Efficiency vs. Performance: Beyond Just Big O Notation
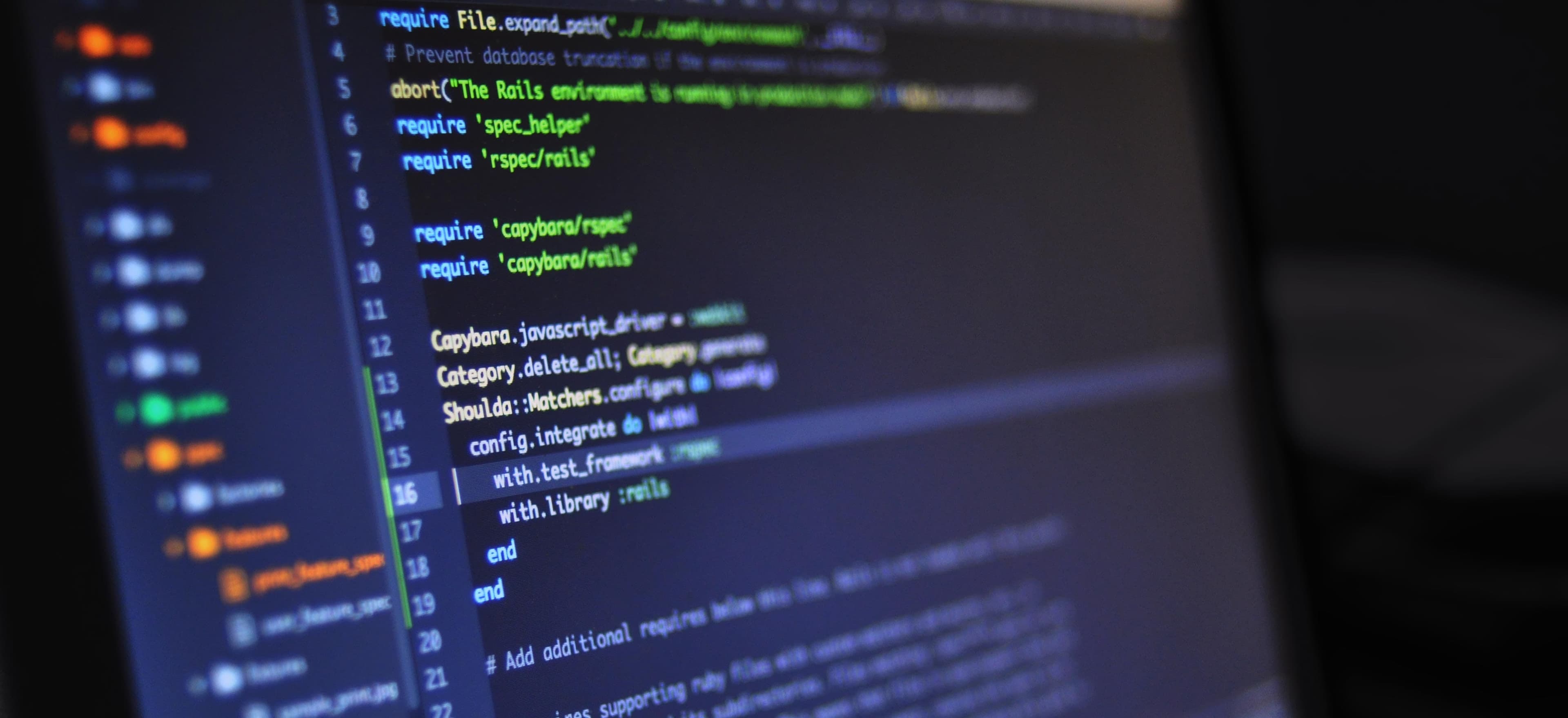
- Published on
When it comes to evaluating the performance of algorithms and data structures, Big O notation often takes the spotlight. While Big O notation provides a useful framework for comparing the efficiency of algorithms, it is crucial to understand that efficiency and performance are multidimensional concepts that cannot be fully captured by just looking at the asymptotic behavior of an algorithm.
In the world of Java programming, developers often aim to write code that is not only correct but also efficient and performant. In this blog post, we'll explore the nuances of efficiency and performance in Java, delving into aspects beyond Big O notation and discussing practical strategies for writing high-performance Java code.
Understanding Efficiency and Performance
Efficiency in the context of software typically refers to how well an algorithm or data structure utilizes computational resources such as time, memory, and other system resources. On the other hand, performance encompasses the broader spectrum of how well a piece of software meets its functional and non-functional requirements, including responsiveness, throughput, scalability, and resource utilization.
In Java, achieving high efficiency and performance involves leveraging the language features, libraries, and best practices to write code that not only executes quickly but also minimizes resource consumption and facilitates system scalability.
The Role of Data Structures and Algorithms
While Big O notation provides valuable insights into the asymptotic behavior of algorithms, it is essential to consider the practical implications of data structures and algorithm choices in the context of real-world Java applications.
For example, when working with collections in Java, the choice of the appropriate data structure can significantly impact the efficiency of operations such as insertions, deletions, and lookups. Understanding the underlying implementation and performance characteristics of data structures such as ArrayList, LinkedList, HashMap, and TreeMap is crucial for making informed decisions in Java programming.
Let's consider a practical scenario where the choice of data structure can make a notable difference in performance. Suppose we need to store a large collection of key-value pairs and frequently perform lookup operations based on the keys. In such a case, opting for a HashMap over an ArrayList can offer constant-time (O(1)) lookups on average, compared to linear-time (O(n)) lookups in an ArrayList.
// Using HashMap for efficient key-value lookups
Map<String, Integer> hashMap = new HashMap<>();
hashMap.put("apple", 10);
hashMap.put("orange", 20);
int orangeCount = hashMap.get("orange");
System.out.println("Count of oranges: " + orangeCount);
In this example, the HashMap provides a more efficient solution for the lookup operation compared to using an ArrayList.
Beyond Big O: Real-world Considerations
While Big O notation serves as a foundational concept for analyzing algorithmic complexity, it's essential to complement theoretical analysis with real-world considerations such as input size, constant factors, and practical constraints imposed by hardware and software environments.
In Java programming, the efficiency of algorithms can be influenced by factors such as memory allocation, garbage collection, I/O operations, and concurrency. Optimizing for Java-specific considerations, such as minimizing object creation to reduce the impact of garbage collection or leveraging parallel streams for multi-core processors, can significantly enhance the performance of Java applications.
// Example of leveraging parallel streams for concurrent processing
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sum = numbers.parallelStream().mapToInt(Integer::intValue).sum();
System.out.println("Sum of numbers: " + sum);
In this example, by using parallel streams, the computation of the sum can be efficiently parallelized to leverage the available processing power, resulting in improved overall performance.
Profiling and Benchmarking in Java
To truly understand and optimize the efficiency and performance of Java applications, developers often resort to profiling and benchmarking tools that provide insights into resource utilization, execution times, and potential bottlenecks.
Tools such as VisualVM, YourKit, and JMH (Java Microbenchmarking Harness) enable developers to identify performance hotspots, analyze memory usage, and compare the performance of alternative implementations. By utilizing these tools, developers can make informed decisions about optimizations and trade-offs, ensuring that their Java code is not only correct but also highly efficient.
A Final Look
In the realm of Java programming, achieving optimal efficiency and performance involves a multifaceted approach that goes beyond just analyzing algorithms through the lens of Big O notation. By understanding the practical implications of data structures, leveraging Java-specific optimizations, and embracing profiling and benchmarking techniques, developers can craft high-performance Java applications that excel in both efficiency and responsiveness.
As Java continues to evolve with advancements in language features, runtime optimizations, and hardware capabilities, the pursuit of efficiency and performance remains an ever-evolving journey for Java developers, demanding a keen understanding of the underlying principles and a commitment to continual improvement.
In conclusion, mastering efficiency and performance in Java entails a deep understanding of the language's ecosystem and a proactive approach to optimization, ultimately paving the way for the development of robust and high-performing Java applications.
Checkout our other articles