Solving the Mystery: Java 8 Default Methods Explained
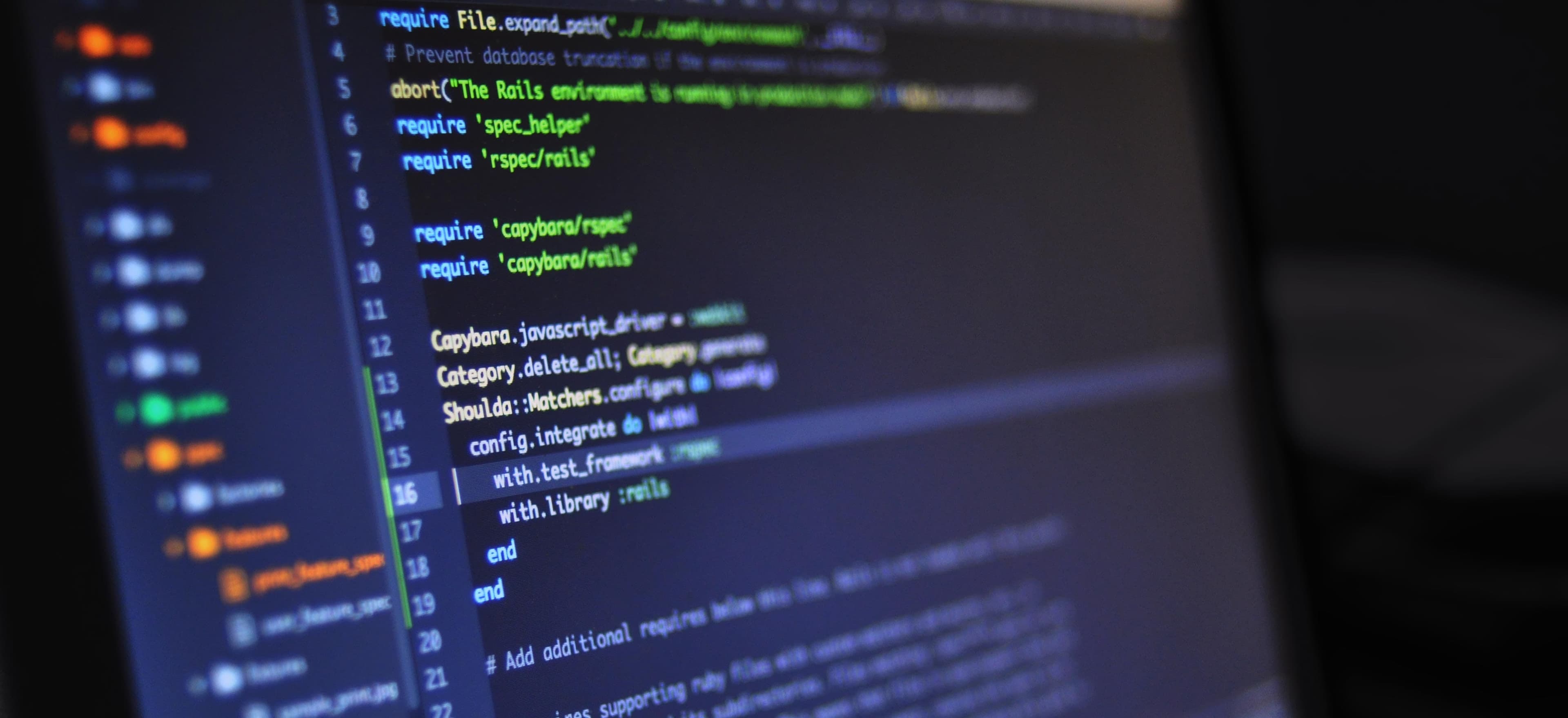
- Published on
Solving the Mystery: Java 8 Default Methods Explained
In the world of Java development, the release of Java 8 brought with it a plethora of new features and enhancements. Among these were default methods, which introduced a new concept to the language. In this article, we will unravel the mystery behind Java 8 default methods, exploring what they are, how they work, and when to use them in your code.
Understanding Default Methods
In prior versions of Java, interfaces could only declare method signatures, leaving implementing classes responsible for defining the method bodies. This proved to be quite restrictive, especially when attempting to evolve interfaces without breaking existing implementations.
Default methods were introduced in Java 8 to address this limitation. They allow interfaces to provide method implementations, thereby enabling the addition of new functionalities to interfaces without affecting the classes that implement them.
How Default Methods Work
Let's delve into the mechanics of default methods. Consider the following interface:
public interface Vehicle {
void start();
default void honk() {
System.out.println("Beep beep!");
}
}
In this example, the honk
method is a default method. If a class implements the Vehicle
interface but does not provide its own implementation of the honk
method, it will inherit the default implementation provided in the interface. This ensures that existing classes implementing the Vehicle
interface will not be impacted by the addition of the honk
method.
When to Use Default Methods
Default methods are particularly useful when working with interfaces that need to evolve without breaking existing code. They provide a way to add new methods to interfaces that have been previously released without forcing implementing classes to immediately provide implementations for these new methods.
Additionally, default methods facilitate the introduction of new functionality in library interfaces without disrupting existing clients of those interfaces.
Pros and Cons of Default Methods
Pros
- Evolution of Interfaces: Default methods allow interfaces to evolve by adding new methods without breaking existing implementations.
- Backward Compatibility: Existing classes that implement interfaces with default methods are not required to provide implementations for the newly added methods.
Cons
- Potential for Ambiguity: If a class implements multiple interfaces, and those interfaces contain default methods with the same signature, ambiguity can arise. However, this can be resolved by explicitly overriding the default method in the implementing class.
Example Use Case: Default Methods in Collections
A classic example of default methods in action can be found in the Java Collections framework introduced in Java 8. The Collection
interface now includes several default methods for performing common operations on collections, such as forEach
, removeIf
, and spliterator
.
public interface Collection<E> extends Iterable<E> {
// Other method declarations...
default void forEach(Consumer<? super E> action) {
for (E e : this) {
action.accept(e);
}
}
// Other default methods...
}
These default methods allow the Collections API to provide enhanced functionality while maintaining compatibility with existing code that relies on the Collection
interface.
Best Practices for Using Default Methods
While default methods offer powerful capabilities, they should be used judiciously and with careful consideration. Here are some best practices for using default methods in your Java code:
- Prefer Interfaces: Where possible, prefer the use of interfaces with default methods over abstract classes. This promotes flexibility and reusability, especially when dealing with multiple inheritance scenarios.
- Avoid Overuse: Default methods should not be used as a substitute for proper interface design. They are best suited for adding new methods to existing interfaces or enhancing library interfaces, rather than as a primary means of designing interfaces.
- Document Intended Behavior: When designing default methods, clearly document the intended behavior and usage guidelines to help developers understand when and how to use them effectively.
Lessons Learned
In conclusion, Java 8 default methods provide a means to enhance the capabilities of interfaces in a backward-compatible manner. By allowing interfaces to provide method implementations, default methods enable the evolution of interfaces without disrupting existing implementations. When used judiciously and in accordance with best practices, default methods can greatly enhance the flexibility and extensibility of your Java code.
Default methods in Java 8 offer a compelling solution to the long-standing challenge of evolving interfaces while maintaining backward compatibility, and embracing them as part of your Java development arsenal can lead to more robust and adaptable code bases.
To delve even deeper into Java 8 default methods, head over to the Java official documentation.
Did this article help demystify Java 8 default methods for you? Let me know in the comments!