Preventing Null Pointer Exceptions in Java
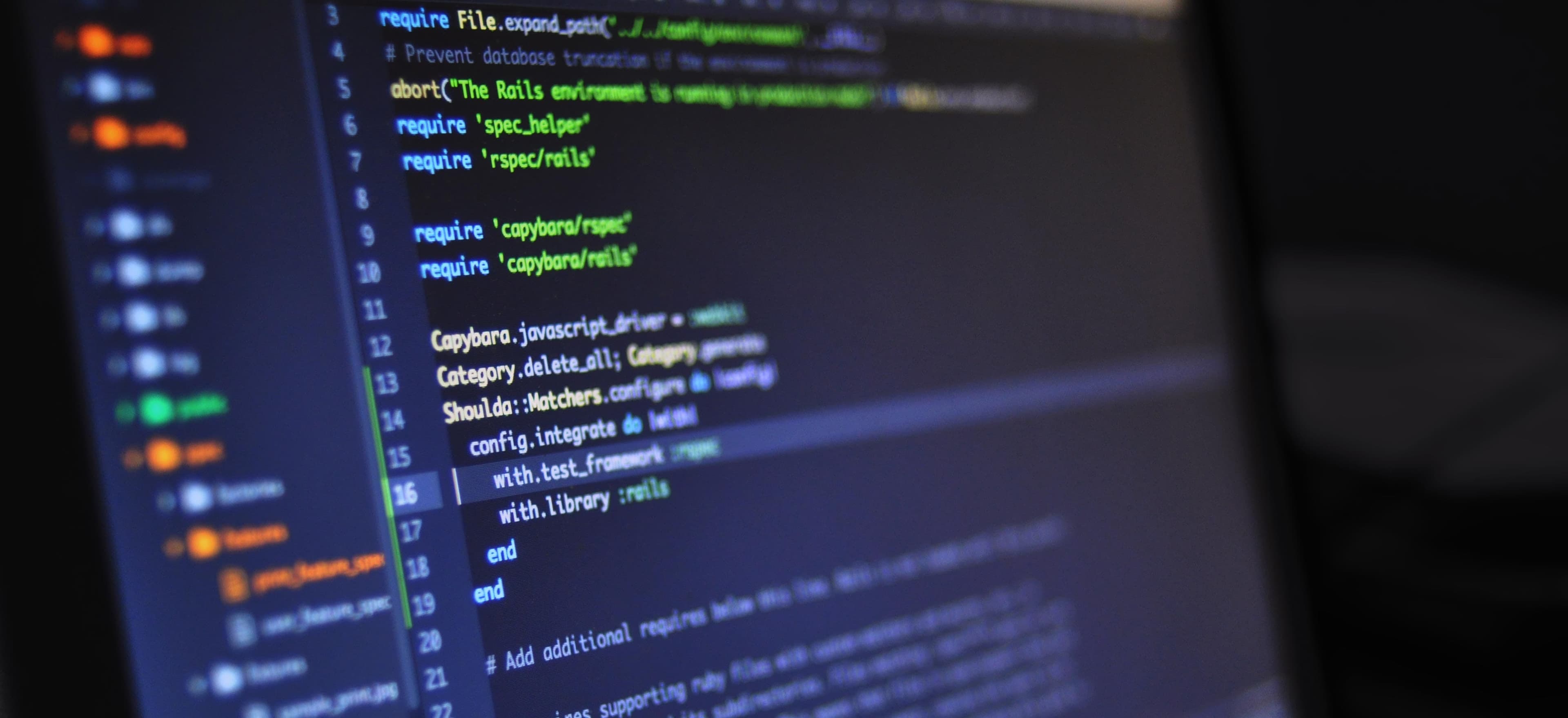
- Published on
Preventing Null Pointer Exceptions in Java
Null Pointer Exceptions (NPEs) are a common source of frustration for Java developers. They occur when a variable expected to hold an object reference instead holds null
, and an attempt is made to access an instance method or field on the variable. This leads to a runtime exception being thrown.
In this blog post, we will explore some best practices and techniques to prevent Null Pointer Exceptions in Java.
1. Use Optional to Avoid Null Checks
Java 8 introduced the Optional
class to represent optional values instead of null
references. By using Optional
, you can explicitly indicate whether a value is present or not, which in turn helps in avoiding NPEs.
Optional<String> optionalName = Optional.ofNullable(getName());
String name = optionalName.orElse("Default Name");
In the above example, getName()
may return null
, but by using Optional
, we can handle this case explicitly without encountering a NPE.
2. Leverage Objects.requireNonNull
The Objects
class in Java provides a useful method called requireNonNull
to check if an object reference is not null
. It throws a NullPointerException
if the given object reference is null
. This can be particularly useful in constructor parameter validation.
public class Person {
private String name;
public Person(String name) {
this.name = Objects.requireNonNull(name, "Name must not be null");
}
}
In the above example, the Person
constructor ensures that the name
parameter is not null
by using Objects.requireNonNull
.
3. Annotation-Based Null Checks
Frameworks like Hibernate and Spring offer annotations (@NotNull
, @NonNull
, etc.) that can be used for compile-time null checks. These annotations help in indicating the nullability of fields and method parameters, allowing for static analysis tools to catch potential NPEs during compilation.
public class User {
private String username;
public void setUsername(@NotNull String username) {
this.username = username;
}
}
By using @NotNull
annotation, we inform the compiler that the username
parameter must not be null
, thus preventing NPEs at runtime.
4. Defensive Coding
Defensive coding involves validating method arguments, return values, and intermediate results before using them to prevent unexpected null
references. It's a good practice to perform these checks in critical parts of your code.
public int calculateLength(String text) {
if (text != null) {
return text.length();
} else {
return 0;
}
}
In the above calculateLength
method, we defensively check if the text
parameter is null
before performing any operation on it. This simple practice can contribute significantly to preventing NPEs.
5. Stream Processing with Optional
When working with streams, using Optional
can be an effective way to handle potential null
elements in the stream and avoid NPEs.
List<String> names = Arrays.asList("Alice", "Bob", null, "Dave");
long validNamesCount = names.stream()
.filter(Objects::nonNull)
.map(String::toUpperCase)
.count();
In the above stream processing, Objects::nonNull
is used to filter out null
elements before performing any operations on the stream.
My Closing Thoughts on the Matter
Null Pointer Exceptions can be a headache for Java developers, but by following the best practices outlined in this post, you can reduce the occurrence of NPEs in your code. From leveraging Optional
to defensive coding, there are several techniques at your disposal to handle null
references more effectively.
Remember, the key to preventing NPEs is to always be mindful of the possibility of null
references and to proactively address them in your code.
By incorporating these techniques, you can write more robust and reliable Java code, minimizing the likelihood of encountering null-related issues.
For further reading on this topic, check out this comprehensive guide on Handling Nulls in Java.
Happy coding!