Optimizing Memory Usage in Java Applications
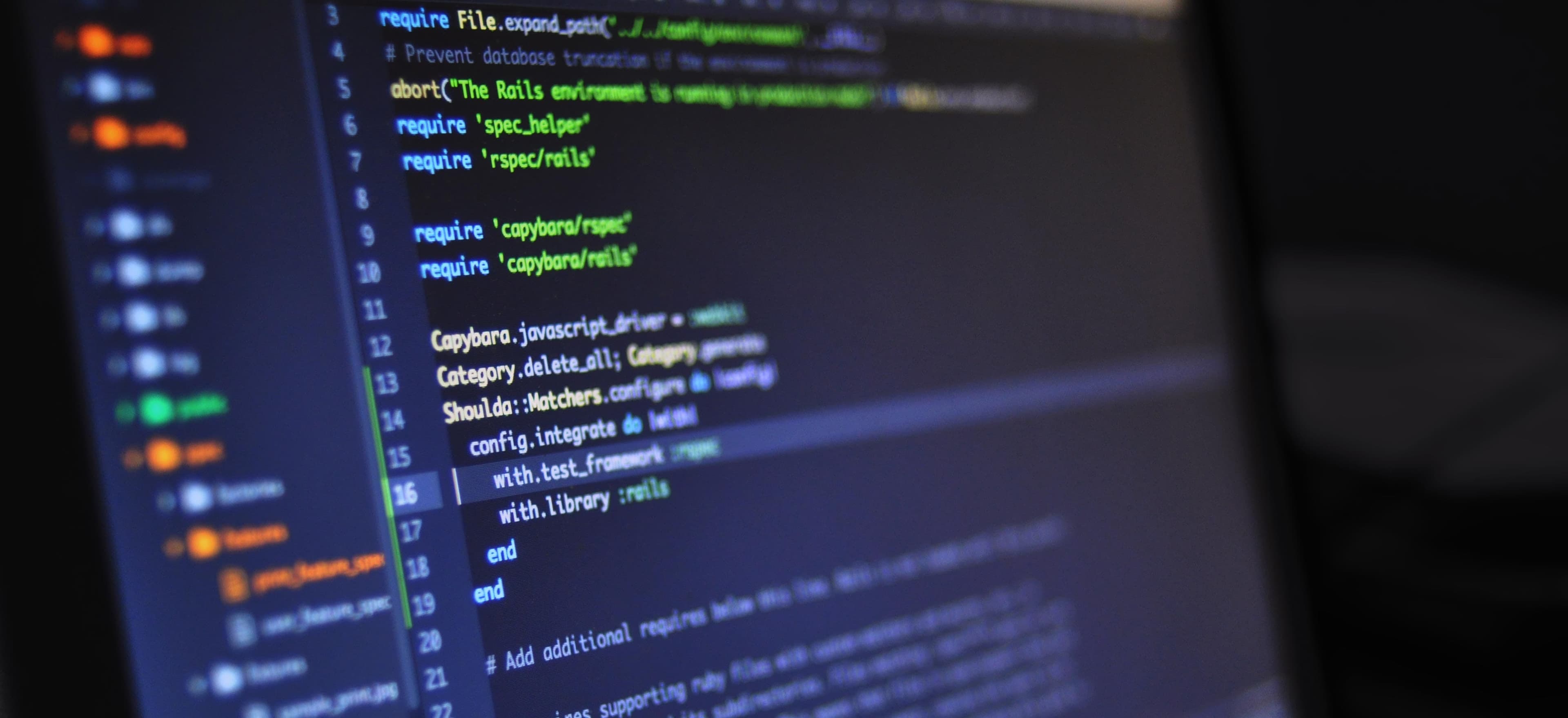
- Published on
Understanding Memory Usage in Java Applications
When developing Java applications, it’s crucial to consider memory usage for optimal performance. Java manages memory through the Java Virtual Machine (JVM), which automatically handles memory allocation and deallocation. However, inefficient memory usage can still lead to performance issues, such as memory leaks or high garbage collection overhead.
In this post, we will explore techniques to optimize memory usage in Java applications, including managing object creation, efficient data structures, and memory profiling tools.
1. Managing Object Creation
Use of String Pool
In Java, the String Pool is a pool of unique strings stored in the heap memory. When a string is created, the JVM first checks if the string already exists in the pool. If it does, the existing reference is returned, saving memory by reusing the same string instance.
String str1 = "Hello";
String str2 = "Hello";
String str3 = new String("Hello");
System.out.println(str1 == str2); // Output: true
System.out.println(str1 == str3); // Output: false
In the example above, str1
and str2
both point to the same string instance in the pool, while str3
points to a new instance created using the new
keyword.
Immutable Objects
Using immutable objects can also help in optimizing memory usage. Immutable objects, once created, cannot be changed. Therefore, they can be cached and reused without the risk of unexpected modifications, leading to efficient memory usage.
public class ImmutableObject {
private final int value;
public ImmutableObject(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
2. Efficient Data Structures
ArrayList vs. LinkedList
Choosing the right data structure can significantly impact memory usage and performance. In Java, ArrayList
and LinkedList
are commonly used to store collections of objects.
ArrayList
is an array-based data structure, providing fast random access but requiring contiguous memory allocation.LinkedList
is a node-based data structure, allowing for efficient insertion and deletion but with higher memory overhead due to the storage of references.
When considering memory optimization, if random access is frequent, and the size of the collection is known, ArrayList
may be a more memory-efficient choice. On the other hand, for frequent insertion and deletion operations, especially with larger collections, LinkedList
might be more suitable despite its higher memory overhead.
List<Integer> arrayList = new ArrayList<>();
List<Integer> linkedList = new LinkedList<>();
Custom Object Pooling
In scenarios where object creation overhead is high, custom object pooling can be implemented. Object pooling involves creating and maintaining a pool of reusable objects, reducing the overhead of creating new objects.
3. Memory Profiling Tools
VisualVM
VisualVM is a visual tool integrating several command-line JDK utilities and lightweight profiling capabilities for both local and remote Java applications. It provides real-time insights into memory usage, garbage collection, and CPU profiling, enabling developers to identify memory-hungry components and optimize their applications.
JProfiler
JProfiler is a fully featured Java performance tuning and profiling tool. It offers memory profiling, thread profiling, and database profiling features. By analyzing memory allocations and object retention, developers can pinpoint memory inefficiencies and optimize their applications.
Final Thoughts
Optimizing memory usage in Java applications is essential for maintaining high performance and efficient resource utilization. By managing object creation, utilizing efficient data structures, and leveraging memory profiling tools, developers can identify and address memory inefficiencies, ensuring their applications run smoothly and effectively.
In conclusion, optimizing memory usage in Java applications is a multifaceted task involving various aspects such as managing object creation, choosing efficient data structures, and utilizing memory profiling tools. By implementing the techniques discussed in this post, developers can significantly improve the memory footprint and overall performance of their Java applications.
Remember, efficient memory management not only enhances performance but also contributes to a better user experience and overall application stability.