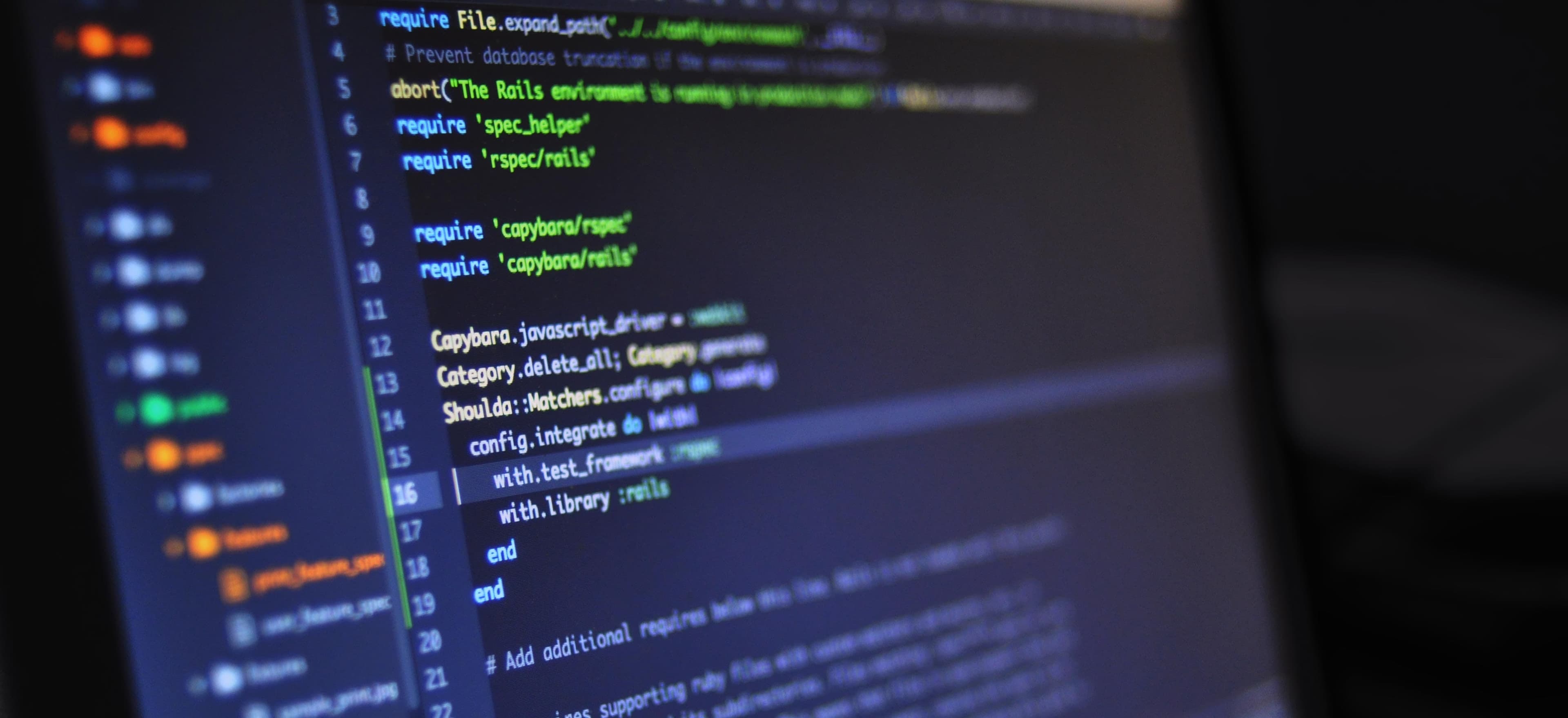
- Published on
Revolutionize Your Tests: Spring & Selenium with Annotations!
Testing is an integral part of the software development process. When it comes to testing web applications, Selenium has been the go-to framework for many developers. However, integrating Selenium tests with the Spring framework can be a game-changer. In this blog post, we will explore how to revolutionize your tests by combining the power of Spring and Selenium with the help of annotations.
Why Use Spring and Selenium Together?
Before we dive into the technical details, let's understand why combining Spring and Selenium can be advantageous.
1. Dependency Injection:
- Spring provides a robust framework for dependency injection, allowing easy management of objects and their dependencies. By integrating Selenium with Spring, we can leverage the benefits of dependency injection for our web testing components.
2. Simplified Configuration:
- Spring's configuration management capabilities allow for a cleaner, more organized setup of Selenium tests. This leads to better maintainability and flexibility when managing test configurations.
3. Test Reusability:
- With Spring's support for bean definition and lifecycle management, Selenium test components can be designed for maximum reusability, promoting the "write once, use everywhere" principle.
Getting Started: Setting Up the Project
To begin, let's set up a new Maven project and add the necessary dependencies for Spring and Selenium.
<dependencies>
<!-- Spring Dependencies -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>{spring-version}</version>
</dependency>
<!-- Selenium Dependencies -->
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>{selenium-version}</version>
</dependency>
</dependencies>
Replace {spring-version}
and {selenium-version}
with the respective versions you intend to use.
Writing Selenium Tests with Spring Annotations
Now, let's see how we can utilize Spring annotations to streamline our Selenium tests.
1. Spring Configuration
Create a Spring configuration file and define the beans for the WebDriver and other test-related components.
@Configuration
public class TestConfig {
@Bean
public WebDriver webDriver() {
// Initialize and return the WebDriver instance (e.g., ChromeDriver)
}
@Bean
public LoginPage loginPage() {
return new LoginPage(webDriver());
}
// Other beans and configurations
}
Here, we have defined a TestConfig
class annotated with @Configuration
. The webDriver
bean provides an instance of the WebDriver while the loginPage
bean represents the login page of the web application.
2. Test Class with Annotations
Now, let's create a test class and utilize Spring annotations for dependency injection.
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = TestConfig.class)
public class LoginTest {
@Autowired
private WebDriver webDriver;
@Autowired
private LoginPage loginPage;
@Test
public void testLogin() {
// Test logic using WebDriver and loginPage
}
}
In the LoginTest
class, we have used the @RunWith
and @ContextConfiguration
annotations to integrate with Spring. The @Autowired
annotation is used for dependency injection of the WebDriver
and LoginPage
beans into the test class.
By leveraging Spring annotations, we have effectively integrated Selenium WebDriver and test components with the Spring framework, simplifying the test setup and promoting maintainability.
To Wrap Things Up
In this blog post, we have explored the benefits of combining Spring and Selenium for test automation, and demonstrated how to leverage Spring annotations for seamless integration. By embracing this approach, developers can streamline their test setup, enhance reusability, and improve the maintainability of their Selenium tests.
If you're interested in diving deeper into Selenium and Spring integration, take a look at Spring's official documentation and the Selenium official website for further insights.
Revolutionize your testing approach today by harnessing the power of Spring and Selenium with annotations!
Checkout our other articles