Decoding JavaEE: Making Sense of Its Complex Concepts
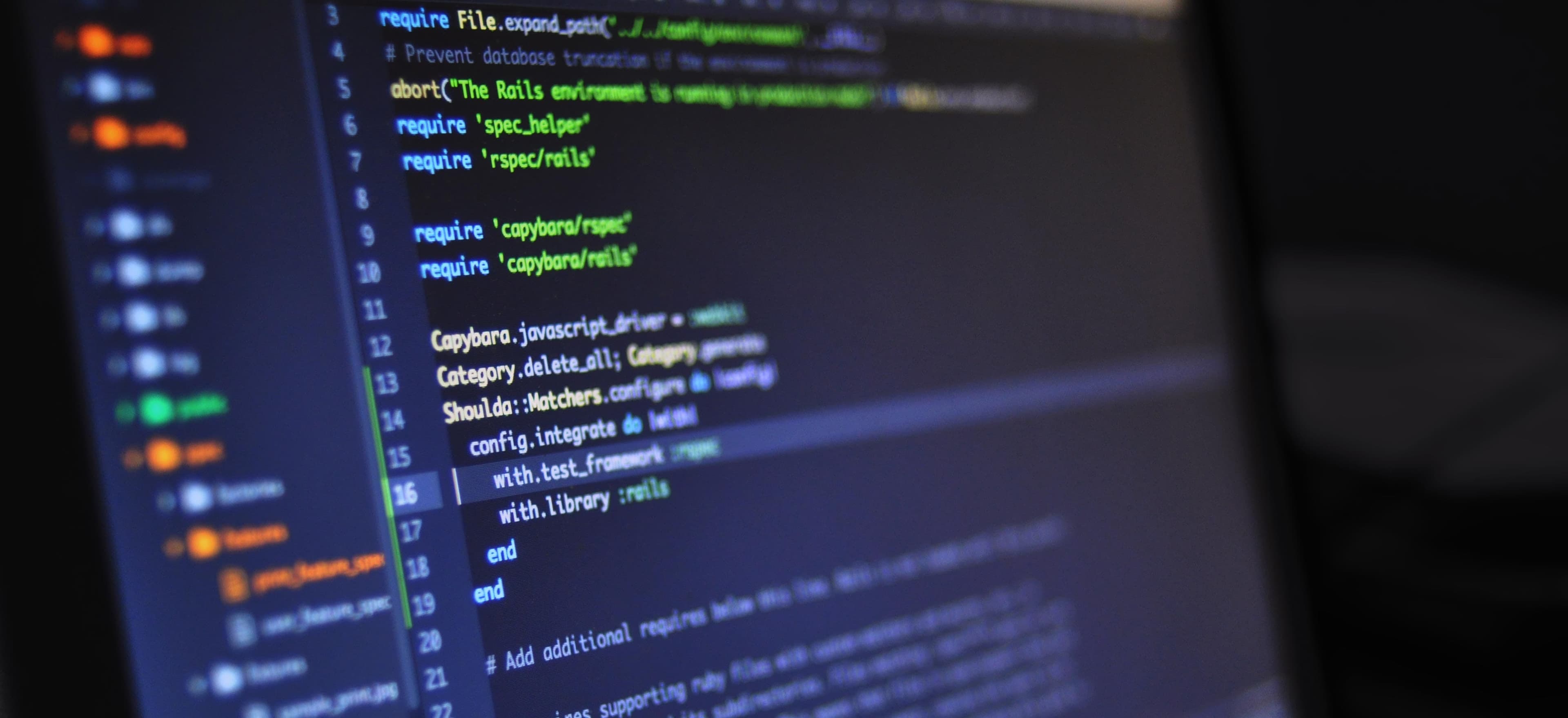
- Published on
Decoding JavaEE: Making Sense of Its Complex Concepts
Java Enterprise Edition (JavaEE) is a powerful platform for building enterprise-level applications. However, its vast array of features and concepts can be daunting for developers new to the platform. In this post, we'll delve into some of the key concepts of JavaEE, unraveling its complexities and shedding light on its inner workings.
What is JavaEE?
JavaEE, now known as Jakarta EE, is a set of specifications that extend the Java SE platform to provide enterprise features such as distributed computing and web services. It encompasses a wide range of APIs and technologies that facilitate the development of large-scale, multi-tiered, scalable, reliable, and secure network applications.
The Anatomy of a JavaEE Application
At the heart of JavaEE is the concept of a JavaEE application, which consists of components such as Enterprise JavaBeans (EJB), Java Persistence API (JPA) entities, servlets, and more. These components are assembled into modules and deployed to application servers, where they are executed and managed.
Enterprise JavaBeans (EJB)
Enterprise JavaBeans (EJB) is a key component of JavaEE for building scalable, distributed, and transactional business logic. There are three types of EJBs: session beans, entity beans, and message-driven beans, each serving different purposes in the enterprise application architecture.
Here's an example of a simple stateless session bean:
@Stateless
public class ShoppingCartBean {
// Business logic methods
}
In this example, the @Stateless
annotation indicates that this EJB is a stateless session bean, which is suitable for most business logic implementations.
Java Persistence API (JPA)
The Java Persistence API (JPA) is the standard way of persisting Java objects into a relational database. It provides an object-relational mapping (ORM) framework that allows developers to interact with databases using plain old Java objects (POJOs).
An example JPA entity class looks like this:
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private BigDecimal price;
// Getters and setters
}
In this example, the @Entity
annotation marks this class as a JPA entity, and the @Id
and @GeneratedValue
annotations specify the primary key generation strategy.
Dependency Injection
JavaEE applications heavily rely on the concept of dependency injection to achieve loose coupling and enhance testability. Dependency injection allows components to be loosely coupled and easily testable by injecting the required dependencies at runtime, usually performed by the application server or a dedicated framework like CDI (Contexts and Dependency Injection).
Here's an example of dependency injection using CDI:
@Stateless
public class ShoppingCartBean {
@Inject
private ProductService productService;
// Business logic methods
}
In this example, the @Inject
annotation marks the field productService
for dependency injection, allowing the ShoppingCartBean
to use the ProductService
without explicitly instantiating it.
Aspect-Oriented Programming (AOP)
Aspect-Oriented Programming (AOP) is a powerful paradigm used in JavaEE for separating cross-cutting concerns from the core business logic. AOP allows developers to encapsulate behaviors that cut across multiple components into reusable modules called aspects, reducing code duplication and enhancing maintainability.
An example of using AOP with CDI interceptor:
@Interceptor
@Loggable
public class LoggingInterceptor {
@AroundInvoke
public Object logMethodCall(InvocationContext context) throws Exception {
// Log method call
return context.proceed();
}
}
In this example, the @Interceptor
annotation declares this class as an interceptor, and the @Loggable
annotation specifies the pointcut at which this interceptor should be applied.
Microservices and JavaEE
The rise of microservices architecture has revolutionized the way enterprise applications are designed and developed. Although JavaEE was traditionally associated with monolithic applications, its latest incarnation as Jakarta EE has adapted to the microservices trend by embracing lightweight, cloud-native architectures, and providing support for technologies such as Docker and Kubernetes.
The Closing Argument
JavaEE, now Jakarta EE, remains an indispensable platform for building enterprise applications, despite the evolving landscape of modern application development. By understanding its key concepts such as Enterprise JavaBeans, Java Persistence API, dependency injection, and aspect-oriented programming, developers can harness the full potential of JavaEE and build scalable, maintainable, and robust enterprise applications.
In future posts, we will delve deeper into each of these concepts, exploring best practices, common pitfalls, and advanced techniques to empower JavaEE developers in their journey towards mastering this powerful platform.
Stay tuned for more insights into the world of Jakarta EE!