Master Arquillian & WildFly 8 for Java EE 7 Unit Testing!
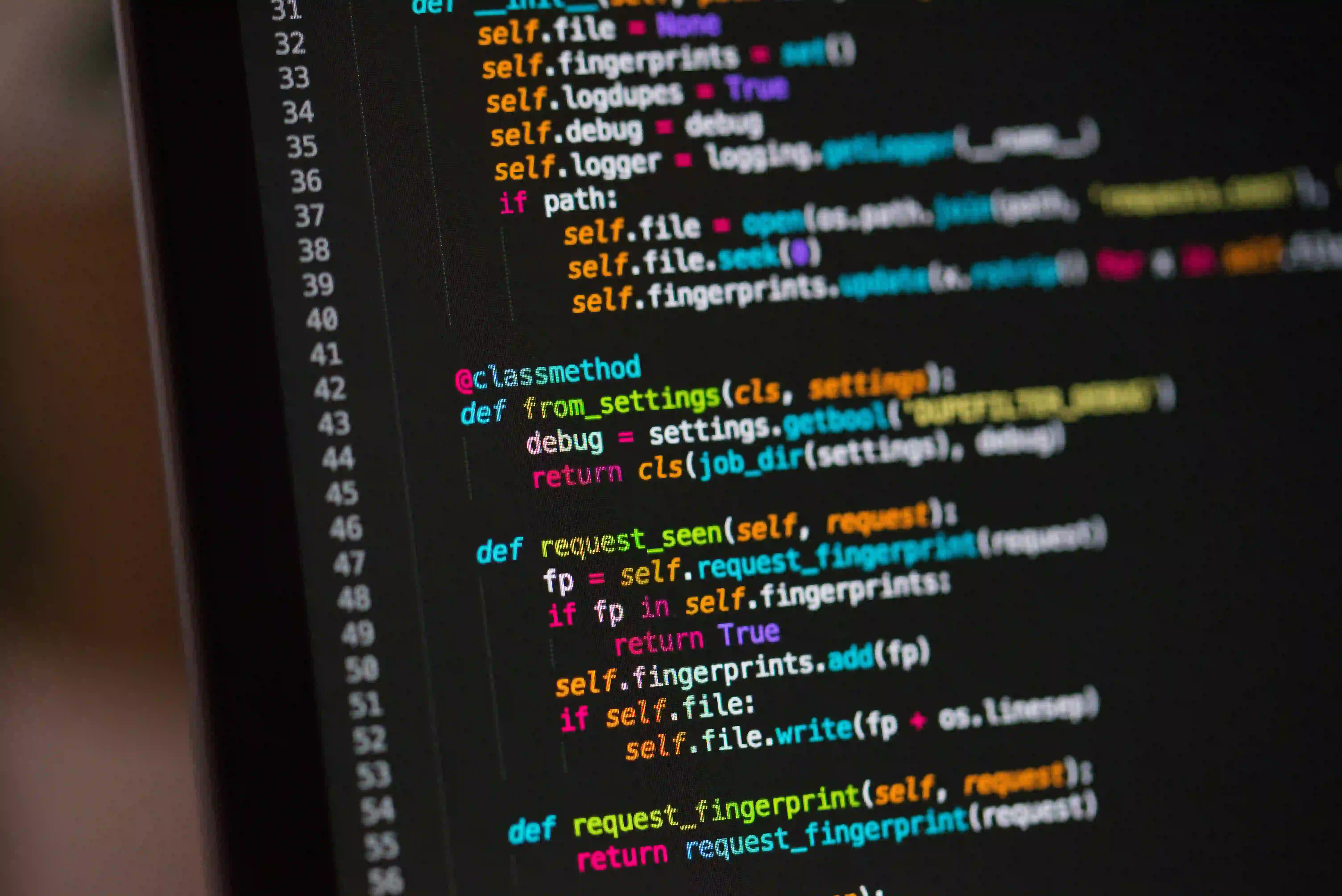
Mastering Arquillian & WildFly 8 for Java EE 7 Unit Testing
Unit testing is an essential part of the development process, ensuring the reliability and robustness of your code. In Java EE 7, the combination of Arquillian and WildFly 8 provides a powerful set of tools for writing effective unit tests. In this post, we will delve into the intricacies of utilizing these technologies to master unit testing in Java EE 7.
Understanding Arquillian and WildFly 8
Arquillian
Arquillian is an innovative and highly extensible testing platform that simplifies the integration testing of Java middleware. It allows developers to test their code in containers which makes it possible to test business logic and other Java EE components in a real environment, providing significant advantages over traditional unit testing frameworks.
Arquillian's extensive support for different containers and its seamless integration with popular Java IDEs makes it a compelling choice for Java EE developers.
WildFly 8
WildFly 8 is a lightweight, flexible, and fully-featured Java EE application server. It provides a fast, scalable, and modular platform for building and running Java applications. WildFly is known for its speed, manageability, and flexibility, making it an ideal choice for Java EE development and testing.
Setting Up the Environment
Before diving into unit testing with Arquillian and WildFly 8, it's crucial to set up the development environment properly. You can download and install WildFly 8 and Arquillian to get started.
Writing Your First Arquillian Test
Let's start by writing a simple Arquillian test to verify the behavior of a basic Java EE component. In this example, we'll write a test for a simple CDI (Contexts and Dependency Injection) bean.
@RunWith(Arquillian.class)
public class GreetingServiceTest {
@Deployment
public static WebArchive createDeployment() {
return ShrinkWrap.create(WebArchive.class)
.addClass(GreetingService.class)
.addAsWebInfResource(EmptyAsset.INSTANCE, "beans.xml");
}
@Inject
GreetingService greetingService;
@Test
public void testGreet() {
String message = greetingService.greet("World");
assertEquals("Hello, World!", message);
}
}
In the above code, we have written a simple JUnit test that is also an Arquillian test. One of the key features of Arquillian is the @Deployment
annotation, which allows us to define and configure the deployment archive for the test. This archive can include classes, resources, and configurations required for the test.
The @Inject
annotation is used to inject the GreetingService
instance into the test. We then proceed to write an assertion to validate the behavior of the greet
method.
Integrating WildFly 8 with Arquillian
Arquillian provides seamless integration with WildFly 8, allowing us to run tests in the container managed by WildFly. To achieve this, we need to configure the Arquillian deployment to include the necessary dependencies for WildFly 8.
<dependency>
<groupId>org.wildfly</groupId>
<artifactId>wildfly-arquillian-container-managed</artifactId>
<version>8.2.1.Final</version>
<scope>test</scope>
</dependency>
After including the dependency, Arquillian can now control the lifecycle of the WildFly 8 container and deploy the test archives into it, allowing for seamless integration testing.
Running the Arquillian Test with WildFly 8
To run the Arquillian test with WildFly 8, you need to configure the test to use the WildFly 8 container in the arquillian.xml
file. Here's an example of the configuration:
<container qualifier="wildfly" default="true">
<configuration>
<property name="jbossHome">/path/to/your/wildfly-8.2.1.Final</property>
</configuration>
</container>
With the setup complete, you can now run the Arquillian test, and it will deploy the test archive to the WildFly 8 container, execute the test, and report the results.
Final Thoughts
In conclusion, Arquillian and WildFly 8 provide a robust and efficient platform for unit testing Java EE 7 applications. Their seamless integration, flexibility, and support for different containers make them a preferred choice for Java EE developers.
By mastering Arquillian and WildFly 8 for unit testing in Java EE 7, developers can ensure the reliability and correctness of their code, leading to higher quality software. Embrace the power of these technologies, and elevate your unit testing game in Java EE 7 development!