Isolation: Overcoming Challenges in Test Attribute 10
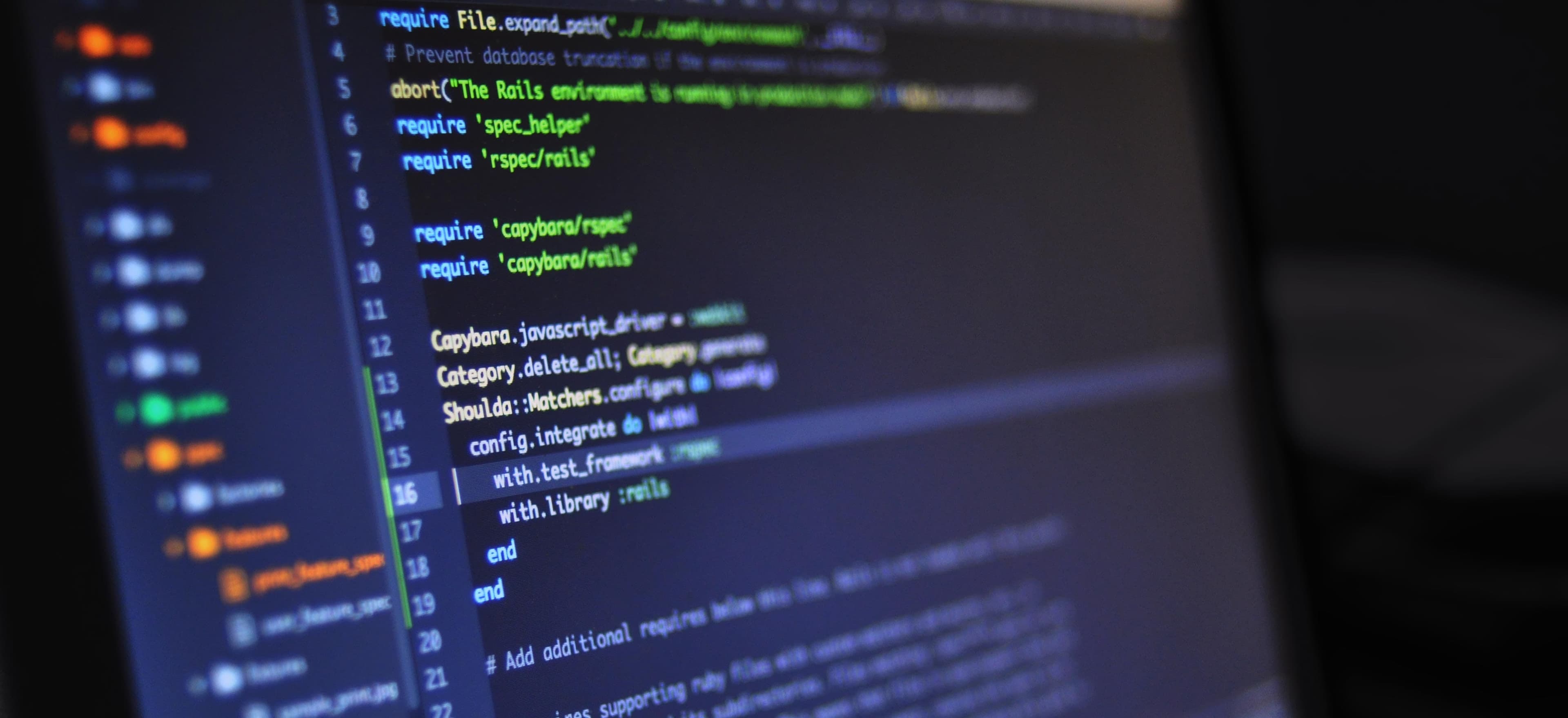
- Published on
Isolation: Overcoming Challenges in Test Attribute 10
Isolation in testing is a crucial aspect of ensuring that tests are independent and predictable. Test Attribute 10, part of the Java language, provides important isolation features that contribute to writing effective and reliable tests. In this blog post, we will explore the challenges of isolation in testing and how Test Attribute 10 can help overcome them.
The Importance of Isolation in Testing
Isolation refers to the ability to run tests independently of each other, without one test affecting the outcome of another. This is vital for producing reliable and repeatable test results. Challenges can arise when tests are not properly isolated, including:
-
Interference Between Tests: Without proper isolation, changes made by one test can impact the outcome of another test, leading to unpredictable results.
-
Dependency on External Factors: Tests relying on external resources such as databases, web services, or file systems can introduce variability and make tests harder to maintain.
-
Performance Issues: Lack of isolation can result in slower test execution times as tests contend for shared resources.
Introducing Test Attribute 10
Test Attribute 10, also known as @TestInstance
, is a feature introduced in JUnit 5 that addresses isolation challenges in testing. By default, JUnit creates a new instance of the test class for each test method, which can lead to unnecessary setup and teardown overhead. @TestInstance
allows tests to be configured with a lifecycle per TestInstance
or per TestMethod
. This can improve test performance and simplify setup and cleanup operations.
Using Test Attribute 10 for Improved Test Isolation
Let's take a look at how to use @TestInstance
to achieve improved test isolation. In the following example, we will demonstrate the usage of @TestInstance
at the class level.
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.TestInstance;
@TestInstance(TestInstance.Lifecycle.PER_CLASS)
public class IsolatedTest {
private DatabaseConnection databaseConnection;
@BeforeAll
public void setupDatabaseConnection() {
databaseConnection = new DatabaseConnection();
// Perform database setup
}
@AfterAll
public void closeDatabaseConnection() {
// Close the database connection
}
@Test
public void test1() {
// Test using databaseConnection
}
@Test
public void test2() {
// Another test using databaseConnection
}
}
In this example, the @TestInstance(TestInstance.Lifecycle.PER_CLASS)
annotation is used to specify that the test instance will be shared across all test methods within the test class. By doing this, we can set up the DatabaseConnection
once and share it between multiple test methods, thereby improving performance and reducing redundant setup code.
Why Use @TestInstance
?
The use of @TestInstance
provides several benefits:
-
Improved Performance: By reusing the same test instance across multiple test methods, the overhead of creating and destroying test instances for each method is reduced, leading to improved test execution times.
-
Reduced Setup Overhead: With shared test instances, setup code that only needs to run once can be placed in methods annotated with
@BeforeAll
and@AfterAll
, reducing redundancy and improving code maintainability. -
Simplified State Management: Shared test instances allow for the sharing of state between test methods, enabling more complex test scenarios to be managed effectively.
Closing the Chapter
In conclusion, isolation is a fundamental aspect of writing effective and reliable tests. Test Attribute 10 (@TestInstance
) in JUnit 5 provides a powerful mechanism for achieving improved test isolation, leading to more robust and maintainable test suites. By carefully utilizing @TestInstance
, developers can overcome the challenges associated with test isolation and produce high-quality tests that contribute to the overall stability and reliability of their Java applications.
As you delve deeper into the world of testing in Java, incorporating Test Attribute 10 as part of your toolset can prove to be beneficial in the long run for maintaining the health of your codebase, and instilling confidence in the quality of your software development.
For further information on JUnit 5 and its features, check out the official documentation and JUnit 5 User Guide.
So dive in and start leveraging the power of @TestInstance
to bolster your testing practices in Java! Happy testing!
Checkout our other articles