Simplify XML: Swap JAXB for Xembly & Ease Your Coding Pain!
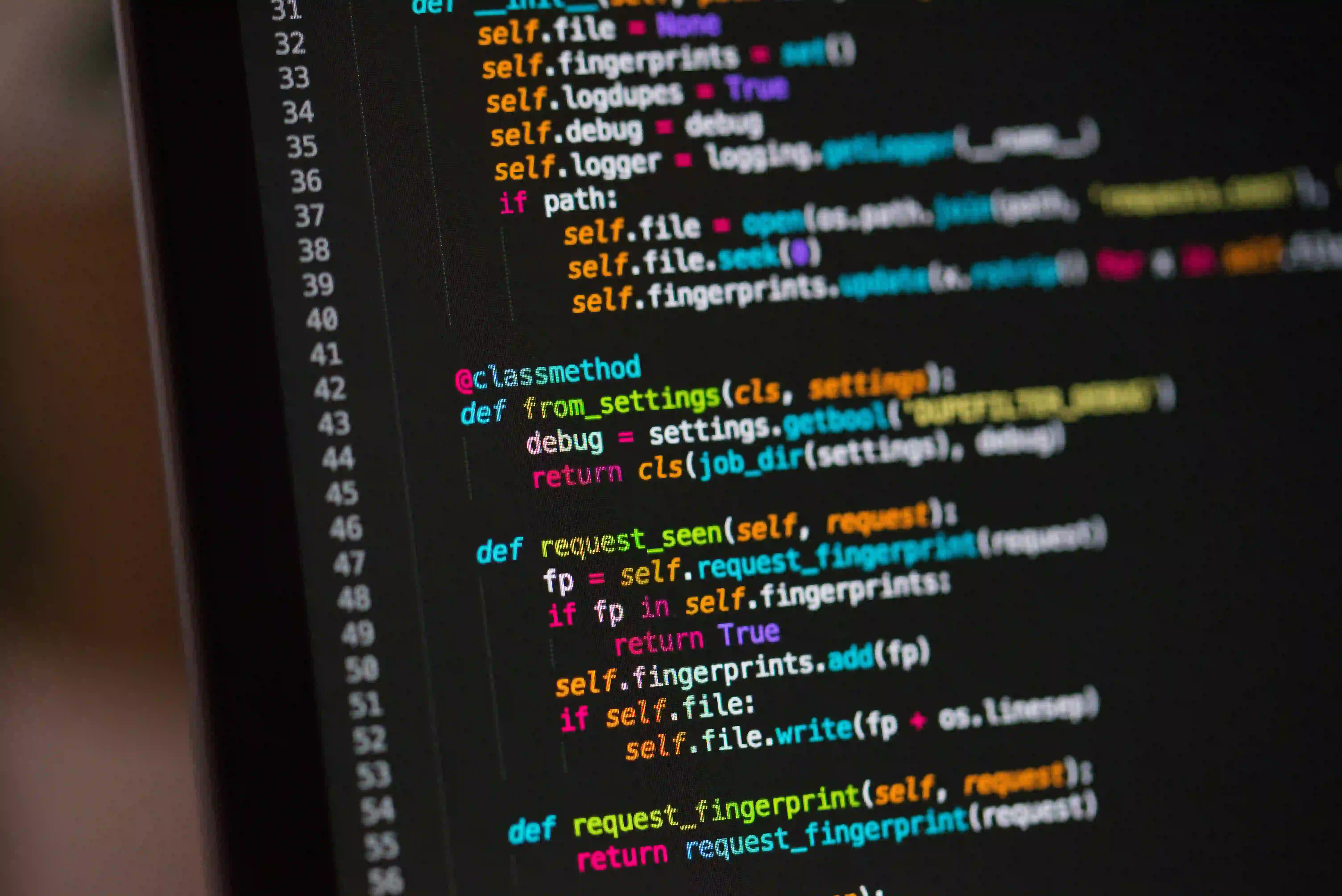
Simplify XML: Swap JAXB for Xembly & Ease Your Coding Pain!
As a Java developer, you are likely familiar with the challenges of working with XML data. While JAXB (Java Architecture for XML Binding) has been a popular choice for XML binding, it can be complex and verbose, leading to tedious and error-prone code. However, there’s a better way to simplify XML manipulation in Java - Xembly.
In this article, we’ll explore how you can swap JAXB for Xembly to ease your coding pain and streamline XML manipulation in your Java projects.
What is Xembly?
Xembly is a concise and powerful XML manipulation language that allows you to write XML transformation commands in a clear and straightforward manner. It provides a more intuitive and compact way to manipulate XML compared to traditional approaches like JAXB.
Why Swap JAXB for Xembly?
Concise and Readable Code
One of the key advantages of using Xembly is its concise and readable syntax. Let’s compare a simple XML manipulation task using JAXB and Xembly to understand the difference.
Consider the task of adding a new <book>
element to an existing XML document.
With JAXB:
// JAXB code for adding a new book element
JAXBContext jaxbContext = JAXBContext.newInstance(Bookstore.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
Bookstore bookstore = (Bookstore) unmarshaller.unmarshal(new File("books.xml"));
List<Book> books = bookstore.getBook();
Book newBook = new Book();
newBook.setTitle("New Book Title");
newBook.setAuthor("Author Name");
books.add(newBook);
Marshaller marshaller = jaxbContext.createMarshaller();
marshaller.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, true);
marshaller.marshal(bookstore, System.out);
Now, let’s achieve the same using Xembly:
// Xembly code for adding a new book element
String modifiedXml = new Xembler(
new Directives()
.xpath("/bookstore")
.add("book")
.attr("category", "New Category")
.append("title", "New Book Title")
.append("author", "Author Name")
).xml();
System.out.println(modifiedXml);
It's evident that the Xembly version is much more concise and readable, making the code easier to understand and maintain.
Flexibility and Agility
Xembly offers greater flexibility and agility when it comes to XML manipulation. Since Xembly commands are written as a sequence of directives, you can easily modify, add, or remove elements and attributes with simple, explicit instructions, without being constrained by the complexities of JAXB binding and unbinding.
No Additional Classes or Annotations
Unlike JAXB, Xembly does not require you to create additional Java classes or annotate your existing classes for XML binding. This reduces the overhead of managing JAXB annotations and enables you to focus solely on the XML manipulation logic.
Getting Started with Xembly
Now that we've highlighted the benefits of using Xembly for XML manipulation, let's delve into how you can get started with Xembly in your Java projects.
Adding Xembly Dependency
To use Xembly in your project, you need to add the Xembly dependency to your Maven or Gradle configuration.
For Maven, include the following dependency in your pom.xml
:
<dependency>
<groupId>org.xembly</groupId>
<artifactId>xembly</artifactId>
<version>0.31.8</version>
</dependency>
For Gradle, add the following dependency to your build.gradle
file:
implementation 'org.xembly:xembly:0.31.8'
Writing Xembly Commands
Once you've added the Xembly dependency, you can start writing Xembly commands to manipulate XML in your Java code.
Here's a simple example of how you can use Xembly to modify an existing XML document:
import org.xembly.Directives;
import org.xembly.Xembler;
public class XmlManipulationExample {
public static void main(String[] args) {
String originalXml = "<bookstore><book><title>Old Title</title></book></bookstore>";
String modifiedXml = new Xembler(
new Directives()
.xpath("/bookstore/book")
.remove()
.add("book")
.append("title", "New Title")
).apply(originalXml);
System.out.println(modifiedXml);
}
}
In this example, we apply a sequence of Xembly directives to the original XML, which results in the modification of the <title>
element within the <book>
element.
Advantages of Xembly Over JAXB
Improved Performance
Xembly typically offers improved performance compared to JAXB, especially for operations involving complex XML manipulation. Since Xembly commands are executed directly on the XML string, there's no overhead of marshalling, unmarshalling, or object instantiation, resulting in faster execution.
Enhanced Control Over XML Structure
With Xembly, you have fine-grained control over the XML structure, allowing you to perform specific and precise manipulations without the need to model the entire XML structure in Java classes, as required by JAXB.
Easier Maintenance and Refactoring
Xembly promotes easier maintenance and refactoring of XML manipulation logic. Its clear and concise syntax makes it simpler to identify and modify XML manipulation commands, leading to improved code maintainability and agility.
The Last Word
In conclusion, swapping JAXB for Xembly can significantly simplify XML manipulation in your Java projects. The concise syntax, flexibility, and agility offered by Xembly make it a compelling choice for handling XML data without the complexities of JAXB.
By leveraging Xembly, you can streamline your XML manipulation code, improve performance, and enhance the maintainability of your Java applications.
So, the next time you find yourself grappling with XML manipulation using JAXB, consider giving Xembly a try for a smoother and more efficient coding experience.
Explore Xembly and witness the power of streamlined XML manipulation in Java!
To learn more about Xembly, check out the official documentation.
Experience the ease of Xembly and revolutionize your XML handling in Java!