Tackling Backpressure in Spring WebFlux for Smooth Scaling
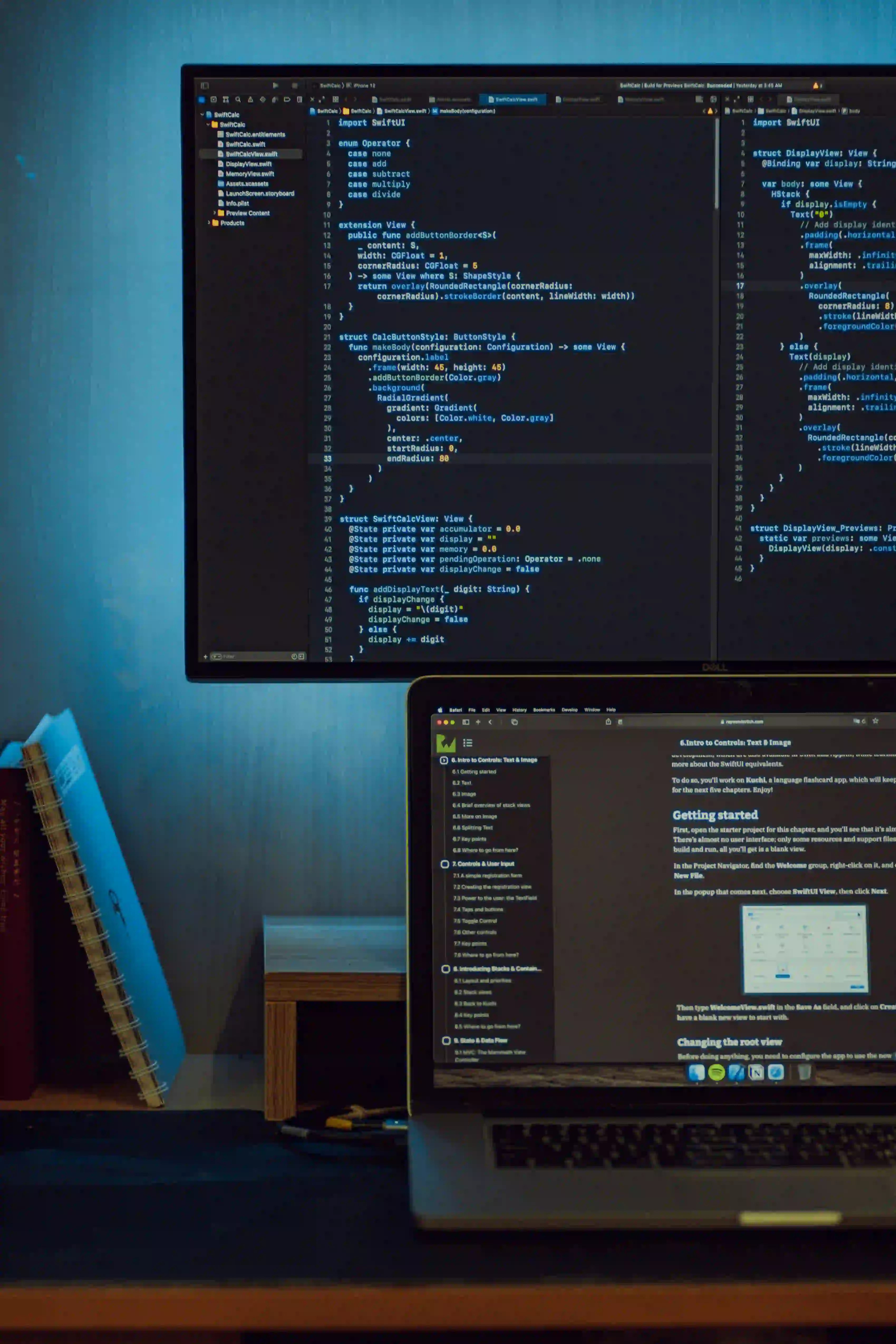
Tackling Backpressure in Spring WebFlux for Smooth Scaling
When building reactive applications in Java, backpressure is a crucial consideration. In reactive programming, backpressure occurs when the data source is producing data faster than the consumer can handle. This can lead to resource exhaustion, latency, and potential system failure. In this article, we'll explore how to tackle backpressure in Spring WebFlux for smooth scaling.
Understanding Backpressure
In traditional imperative programming, the producer typically pushes data to the consumer, and the consumer processes it as quickly as it can. However, in reactive programming, with the concept of backpressure, the consumer can signal to the producer how much data it can handle, thus controlling the flow of data.
Handling Backpressure in Spring WebFlux
Spring WebFlux, with its reactive programming model, provides tools to handle backpressure efficiently. Let's take a look at some strategies for tackling backpressure in Spring WebFlux:
Strategy 1: Buffering
One way to handle backpressure is through buffering. When the data source is producing data faster than the consumer can handle, buffering the excess data can prevent loss. In Spring WebFlux, you can use the onBackpressureBuffer
operator to buffer the data.
Flux<Data> dataStream = getDataStream()
.onBackpressureBuffer(100, BufferOverflowStrategy.DROP_OLDEST);
In this example, the onBackpressureBuffer
operator creates a buffer with a capacity of 100 elements and a strategy of dropping the oldest elements if the buffer overflows.
Strategy 2: Dropping
In some scenarios, it may be acceptable to drop the excess data when backpressure occurs. This can be achieved using the onBackpressureDrop
operator in Spring WebFlux.
Flux<Data> dataStream = getDataStream()
.onBackpressureDrop(data -> log.info("Dropping data: {}", data));
The onBackpressureDrop
operator drops the excess data and allows you to log or handle the dropped data as needed.
Strategy 3: Error Handling
When backpressure is not manageable through buffering or dropping, you can handle it as an error using the onBackpressureError
operator.
Flux<Data> dataStream = getDataStream()
.onBackpressureError();
The onBackpressureError
operator signals backpressure as an error, allowing you to handle it using error-handling operators in Spring WebFlux.
Scaling Strategies with Backpressure
In addition to handling backpressure, it's essential to consider scaling strategies in reactive applications. When dealing with backpressure, scaling out by adding more instances of the application can help distribute the load and handle the data flow more efficiently.
Strategy 1: Load Balancing
By using a load balancer, you can distribute the incoming data across multiple instances of your Spring WebFlux application. This helps prevent a single instance from being overwhelmed by backpressure and improves the overall throughput.
Strategy 2: Reactive Caching
Utilizing reactive caching mechanisms, such as Spring's @Cacheable
annotation with a reactive cache manager, can help reduce the impact of backpressure by serving cached data when the original data source experiences backpressure.
The Bottom Line
In conclusion, tackling backpressure in Spring WebFlux is essential for building scalable and resilient reactive applications. By utilizing buffering, dropping, error handling, and scaling strategies, you can effectively manage backpressure and ensure smooth scaling of your Spring WebFlux applications.
Adopting these strategies will not only enhance the performance and resilience of your reactive applications, but also provide a better experience for your end users. Stay reactive, stay scalable with Spring WebFlux!
To dive deeper into Spring WebFlux and backpressure handling, check out the official documentation here.
Happy coding!