Overcoming API Challenges in Android Weather Apps
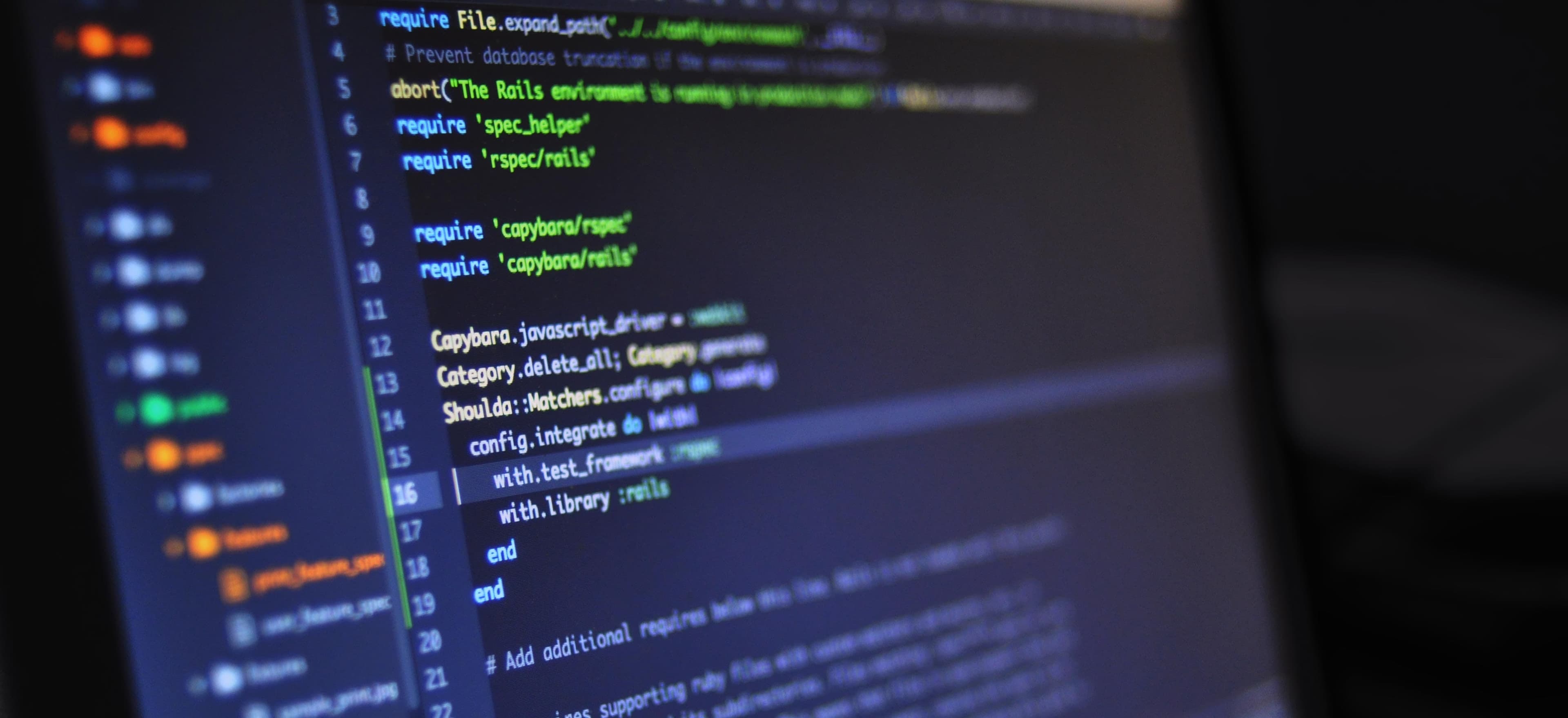
- Published on
The Challenge of Working with APIs in Android Weather Apps
When developing Android weather apps, one of the most vital components is the integration with external APIs to fetch weather data. However, integrating APIs into Android apps can be challenging due to various factors such as network connectivity, data parsing, and handling asynchronous operations. In this blog post, we will explore the common challenges faced when working with APIs in Android weather apps and how to overcome them using Java.
1. Handling Network Connectivity
Code Example: Checking Network Connectivity
ConnectivityManager connectivityManager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo activeNetwork = connectivityManager.getActiveNetworkInfo();
boolean isConnected = activeNetwork != null && activeNetwork.isConnectedOrConnecting();
if (isConnected) {
// Make API request
} else {
// Show error message for no internet connection
}
When making API calls in Android weather apps, it is crucial to handle network connectivity to ensure that the device is connected to the internet. By using the ConnectivityManager
class, we can check the network connectivity status before making any API requests. In case of no internet connection, appropriate error messages should be displayed to the user.
2. Data Parsing and Model Mapping
Code Example: Parsing JSON Response
JSONObject jsonResponse = new JSONObject(response);
String location = jsonResponse.getString("location");
JSONArray forecastArray = jsonResponse.getJSONArray("forecast");
List<Weather> weatherList = new ArrayList<>();
for (int i = 0; i < forecastArray.length(); i++) {
JSONObject forecastObject = forecastArray.getJSONObject(i);
String date = forecastObject.getString("date");
String condition = forecastObject.getString("condition");
// Create Weather object and add to list
Weather weather = new Weather(date, condition);
weatherList.add(weather);
}
When the weather data is fetched from the API, it is usually in a structured format such as JSON. Parsing this data into meaningful objects involves extracting relevant fields and mapping them to the app's model classes. In the above example, the JSON response is parsed to extract location and forecast information, which is then mapped to a Weather
model.
3. Asynchronous Operations and Threading
Code Example: Making Asynchronous API Call
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(apiUrl)
.build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
// Handle failure
}
@Override
public void onResponse(Call call, Response response) throws IOException {
// Handle response
}
});
API calls in Android apps should be performed asynchronously to avoid blocking the main UI thread. Using libraries like OkHttp allows us to make asynchronous network requests easily. The enqueue
method performs the API call in the background and provides callbacks for handling success or failure responses.
4. Error Handling and Feedback to Users
Code Example: Handling API Errors
@Override
public void onResponse(Call call, Response response) throws IOException {
if (response.isSuccessful()) {
// Process successful response
} else {
// Handle API error
}
}
When working with APIs, it's essential to handle errors gracefully and provide meaningful feedback to the users. In the onResponse
callback, we can check if the API call was successful and process the response accordingly. If the call is unsuccessful, appropriate error handling should be implemented to communicate the issue to the user.
5. Caching and Data Persistence
Code Example: Caching API Responses
Cache cache = new Cache(context.getCacheDir(), CACHE_SIZE);
OkHttpClient client = new OkHttpClient.Builder()
.cache(cache)
.build();
Caching API responses can improve the performance and user experience of the weather app by reducing the need to make frequent network requests. By leveraging the Cache
class in conjunction with OkHttp client, we can cache API responses and serve the cached data when the same API request is made again within a certain timeframe.
Closing the Chapter
Developing Android weather apps that seamlessly integrate with APIs requires overcoming several challenges, including handling network connectivity, parsing data, managing asynchronous operations, error handling, and implementing caching strategies. By employing the discussed strategies and code examples in Java, developers can enhance the reliability and responsiveness of their weather apps, providing users with accurate and up-to-date weather information.
By mastering these key aspects of API integration, Android weather app developers can ensure the seamless operation and reliability of their applications, providing users with accurate and up-to-date weather information regardless of network conditions and other potential challenges. Utilizing Java and the techniques discussed in this post will enable developers to build robust, user-friendly weather apps that deliver an exceptional experience to users.
For further reading, consider exploring the official Android Developer Documentation on Network Operations and JSON Parsing.
Happy coding!
Checkout our other articles