Troubleshooting: Accessing Process ID in JDK 10
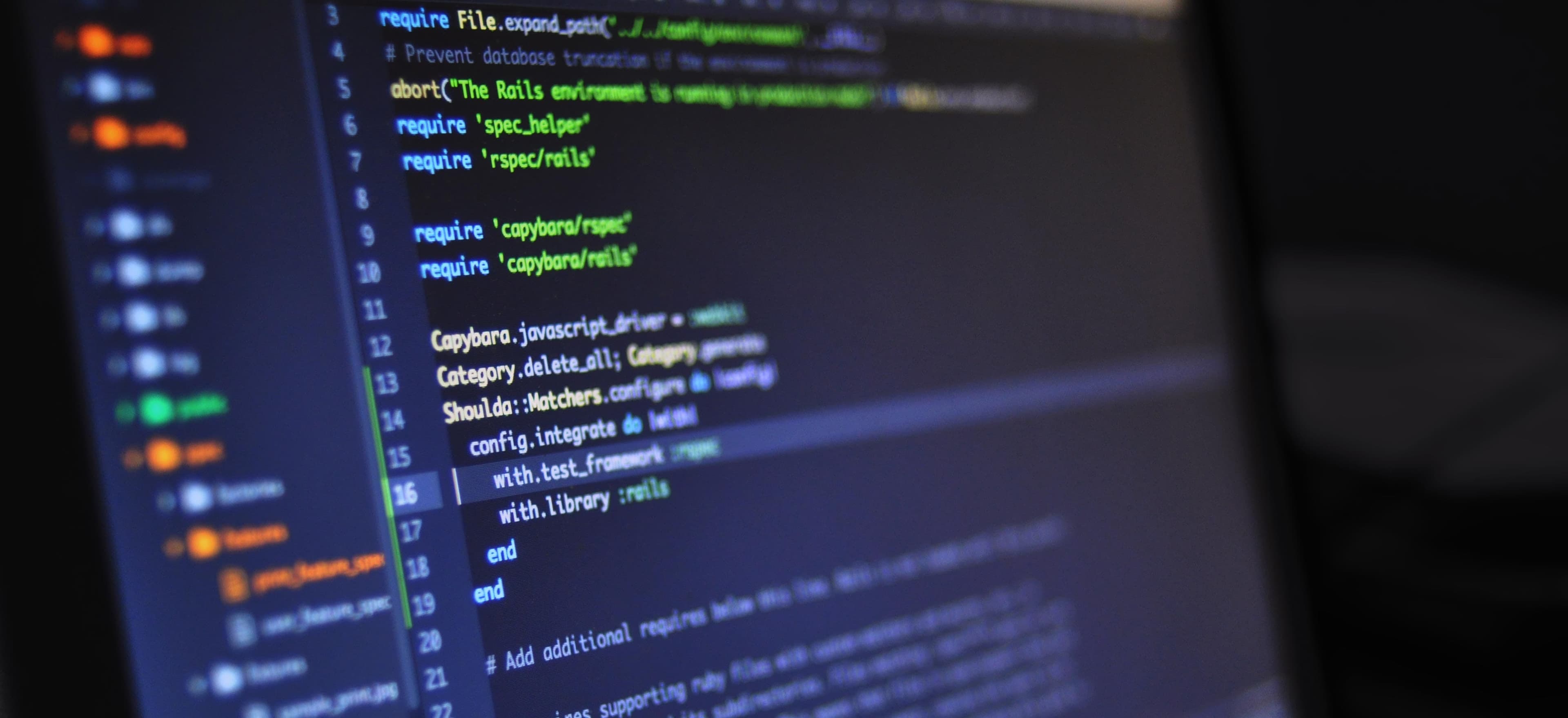
- Published on
Troubleshooting: Accessing Process ID in JDK 10
Java 10 introduces a new feature that allows easy access to the process ID of the current running Java application. This enhancement provides a more straightforward way to obtain the process ID without relying on native code or external libraries. In this article, we will explore how to leverage this feature and address common issues related to process ID retrieval in JDK 10.
Using ProcessHandle
The ProcessHandle
class in Java 9 and later versions provides methods to manage and monitor processes. One of its useful functionalities is the ability to retrieve the process ID, which can be obtained by calling the pid()
method.
long processId = ProcessHandle.current().pid();
System.out.println("Process ID: " + processId);
In the above code snippet, ProcessHandle.current()
returns the current process handle, and pid()
retrieves the process ID associated with that process handle. This straightforward approach eliminates the need for platform-specific code or external dependencies to access the process ID.
Troubleshooting Common Issues
Compatibility with Earlier Java Versions
If your project needs to maintain compatibility with earlier versions of Java, such as JDK 8, where ProcessHandle
is not available, you can use libraries like JNA (Java Native Access) or JNR (Java Native Runtime) to access the process ID. Additionally, you can explore third-party libraries like SIGAR for this purpose.
It is essential to evaluate the trade-offs between using the native method and leveraging the built-in ProcessHandle
based on the project's specific requirements and compatibility constraints.
Security Manager Restrictions
In certain scenarios, security manager restrictions may prevent access to process IDs. It is crucial to ensure that the security policy grants the necessary permissions to retrieve process information. Granting RuntimePermission("getProcessId")
in the security policy file allows the code to access process IDs.
grant {
permission java.lang.RuntimePermission "getProcessId";
};
By explicitly defining the getProcessId
permission, the security manager permits the Java application to access process IDs without being restricted.
Handling Platform Variations
Although the ProcessHandle
provides a standardized way to retrieve the process ID, it is essential to consider platform variations. The behavior of process management can differ across operating systems, and certain platform-specific considerations may need to be addressed when working with process IDs.
Why Use the Built-In Process ID Access?
Utilizing the built-in process ID access in JDK 10 offers several advantages:
-
Cross-Platform Compatibility: The
ProcessHandle
class abstracts the process management operations and provides a consistent approach to access process IDs across different operating systems. -
Simplicity and Readability: By leveraging the
ProcessHandle
API, the code for accessing process IDs becomes more straightforward and readable as it avoids platform-specific conditional logic or external dependencies. -
Future Compatibility: As Java evolves, relying on built-in features ensures that the codebase aligns with the advancements in the language, minimizing the need for frequent updates related to process management.
In summary, the built-in process ID access in JDK 10 simplifies the retrieval of process IDs and enhances the overall robustness of Java applications.
Bringing It All Together
Accessing the process ID in JDK 10 is a significant improvement that provides a standardized and platform-independent approach to retrieve process IDs. By utilizing the ProcessHandle
class, Java developers can streamline process management operations and mitigate the complexities associated with platform-specific code or external libraries.
In this article, we discussed the usage of ProcessHandle
to access process IDs, troubleshoot common issues, and highlighted the benefits of leveraging the built-in feature. It is crucial to embrace these advancements in Java to enhance the efficiency and maintainability of applications.
For further insights into process management in Java and JDK 10 features, consider exploring the official Java documentation.
Stay tuned for more informative content on Java and its latest enhancements!