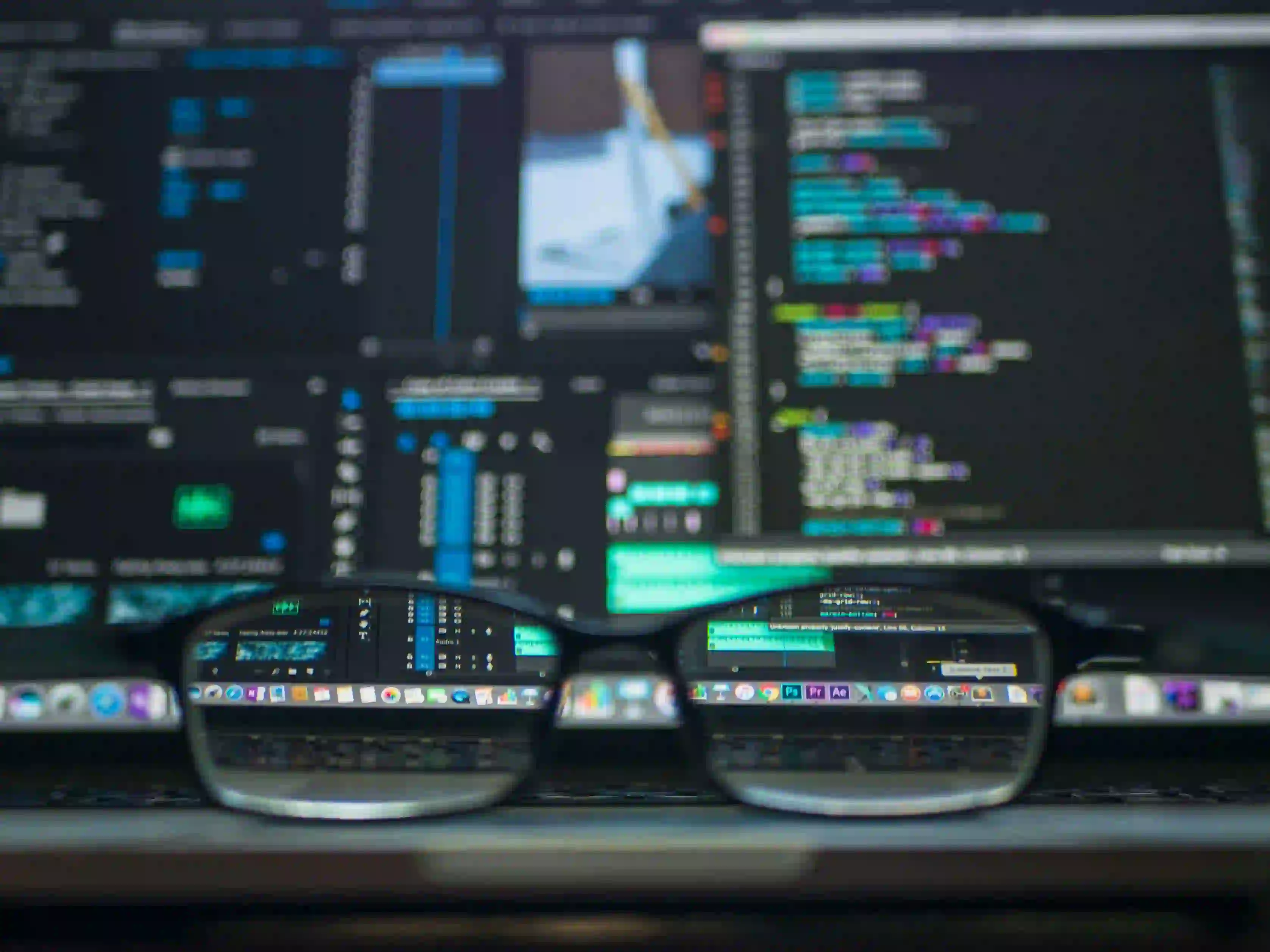
Mastering Akka HTTP Deployment: Maven & Docker for Java Devs
Are you a Java developer looking to deploy your Akka HTTP application with ease and efficiency? In this guide, we will explore the seamless integration of Maven, Docker, and Akka HTTP for streamlined deployment. By implementing best practices and leveraging the power of these tools, you will be able to achieve a robust and scalable deployment process for your Akka HTTP applications.
Understanding Akka HTTP
Akka HTTP is a powerful toolkit for building HTTP-based services and APIs using the Actor model and reactive programming principles. It provides a high-level server-side and client-side API for building RESTful and WebSocket-based services. With its asynchronous, non-blocking, and highly performant nature, Akka HTTP is well-suited for developing modern, scalable web applications.
Leveraging Maven for Dependency Management
Maven is a widely-used build automation and dependency management tool for Java projects. It simplifies the process of managing project dependencies, building and packaging projects, and handling project documentation. By utilizing Maven, you can streamline the deployment process and ensure that all necessary dependencies are readily available.
Setting up the Maven POM file
Let's start by configuring the pom.xml
file to include the necessary dependencies for our Akka HTTP application. We'll need to ensure that the Akka HTTP libraries are included and any other dependencies are properly managed.
<project>
<!-- Other configurations -->
<dependencies>
<!-- Akka HTTP -->
<dependency>
<groupId>com.typesafe.akka</groupId>
<artifactId>akka-http_2.12</artifactId>
<version>10.2.6</version>
</dependency>
<!-- Other dependencies -->
</dependencies>
<!-- Other configurations -->
</project>
By declaring the Akka HTTP dependency within the pom.xml
file, Maven will automatically resolve and download the required libraries, ensuring that the application has access to the necessary components during both development and deployment.
Containerizing with Docker
Docker is a leading containerization platform that enables developers to package applications and their dependencies into standardized units called containers. These containers can be easily deployed across different environments, providing consistency and portability for your applications.
Creating a Dockerfile for Akka HTTP
To containerize our Akka HTTP application, we'll create a Dockerfile
that defines the steps to build the Docker image. This will include copying the application code, managing dependencies, and exposing the necessary ports for communication.
# Use a base image with Java
FROM openjdk:11
# Set the working directory in the container
WORKDIR /app
# Copy the JAR file into the container
COPY target/akka-http-app.jar /app
# Expose the port
EXPOSE 8080
# Define the command to run the application
CMD ["java", "-jar", "akka-http-app.jar"]
By specifying the base image, copying the application JAR file, and exposing the required ports, the Dockerfile
provides the blueprint for creating a container that encapsulates our Akka HTTP application.
Building and Running the Docker Image
With our Dockerfile
in place, we can build the Docker image using the following command:
docker build -t akka-http-app .
Once the image is built, we can run a container based on this image using:
docker run -p 8080:8080 akka-http-app
Automating Deployment with Maven and Docker
To streamline the deployment process for our Akka HTTP application, we can leverage the power of Maven and Docker in combination. By integrating Docker commands into the Maven build lifecycle, we can automate the building, tagging, and pushing of Docker images as part of the Maven build process.
Using the Spotify Docker Plugin
The Spotify Docker Plugin is a Maven plugin that provides a set of goals to work with Docker images and containers. We can utilize this plugin to seamlessly integrate Docker tasks within our Maven project.
Let's configure the pom.xml
to include the Spotify Docker Plugin:
<project>
<!-- Other configurations -->
<build>
<plugins>
<!-- Spotify Docker Plugin -->
<plugin>
<groupId>com.spotify</groupId>
<artifactId>docker-maven-plugin</artifactId>
<version>1.2.1</version>
<configuration>
<imageName>akka-http-app</imageName>
<imageTags>
<imageTag>${project.version}</imageTag>
</imageTags>
<baseImage>openjdk:11</baseImage>
<entryPoint>["java", "-jar", "/app/akka-http-app.jar"]</entryPoint>
<resources>
<resource>
<targetPath>/</targetPath>
<directory>${project.basedir}/target</directory>
<include>${project.build.finalName}.jar</include>
</resource>
</resources>
</configuration>
<executions>
<execution>
<phase>package</phase>
<goals>
<goal>build</goal>
</goals>
</execution>
</executions>
</plugin>
<!-- Other plugins -->
</plugins>
</build>
<!-- Other configurations -->
</project>
By configuring the Spotify Docker Plugin within the pom.xml
, we instruct Maven to build the Docker image during the package phase of the build lifecycle. The plugin specifications define the image name, tags, base image, entry point, and resources to be included in the image.
Streamlining Deployment with Maven and Docker
Now, when we run the Maven package command, the Spotify Docker Plugin will automatically build the Docker image based on our Dockerfile
, using the specified configuration. This seamless integration allows for a streamlined deployment process, eliminating the need for manual Docker commands and ensuring consistency across different environments.
By harnessing the capabilities of Maven and Docker, Java developers can achieve a smooth and efficient deployment pipeline for their Akka HTTP applications. The combined power of these tools enables automation, portability, and scalability, paving the way for seamless deployment in both development and production environments.
The Last Word
In this guide, we delved into the deployment of Akka HTTP applications using Maven and Docker. By leveraging Maven for dependency management and the Spotify Docker Plugin for seamless integration with Docker, Java developers can establish a robust deployment pipeline for their Akka HTTP projects. The integration of these tools facilitates automation, consistency, and scalability, empowering developers to deploy their applications with confidence and efficiency.
With a clear understanding of the principles and best practices outlined in this guide, Java developers can embrace the power of Akka HTTP, Maven, and Docker to elevate their deployment processes and deliver resilient, high-performance web applications.
Now it's your turn to put these insights into action and streamline the deployment of your Akka HTTP applications with Maven and Docker!