Eclipse & Maven: Solving Java 9 Module Headaches
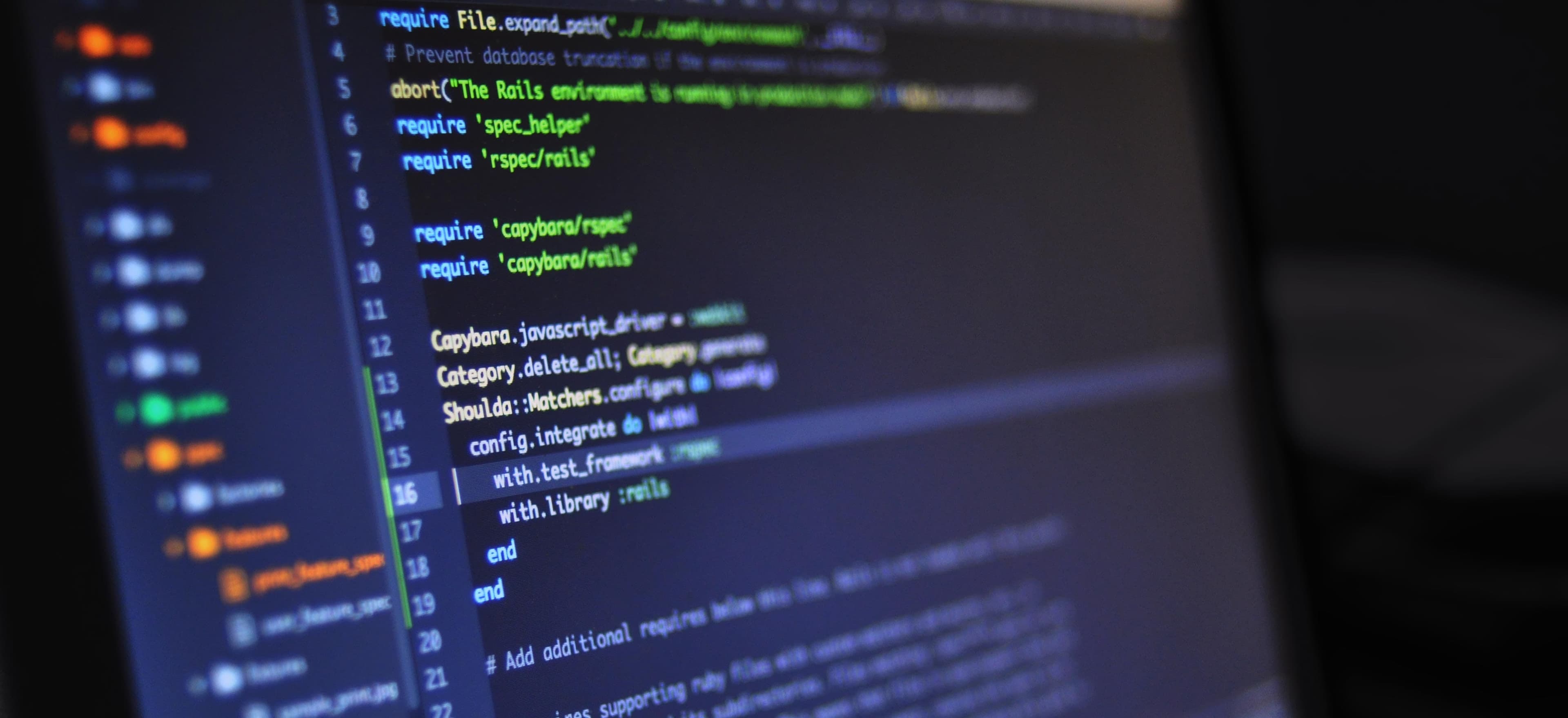
- Published on
Solving Java 9 Module Headaches with Eclipse and Maven
Java 9 introduced a significant change in the way developers work with modules. While modules provide a more organized and secure structure for Java applications, they also introduced complexities for developers, particularly with existing projects. In this post, we will explore how to use Eclipse and Maven to ease the integration of Java 9 modules into your projects.
The Java 9 Module Challenge
With the introduction of modules in Java 9, developers are now required to define module-info.java file to explicitly specify dependencies and exports. While this offers better encapsulation and organization, it also means existing projects that were not originally developed as modules need to be refactored.
Using Maven to Manage Java 9 Modules
Maven, the widely used build automation tool, can be leveraged to manage dependencies and builds for Java 9 modules seamlessly. Let's look at some essential configurations and plugins that can help in this process:
Maven Compiler Plugin
The Maven Compiler Plugin can be configured to use Java 9 as the target runtime. This ensures that the project is compiled in a way that is compatible with Java 9 modules.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.0</version>
<configuration>
<source>9</source>
<target>9</target>
</configuration>
</plugin>
</plugins>
</build>
In this snippet, we set the <source>
and <target>
versions to 9, indicating that the project is compatible with Java 9.
Maven Javadoc Plugin
The Maven Javadoc Plugin can be configured to handle Java 9 module documentation generation. By setting the release
parameter, the plugin ensures that the generated documentation complies with the module structure.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-javadoc-plugin</artifactId>
<version>3.1.1</version>
<configuration>
<release>9</release>
</configuration>
</plugin>
By configuring the Maven Javadoc Plugin in this way, the documentation generation process aligns with the Java 9 module system.
Maven Surefire Plugin
The Maven Surefire Plugin, used for running tests, can be configured to accommodate Java 9 and its module system.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M5</version>
<configuration>
<argLine>
--add-opens java.base/java.lang=ALL-UNNAMED
</argLine>
</configuration>
</plugin>
By adding the --add-opens
option within the <argLine>
configuration, the plugin enables proper test execution within a Java 9 module environment.
Eclipse Support for Java 9 Modules
Eclipse, one of the most popular integrated development environments (IDEs) for Java, provides strong support for Java 9 modules through its Java Development Tools (JDT) plugin. Here's how you can leverage Eclipse to work with Java 9 modules seamlessly:
Using Project Jigsaw
Eclipse Oxygen and further versions have built-in support for the Java 9 module system, known as Project Jigsaw. This means that when you create a new Java project in Eclipse with a Java runtime environment (JRE) set to Java 9 or above, it handles the module-info.java file automatically.
Module Path Configuration
Eclipse allows you to configure module paths for your projects, making it easy to manage module dependencies within your workspace. This ensures that modules are resolved correctly during compilation and execution.
Module Graph Visualization
Eclipse provides a visual representation of the module graph, making it easier to understand the dependencies between modules in your project. This can be incredibly useful when working with complex modular systems.
Integrating Maven and Eclipse for Java 9 Modules
To effectively leverage the power of Maven and Eclipse for Java 9 modules, seamless integration between these two tools is essential. Here are some tips to ensure smooth collaboration between Maven and Eclipse for Java 9 module development:
Maven Project Configuration
When creating a new Maven project for Java 9 modules, ensure that the project's POM file includes the necessary configurations for Java 9 compatibility and module support, as outlined earlier in this post.
Eclipse Project Setup
When importing a Maven project into Eclipse, ensure that the project's Java Build Path and Module Path in Eclipse reflect the module configurations defined in the project's POM file. This alignment ensures that Eclipse understands the module structure and dependencies as specified in the Maven project.
Maven-Eclipse Integration Plugins
Consider using Maven integration plugins for Eclipse, such as "m2e" (Maven Integration for Eclipse). These plugins ensure that changes made to the Maven project configuration are seamlessly propagated to the Eclipse environment, maintaining consistency between the two.
The Bottom Line
In conclusion, Java 9 modules bring a powerful new way of organizing and structuring Java projects, but they also introduce complexities, especially for existing projects. By leveraging Maven for managing dependencies and builds, and integrating it with the robust support for Java 9 modules in Eclipse, developers can effectively tackle the transition to modular development in Java.
With the essential Maven configurations and Eclipse features discussed in this post, developers can navigate the challenges of Java 9 modules with confidence, ensuring a smooth transition and continued productivity in their Java projects. Embracing the modularity provided by Java 9 leads to more maintainable, scalable, and secure applications, making the effort well worth it.
Do you plan to migrate your Java projects to Java 9 modules? Share your thoughts and experiences in the comments below!
Checkout our other articles