Boost Your GWT App Security with Captcha Integration
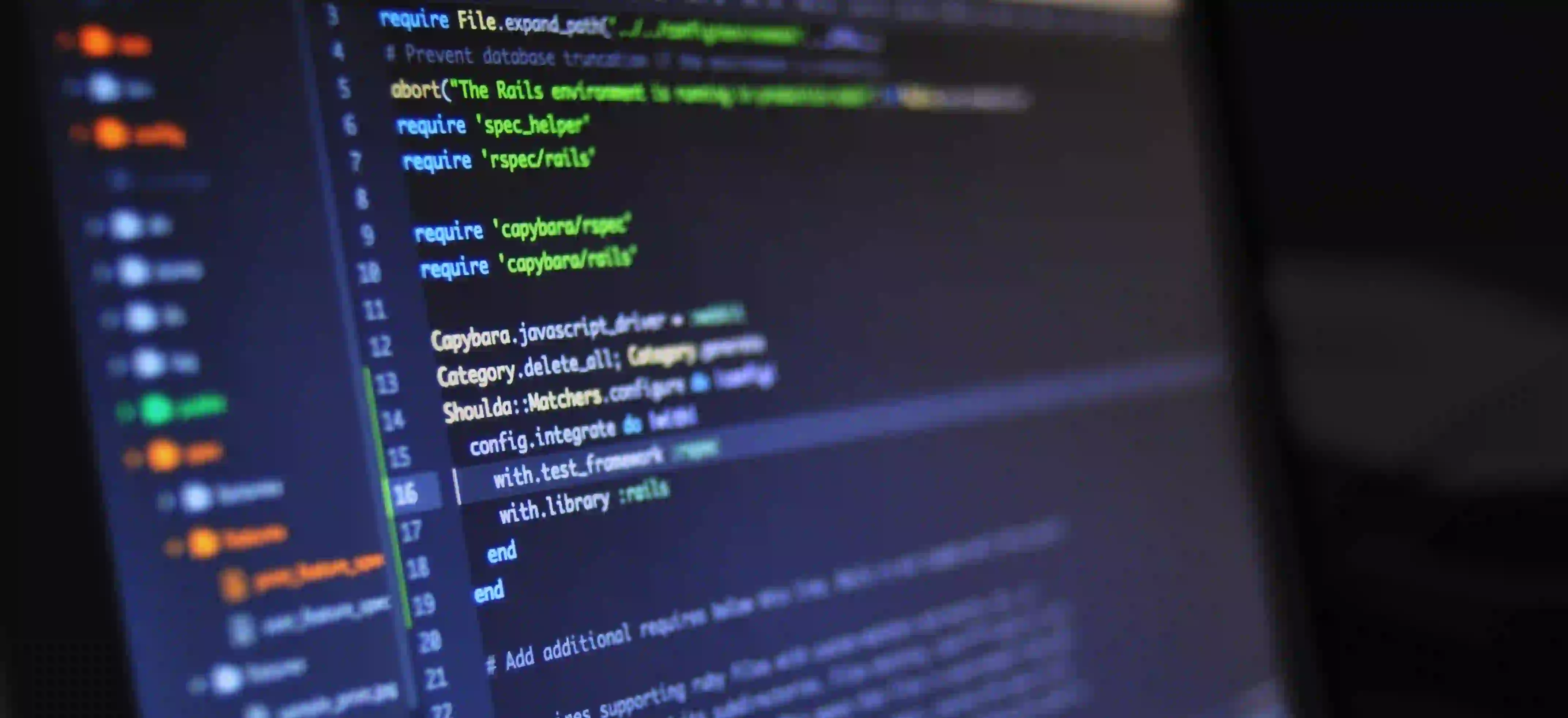
Boost Your GWT App Security with Captcha Integration
When developing a web application, ensuring security is paramount. One of the most effective ways to enhance security is by integrating CAPTCHA into your application. CAPTCHA, which stands for "Completely Automated Public Turing test to tell Computers and Humans Apart," helps to prevent bots and automated scripts from carrying out malicious activities on your website. In this article, we'll explore how to integrate CAPTCHA into a Google Web Toolkit (GWT) application to bolster its security.
What is GWT?
GWT is an open-source web development framework created by Google that allows developers to build and optimize complex browser-based applications. It enables developers to write client-side Java code and then compile it into highly optimized JavaScript. GWT provides a rich set of tools and libraries for creating interactive web applications, making it a popular choice for developers.
Why Integrate CAPTCHA?
Integrating CAPTCHA into your GWT application can significantly reduce the risk of automated attacks such as spamming, brute force login attempts, and other forms of abuse. By adding CAPTCHA to key areas of your application, such as user registration, login, and forms, you can effectively block bot-based abuse and enhance overall security.
Getting Started with CAPTCHA Integration in GWT
Step 1: Choose a CAPTCHA Service Provider
There are several CAPTCHA service providers available, each offering their own set of features and capabilities. Popular choices include Google reCAPTCHA, hCaptcha, and CAPTCHA.net. For this tutorial, we'll focus on integrating Google reCAPTCHA due to its widespread use and robust security features.
Step 2: Obtain API Keys
To get started with Google reCAPTCHA, you'll need to obtain site and secret keys by registering your website with Google's reCAPTCHA service. This can be done through the Google reCAPTCHA admin console. Once registered, you'll receive unique site and secret keys that will be used to integrate reCAPTCHA into your GWT application.
Step 3: Add the reCAPTCHA Widget to Your GWT Application
You can integrate the reCAPTCHA widget by adding the necessary JavaScript and configuring it to interact with your GWT code. Here's an example of how you can add the reCAPTCHA widget to your GWT application:
import com.google.gwt.core.client.EntryPoint;
import com.google.gwt.user.client.ui.RootPanel;
import com.google.gwt.user.client.ui.HTML;
public class MyGWTApplication implements EntryPoint {
public void onModuleLoad() {
// Add the reCAPTCHA widget to the desired location in your application
String siteKey = "YOUR_SITE_KEY"; // Replace with your actual site key
String recaptchaScript = "<script src='https://www.google.com/recaptcha/api.js' async defer></script>";
String recaptchaWidget = "<div class='g-recaptcha' data-sitekey='" + siteKey + "'></div>";
RootPanel.get("recaptchaContainer").add(new HTML(recaptchaScript + recaptchaWidget));
}
}
In this code snippet, we're adding the reCAPTCHA widget to the recaptchaContainer
in our GWT application. We fetch the site key obtained from the Google reCAPTCHA admin console and use it to configure the reCAPTCHA widget.
Step 4: Validate the reCAPTCHA Response on the Server Side
After integrating the reCAPTCHA widget into your GWT application, the next step is to validate the user's response on the server side to ensure that it's not coming from a bot. Google provides an API endpoint for this purpose. Here's an example of how you can validate the reCAPTCHA response on the server side using a simple servlet:
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class RecaptchaValidatorServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String recaptchaResponse = request.getParameter("g-recaptcha-response");
String secretKey = "YOUR_SECRET_KEY"; // Replace with your actual secret key
String url = "https://www.google.com/recaptcha/api/siteverify?secret=" + secretKey + "&response=" + recaptchaResponse;
InputStream res = new URL(url).openStream();
JsonObject json = (JsonObject) JsonParser.parseReader(new InputStreamReader(res, "UTF-8"));
boolean success = json.get("success").getAsBoolean();
if (success) {
// CAPTCHA validation successful
// Proceed with your application's logic
} else {
// CAPTCHA validation failed
// Handle accordingly, e.g., show an error message to the user
}
}
}
In this code, we retrieve the user's reCAPTCHA response from the request and validate it by making a call to Google's reCAPTCHA verification endpoint. Based on the validation result, you can then proceed with your application's logic, such as allowing the user to submit a form or displaying an error message.
A Final Look
Incorporating CAPTCHA into your GWT application is a crucial step towards enhancing its security and protecting it from automated attacks. By following the steps outlined in this article, you can seamlessly integrate Google reCAPTCHA into your GWT application, thereby fortifying its defenses against bots and automated scripts.
Remember, while CAPTCHA is a powerful tool for thwarting automated abuse, it's essential to combine it with other security measures such as input validation, multi-factor authentication, and rate limiting to ensure comprehensive protection for your application.
So, why wait? Take the proactive step to integrate CAPTCHA into your GWT application today and safeguard it from malicious bot activities!