Dockerizing Rails Apps: A Guide to Smooth Migration
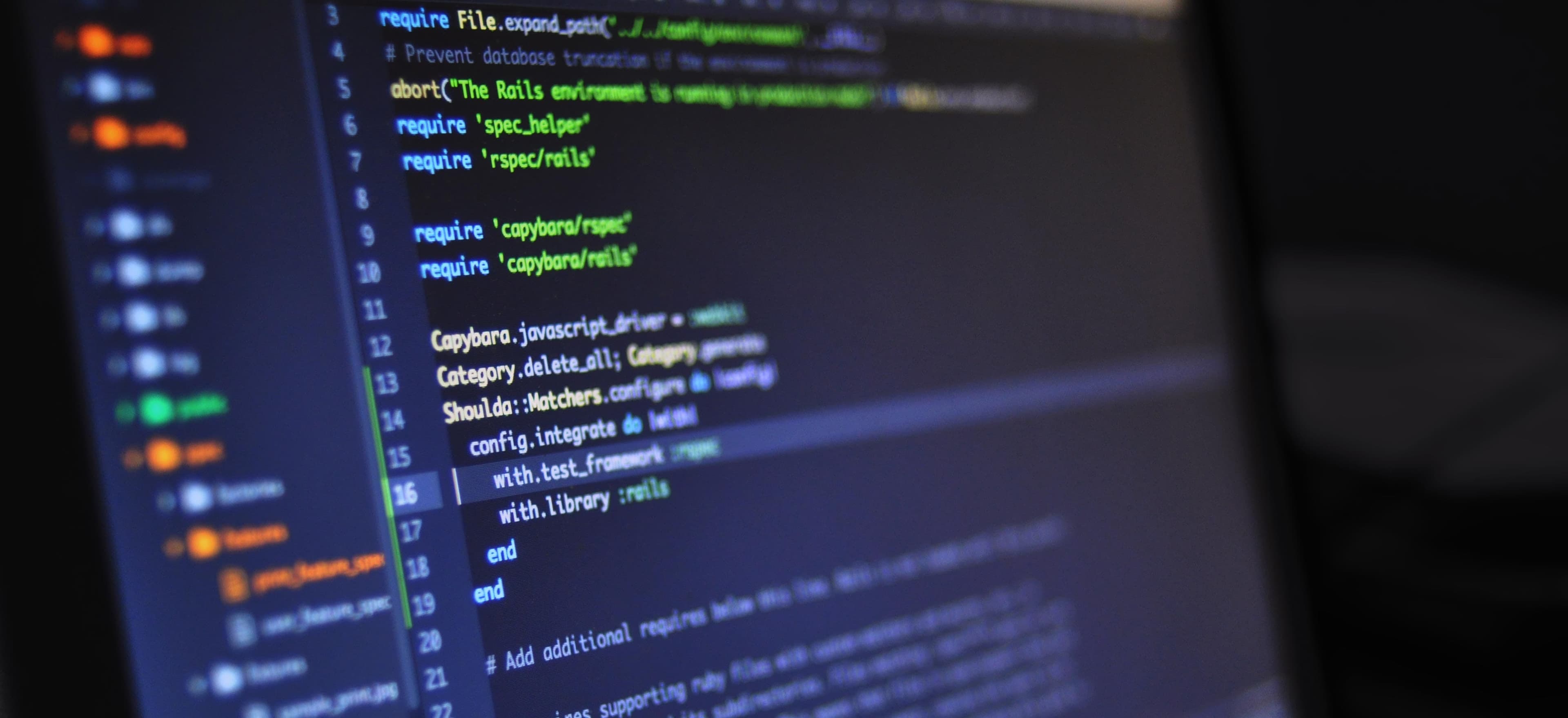
- Published on
Dockerizing Rails Apps: A Guide to Smooth Migration
As Rails applications grow in complexity, managing dependencies and environments becomes increasingly challenging. Docker provides a solution by enabling developers to encapsulate their Rails applications, runtime, and dependencies into a consistent environment. In this guide, we'll explore the process of Dockerizing a Rails application, highlighting the benefits and best practices along the way.
Why Dockerize a Rails App?
Dockerizing a Rails application offers several advantages:
-
Consistent Development Environment: Docker ensures that all developers work in the same environment, minimizing the "it works on my machine" problem.
-
Simplified Deployment: With Docker, you can deploy your Rails application along with its dependencies as a single unit, reducing configuration errors in production.
-
Scalability: Docker containers are lightweight and can be easily scaled horizontally to handle increased traffic.
-
Isolation: Each Docker container encapsulates a specific component of the application, ensuring isolation and security.
Now, let's dive into the steps to Dockerize a Rails application.
Step 1: Dockerfile Configuration
The first step is to create a Dockerfile
in the root directory of the Rails application. This file will define the environment and dependencies for the application.
# Use an official Ruby runtime as the base image
FROM ruby:2.6.3
# Set the working directory in the container
WORKDIR /app
# Install dependencies
COPY Gemfile Gemfile.lock ./
RUN bundle install
# Copy the Rails application into the container
COPY . .
# Expose the port on which the Rails app will run
EXPOSE 3000
# Command to start the Rails application
CMD ["rails", "server", "-b", "0.0.0.0"]
In this Dockerfile
, we start by specifying the base image as Ruby 2.6.3. We then set the working directory, install the Ruby dependencies, copy the Rails application into the container, expose the port 3000 for the Rails server, and define the command to start the Rails application.
Step 2: Docker Compose for Multi-Container Setup
For complex Rails applications that require additional services like a database, using Docker Compose is a best practice. It allows you to define and run multi-container Docker applications.
Create a docker-compose.yml
file in the root directory of the application with the following configuration:
version: '3'
services:
db:
image: postgres:12
volumes:
- ./tmp/db:/var/lib/postgresql/data
environment:
POSTGRES_DB: myapp_development
POSTGRES_USER: postgres
POSTGRES_PASSWORD: password
web:
build: .
command: bash -c "rm -f tmp/pids/server.pid && bundle exec rails s -p 3000 -b '0.0.0.0'"
volumes:
- .:/app
ports:
- "3000:3000"
depends_on:
- db
In this docker-compose.yml
file, we define two services: db
for the PostgreSQL database and web
for the Rails application. The web
service builds from the current directory, specifies the command to start the Rails server, and maps the ports.
Step 3: Building and Running the Docker Containers
With the Dockerfile
and docker-compose.yml
in place, you can build and run the Docker containers using the following commands:
docker-compose build
docker-compose up
The docker-compose build
command builds the services defined in the docker-compose.yml
, and docker-compose up
starts the services.
The Last Word
Dockerizing a Rails application streamlines development, simplifies deployment, and enhances scalability and isolation. While the initial setup may seem daunting, the benefits far outweigh the effort. By following the steps outlined in this guide, you can smoothly migrate your Rails application to a Dockerized environment, unlocking its full potential in modern containerized infrastructure.
To further enhance your understanding of Docker and Rails, I recommend exploring Docker's official documentation for in-depth insights and best practices, as well as the official Rails guides for comprehensive information on building and deploying Rails applications. Happy Dockerizing!