Balancing Acts: Unveiling CAP Theorem's Critical Trade-offs
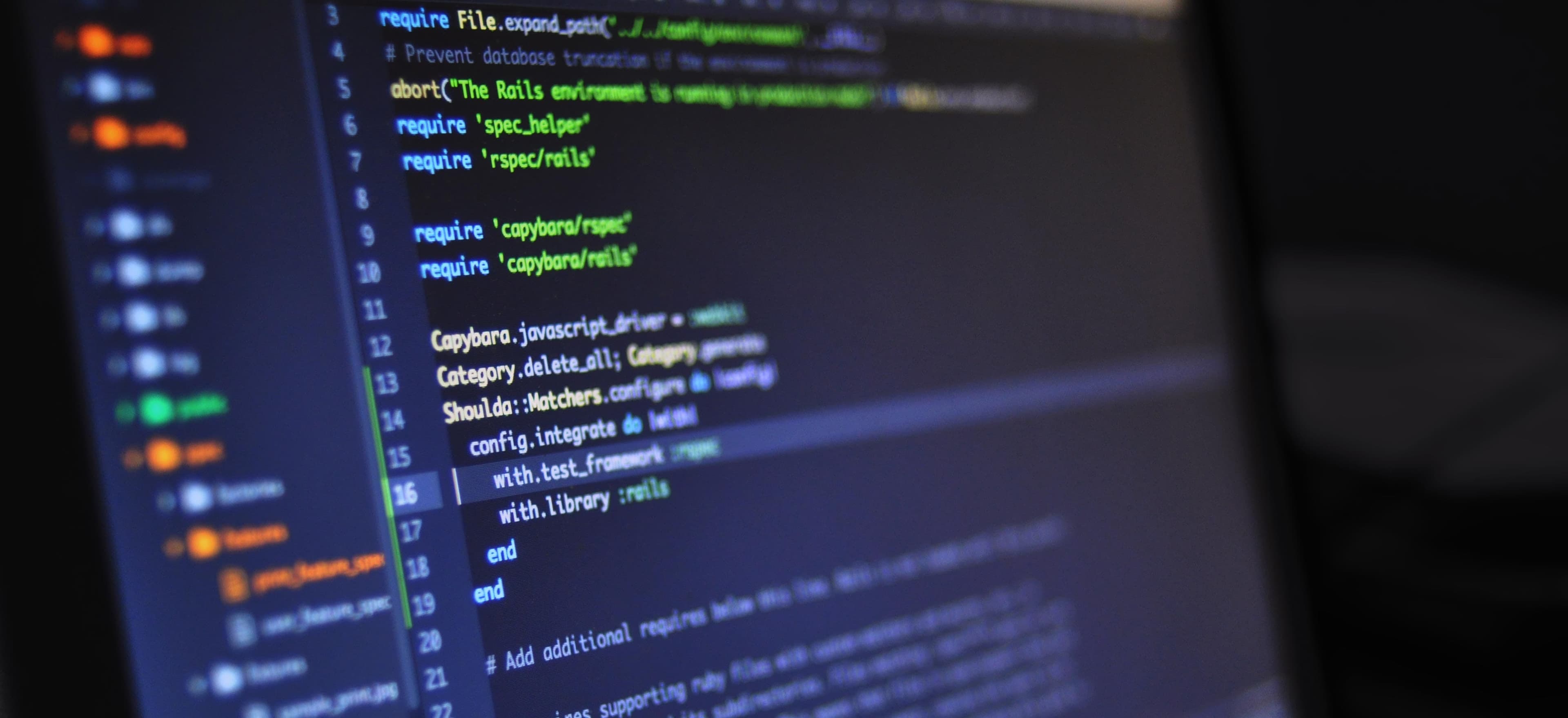
- Published on
Balancing Acts: Unveiling CAP Theorem's Critical Trade-offs
In the realm of distributed systems, where reliability and scalability are paramount, engineers often encounter the CAP theorem. Coined by computer scientist Eric Brewer, the CAP theorem asserts that it's impossible for a distributed data store to simultaneously provide more than two out of the following three guarantees: consistency, availability, and partition tolerance.
Understanding the trade-offs entailed by the CAP theorem is pivotal for architects and developers. In this blog post, we'll delve into the intricacies of the CAP theorem, dissect its critical trade-offs, and explore how Java helps navigate these challenges.
Demystifying the CAP Theorem
Consistency
Consistency in the context of the CAP theorem signifies that all nodes in a distributed system possess the same data at the same time. When a new piece of data is entered into the system, all subsequent retrievals of that data will yield the latest value.
Availability
Availability dictates that every request for data in a distributed system receives a response, regardless of whether a node is experiencing a failure or delay. In other words, the system remains operational even under adverse conditions.
Partition Tolerance
Partition tolerance refers to the system's ability to sustain network partitions—i.e., communication breakdowns among nodes—without compromising its functionality. This aspect is particularly significant in large-scale distributed systems susceptible to network issues.
Understanding the Trade-offs
The essence of the CAP theorem lies in the trade-offs between consistency, availability, and partition tolerance. Embracing all three aspects simultaneously is unfeasible; hence, architects and developers encounter the dilemma of prioritizing two and making concessions on the third. Let's dissect the trade-offs:
- CP Systems: Prioritize consistency and partition tolerance, sacrificing availability in the event of network partitioning. These systems ensure data uniformity across all nodes but risk momentary unavailability.
- AP Systems: Emphasize availability and partition tolerance while allowing for eventual consistency. In this scenario, the system remains functional despite network partitioning, but data may not be immediately consistent across all nodes.
- CA Systems: Strive for consistency and availability, disregarding partition tolerance. These systems prioritize data consistency and continuous availability, but are susceptible to disruptions in case of network partitioning.
Navigating Trade-offs with Java
Java, with its robust ecosystem and versatile features, equips developers to navigate the intricate trade-offs posed by the CAP theorem. Let's explore key strategies and tools in the Java landscape that aid in striking the right balance.
Asynchronous Communication
In distributed systems, asynchrony plays a pivotal role in achieving partition tolerance and availability. Java's extensive support for asynchronous programming, exemplified by CompletableFuture and reactive libraries like Project Reactor, empowers developers to design resilient systems capable of withstanding network partitions and sustaining responsiveness.
CompletableFuture.supplyAsync(() -> fetchDataFromRemoteService())
.thenApply(data -> processAndReturnResult(data))
.exceptionally(ex -> handleException(ex));
In this code snippet, CompletableFuture facilitates asynchronous execution, essential for managing network partitioning and ensuring system availability.
Consistency Models
Java's support for various consistency models, such as strong consistency through distributed transactions and eventual consistency via asynchronous replication, enables developers to tailor their approach based on specific application requirements. Leveraging Java Transaction API (JTA) for distributed transactions and frameworks like Apache ZooKeeper for maintaining consensus, developers can navigate the trade-offs with precision.
try (Transaction tx = TM.begin()) {
// Perform operations across distributed resources
TM.commit(tx);
} catch (Exception e) {
// Handle transactional failures
TM.rollback(tx);
}
The code snippet showcases Java Transaction API usage for ensuring strong consistency across distributed resources, offering a meticulous approach to trade-offs.
Fault-tolerant Design Patterns
Java's support for fault-tolerant design patterns, such as Circuit Breaker and Retry, provides developers with tools to fortify systems against transient faults, thereby enhancing availability. By incorporating resilience patterns from libraries like Hystrix and resilience4j, Java empowers developers to navigate the trade-offs without compromising on system robustness.
CircuitBreaker circuitBreaker = CircuitBreaker.of("backendService", circuitBreakerConfig);
Supplier<String> supplier = () -> backendService.getData();
String result = circuitBreaker.executeSupplier(supplier);
The above code snippet exemplifies the utilization of a Circuit Breaker pattern in Java, showcasing its role in safeguarding availability amidst transient faults.
Closing Remarks
As distributed systems continue to shape modern technological landscapes, confronting the trade-offs encapsulated by the CAP theorem remains inevitable. However, with Java's versatile toolkit encompassing asynchronous communication, consistency models, and fault-tolerant design patterns, engineers can adeptly navigate these trade-offs without compromising on the system's reliability and scalability.
By comprehending the underlying principles of the CAP theorem and harnessing Java's capabilities, engineers are equipped to orchestrate distributed systems that strike a harmonious balance between consistency, availability, and partition tolerance—a feat essential for thriving in an era dominated by distributed computing.
In the complex interplay of distributed systems and the CAP theorem, Java stands as a stalwart ally, enabling engineers to navigate the intricate trade-offs with poise and precision.
In the grand tapestry of distributed systems, Java emerges as a guiding force, empowering engineers to harmonize the intricate trade-offs embedded in the CAP theorem with dexterity and finesse.