Stop Missing Out: Real-Time Alerts for S3 Uploads Explained
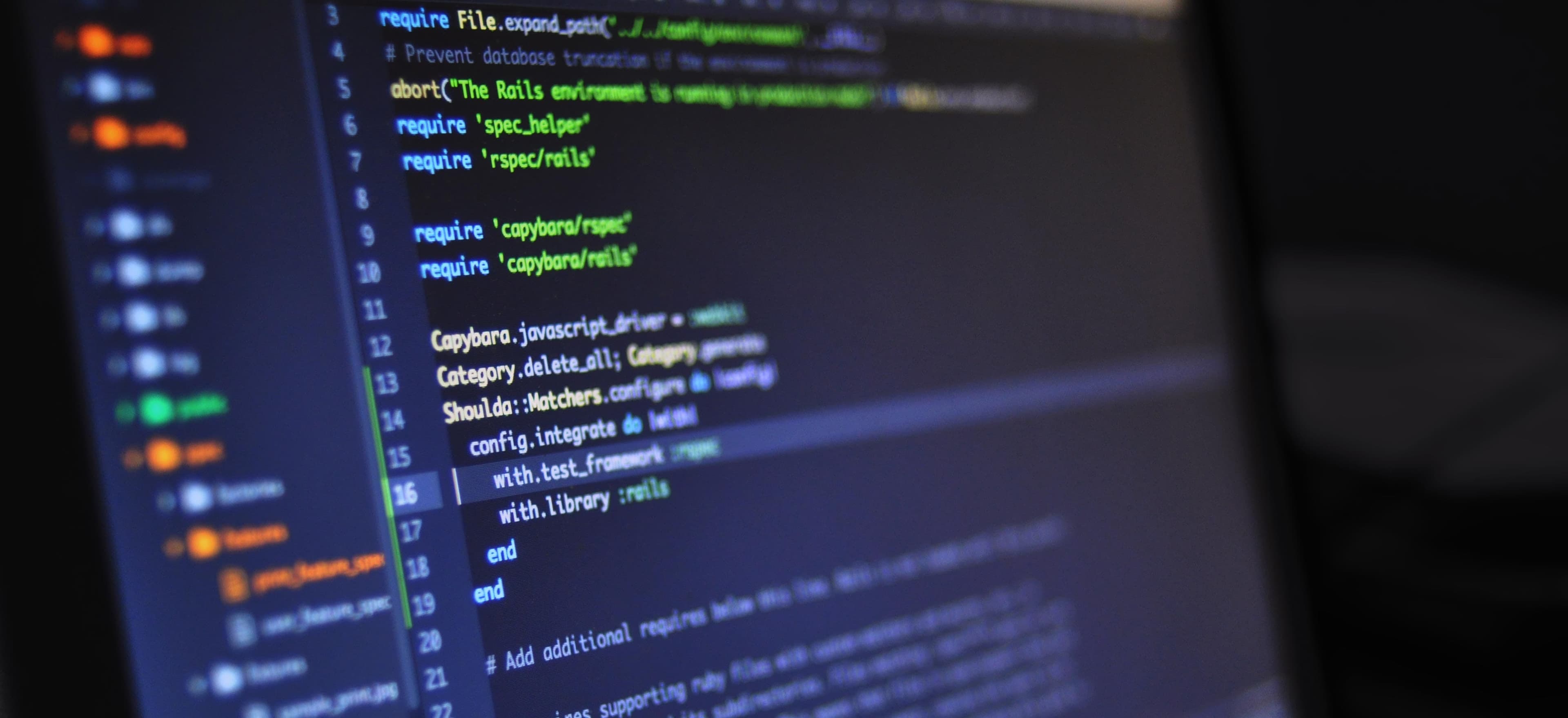
- Published on
Real-Time Alerts for S3 Uploads with Java
Have you ever wished to receive real-time alerts whenever a file is uploaded to your Amazon S3 bucket? In this blog post, we will dive into how you can achieve this using Java and the AWS SDK to set up real-time alerts for S3 uploads. By the end of this guide, you will have a robust solution that can send notifications the moment a file is uploaded to your S3 bucket.
Prerequisites
Before jumping into the implementation, make sure you have the following prerequisites in place:
- Java Development Kit (JDK) installed
- AWS account with access to Amazon S3
- AWS SDK for Java
Setting up the Project
First, let's set up a new Java project, either using your favorite IDE or a build tool such as Maven or Gradle.
If you're using Maven, add the following dependency to your pom.xml
file:
<dependency>
<groupId>software.amazon.awssdk</groupId>
<artifactId>s3</artifactId>
</dependency>
For Gradle, include the following in your build.gradle
file:
implementation 'software.amazon.awssdk:s3'
Creating Real-Time Alerts
To create real-time alerts for S3 uploads, we will leverage the Amazon S3 event notifications. This feature allows us to set up event notifications that send alerts when specific actions occur in our S3 bucket, such as object creation.
Let's start by creating an instance of the S3Client
and configuring the S3 event notification using the AWS SDK for Java.
import software.amazon.awssdk.services.s3.S3Client;
import software.amazon.awssdk.services.s3.model.*;
public class S3RealTimeAlerts {
public static void main(String[] args) {
S3Client s3Client = S3Client.builder().build();
S3BucketNotificationConfiguration notificationConfiguration = S3BucketNotificationConfiguration.builder()
.notificationConfiguration(NotificationConfiguration.builder()
.queueConfigurations(QueueConfiguration.builder()
.queueArn("arn:aws:sqs:us-east-1:123456789012:my-queue")
.events(S3Event.OBJECT_CREATED)
.build())
.build())
.build();
s3Client.putBucketNotificationConfiguration(PutBucketNotificationConfigurationRequest.builder()
.bucket("my-bucket")
.notificationConfiguration(notificationConfiguration)
.build());
}
}
In the above code, we create an instance of the S3Client
and configure the S3 event notification to send alerts to the specified Amazon SQS queue whenever an object is created in the S3 bucket.
Why This Works
By using the AWS SDK for Java, we are able to interact with the Amazon S3 service programmatically. The S3Client
allows us to communicate with S3 and perform operations such as setting up bucket event notifications.
The S3BucketNotificationConfiguration
and NotificationConfiguration
classes provide a structured way to define the notification settings. In this case, we specify that we want to send notifications to an SQS queue for the OBJECT_CREATED
event.
Receiving Real-Time Alerts
Now that we have set up the S3 event notification, let's explore how we can receive real-time alerts when a file is uploaded to our S3 bucket.
We will create a simple SQS listener using the AWS SDK for Java to receive messages from the SQS queue we configured in the S3 event notification.
import software.amazon.awssdk.services.sqs.SqsClient;
import software.amazon.awssdk.services.sqs.model.*;
public class S3SQSListener {
public static void main(String[] args) {
SqsClient sqsClient = SqsClient.builder().build();
ReceiveMessageRequest receiveMessageRequest = ReceiveMessageRequest.builder()
.queueUrl("https://sqs.us-east-1.amazonaws.com/123456789012/my-queue")
.build();
List<Message> messages = sqsClient.receiveMessage(receiveMessageRequest).messages();
for (Message message : messages) {
System.out.println("Received message: " + message.body());
// Add your alerting logic here
}
}
}
In this code snippet, we create an instance of the SqsClient
and use it to receive messages from the specified SQS queue. Upon receiving messages, you can add your custom logic to handle the alerts according to your requirements.
Why This Matters
By using the AWS SDK for Java to create an SQS client, we can seamlessly integrate with Amazon SQS to receive messages from the queue. This allows us to process the real-time alerts triggered by S3 uploads and take appropriate actions based on the received messages.
Putting It All Together
Now that we have set up the S3 event notification and created an SQS listener to receive real-time alerts, let's see how both pieces fit together in a complete scenario.
public class S3RealTimeAlertsDemo {
public static void main(String[] args) {
// Set up S3 event notification
S3Client s3Client = S3Client.builder().build();
S3BucketNotificationConfiguration notificationConfiguration = S3BucketNotificationConfiguration.builder()
.notificationConfiguration(NotificationConfiguration.builder()
.queueConfigurations(QueueConfiguration.builder()
.queueArn("arn:aws:sqs:us-east-1:123456789012:my-queue")
.events(S3Event.OBJECT_CREATED)
.build())
.build())
.build();
s3Client.putBucketNotificationConfiguration(PutBucketNotificationConfigurationRequest.builder()
.bucket("my-bucket")
.notificationConfiguration(notificationConfiguration)
.build());
// Create SQS listener
SqsClient sqsClient = SqsClient.builder().build();
ReceiveMessageRequest receiveMessageRequest = ReceiveMessageRequest.builder()
.queueUrl("https://sqs.us-east-1.amazonaws.com/123456789012/my-queue")
.build();
List<Message> messages = sqsClient.receiveMessage(receiveMessageRequest).messages();
for (Message message : messages) {
System.out.println("Received message: " + message.body());
// Add your alerting logic here
}
}
}
Running the S3RealTimeAlertsDemo
class will set up the S3 event notification and begin listening for real-time alerts on the SQS queue. Any file uploads to the specified S3 bucket will trigger messages to be sent to the SQS queue, which will be subsequently received and processed by the SQS listener.
A Final Look
In this blog post, we've explored how to implement real-time alerts for S3 uploads using Java and the AWS SDK. By leveraging the S3 event notification feature and integrating with Amazon SQS, we can proactively receive alerts the moment files are uploaded to our S3 bucket. This has various applications, from building real-time monitoring systems to triggering automated workflows based on incoming data.
Now that you have a solid understanding of this process, consider exploring further customization and integrating the real-time alerts into your existing systems. Embrace the power of real-time notifications to stay ahead in your AWS S3 workflows!