Java OutOfMemoryError: Ways to Prevent and Resolve
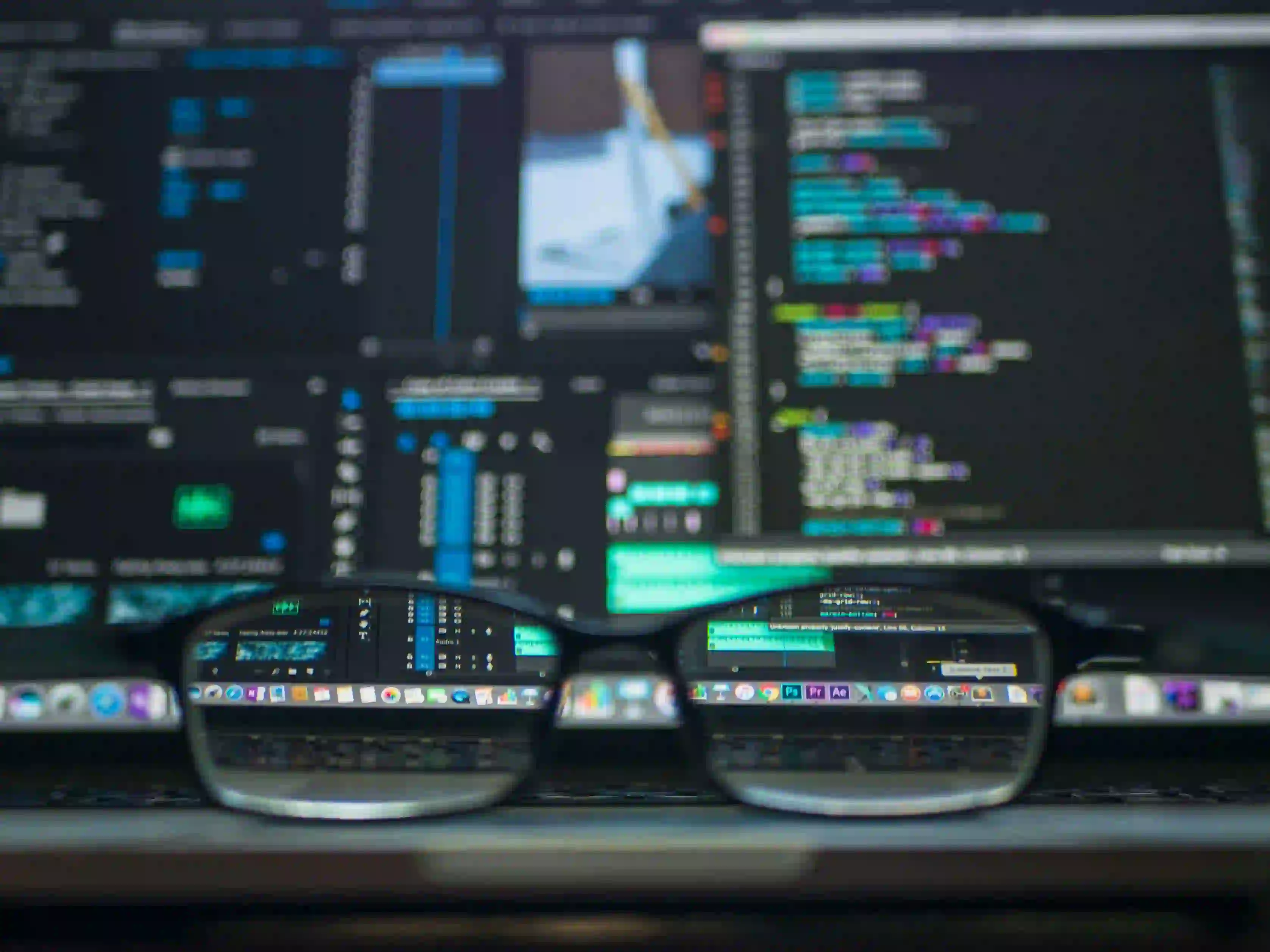
Preventing and Resolving OutOfMemoryError in Java
In the world of Java development, encountering an OutOfMemoryError
is a common occurrence. This error arises when the Java Virtual Machine (JVM) runs out of memory and is unable to allocate more memory for new objects. The good news is that this issue is entirely solvable through a combination of preventive measures and resolution strategies.
In this article, we will explore the common causes of OutOfMemoryError
and discuss effective preventive steps and resolutions to keep your Java application running smoothly.
Understanding the Causes
Before delving into preventive and resolution techniques, it's important to grasp the various factors that can lead to OutOfMemoryError
. Some common causes include:
-
Memory Leaks: When objects are no longer needed but are not released by the application, they accumulate in memory, eventually leading to an out-of-memory situation.
-
Insufficient Heap Space: If the allocated heap space is too small to accommodate the application's memory demands, an
OutOfMemoryError
can occur. -
High Object Creation Rate: Rapid creation of objects, especially large ones, can quickly deplete the available memory.
Preventive Measures
1. Efficient Memory Management
Example of efficient memory management:
public void processLargeData() {
try (InputStream is = new FileInputStream("largefile.txt");
BufferedReader br = new BufferedReader(new InputStreamReader(is))) {
// Process the data
} catch (IOException e) {
// Handle exception
}
}
In the above example, the try-with-resources statement ensures that the input streams are closed after being used, preventing memory leaks.
Efficient memory management entails properly releasing resources, using try-with-resources for automatic resource management, and employing tools like Apache Commons IO for stream manipulation to avoid memory leaks.
2. Monitoring and Profiling
Using tools like VisualVM, JConsole, or Java Mission Control to monitor memory usage and identify potential leaks can help in taking proactive measures to prevent OutOfMemoryError
.
3. JVM Heap Configuration
Adjusting the JVM heap size based on the application's memory requirements can prevent OutOfMemoryError
. This can be achieved using the -Xms
and -Xmx
parameters to set the initial and maximum heap size respectively.
Example of JVM heap size configuration:
java -Xms512m -Xmx1024m MyApplication
By configuring the initial heap size to 512 megabytes and the maximum heap size to 1024 megabytes, the JVM's memory management can be optimized to prevent out-of-memory situations.
Resolving OutOfMemoryError
1. Analyzing Heap Dumps
Heap dumps provide valuable insights into memory usage, object allocation, and potential leaks. Tools like Eclipse MAT (Memory Analyzer Tool) can be used to analyze heap dumps and identify memory-hungry objects or potential leaks.
2. Optimizing Data Structures
Using efficient data structures and algorithms can significantly reduce memory usage. For example, opting for HashMap
instead of Hashtable
can lead to better memory utilization.
3. Avoiding String Concatenation in Loops
Example of avoiding string concatenation in loops:
StringBuilder result = new StringBuilder();
for (int i = 0; i < 1000; i++) {
result.append("data" + i);
}
In the above code snippet, using StringBuilder
instead of string concatenation within the loop can prevent unnecessary object creation and reduce memory consumption.
4. Caching and Object Reuse
For frequent and costly operations, caching results and reusing objects can mitigate memory pressure. This approach minimizes the creation of new objects, thereby reducing the risk of running out of memory.
5. Tuning Garbage Collection
Fine-tuning garbage collection settings can optimize memory management and prevent OutOfMemoryError
. Parameters such as -XX:+UseConcMarkSweepGC
and -XX:NewRatio
can be adjusted based on the application's memory requirements.
Closing the Chapter
Preventing and resolving OutOfMemoryError
in Java necessitates a comprehensive approach encompassing efficient memory management, proactive monitoring, and strategic optimization. By adhering to the preventive measures and resolution strategies discussed in this article, developers can maintain the stability and performance of their Java applications, ensuring a seamless user experience.
Remember that addressing memory issues is vital for the long-term success of any Java application, and a proactive approach can save a lot of time and effort in the long run. By implementing these best practices, you can significantly reduce the occurrence of OutOfMemoryError
and ensure the smooth operation of your Java applications.