Solving Spring Boot Data Loss in REST Services
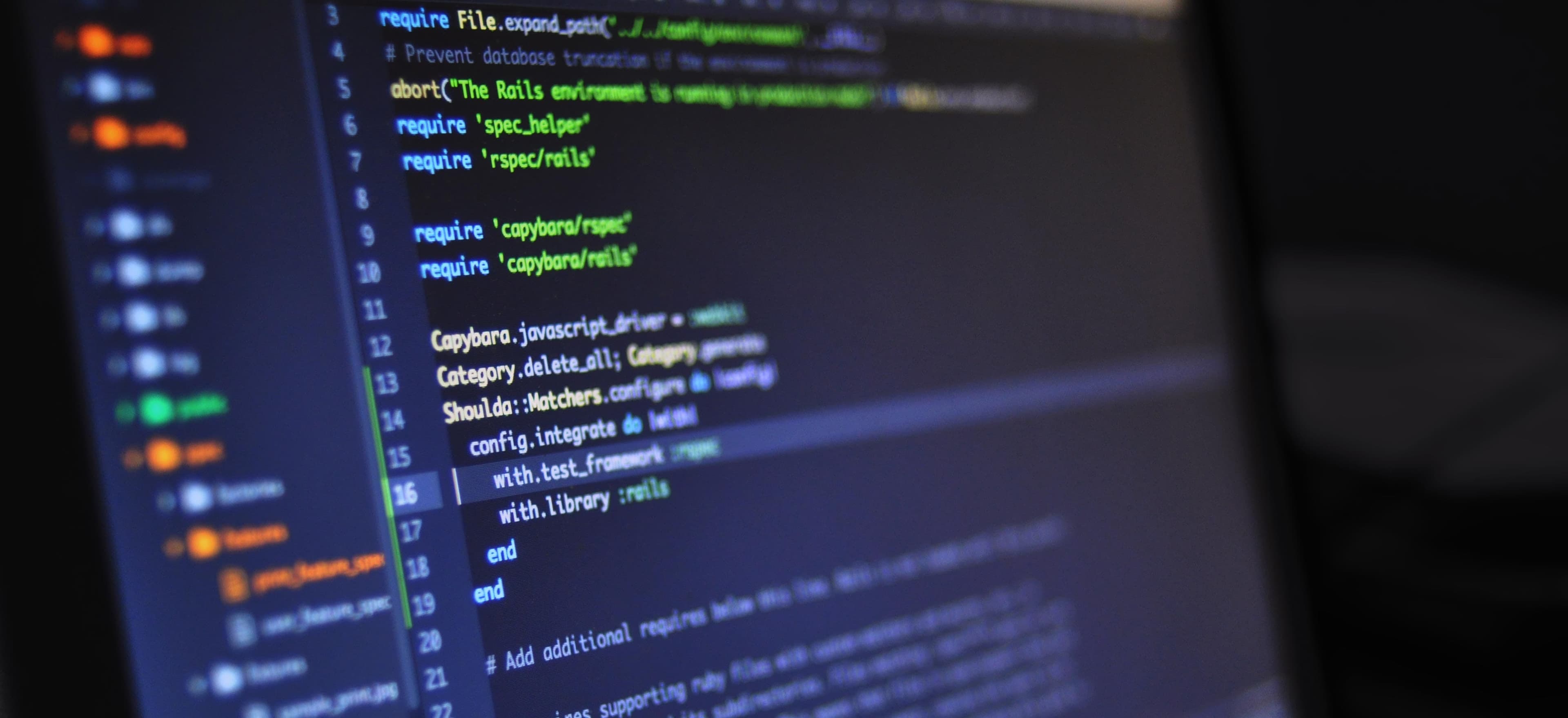
- Published on
Solving Spring Boot Data Loss in REST Services
When building a RESTful service using Spring Boot, data loss can sometimes occur due to improper handling of requests and responses. This can lead to inconsistencies in the application's state and data corruption. In this article, we will discuss some common reasons for data loss in Spring Boot REST services and explore solutions to prevent it.
Understanding Data Loss in REST Services
Data loss in REST services can occur due to a variety of reasons, including:
-
Incomplete or Incorrect Requests: When clients send incomplete or incorrect data in their requests, it can lead to data loss or corruption on the server side if not handled properly.
-
Unforeseen Exceptions: Unhandled exceptions during request processing can result in data loss if the transaction is not properly rolled back or if the error response is not communicated effectively.
-
Concurrency Issues: In a multi-threaded environment, concurrent requests and data manipulation can lead to data loss if synchronization and transaction management are not implemented correctly.
Now, let's dive into some practical solutions to mitigate these issues.
1. Validating Request Data
To prevent data loss caused by incomplete or incorrect requests, it's crucial to validate the incoming data before processing it. Spring Boot provides robust support for data validation through the use of annotations such as @Valid
and @NotNull
.
Here's an example of how you can validate request data in a REST controller:
@RestController
@RequestMapping("/api/users")
public class UserController {
@PostMapping
public ResponseEntity<User> createUser(@Valid @RequestBody User user) {
// Process the validated user data
}
}
In this example, the @Valid
annotation triggers validation of the User
object received in the request body. If the data is incomplete or invalid, Spring Boot will automatically return a 400 Bad Request response, preventing the incomplete data from causing data loss in the system.
2. Exception Handling with ControllerAdvice
Handling exceptions properly is essential to avoid data loss in REST services. By using Spring's @ControllerAdvice
alongside @ExceptionHandler
, you can centralize your exception handling and provide consistent error responses.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleGenericException(Exception ex) {
// Build and return an appropriate error response
}
@ExceptionHandler(DataIntegrityViolationException.class)
public ResponseEntity<ErrorResponse> handleDataIntegrityViolationException(DataIntegrityViolationException ex) {
// Handle data integrity violation and return an error response
}
}
In this example, the GlobalExceptionHandler
class handles generic exceptions as well as specific DataIntegrityViolationException
instances. By customizing error responses and handling various exception scenarios, you can prevent data loss and effectively communicate issues back to the client.
3. Transaction Management
Proper transaction management is crucial in preventing data loss, especially in scenarios involving multiple database operations. Spring Boot's declarative transaction management simplifies this process by using annotations such as @Transactional
.
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional
public void updateUser(User user) {
// Update user data in the database
}
}
In this example, the @Transactional
annotation ensures that the updateUser
method operates within a transactional context. If an exception occurs during the update operation, the transaction will be rolled back, preventing any data loss or inconsistency.
Key Takeaways
In a Spring Boot application, data loss in REST services can be mitigated through proper request data validation, exception handling, and transaction management. By incorporating these best practices, you can build robust and reliable RESTful services that maintain data integrity and consistency.
For further reading, you can explore the official Spring Boot documentation to deepen your understanding of these concepts.
Remember, ensuring data integrity and preventing data loss is not only an essential aspect of application reliability but also a critical component of maintaining user trust and satisfaction.
Checkout our other articles