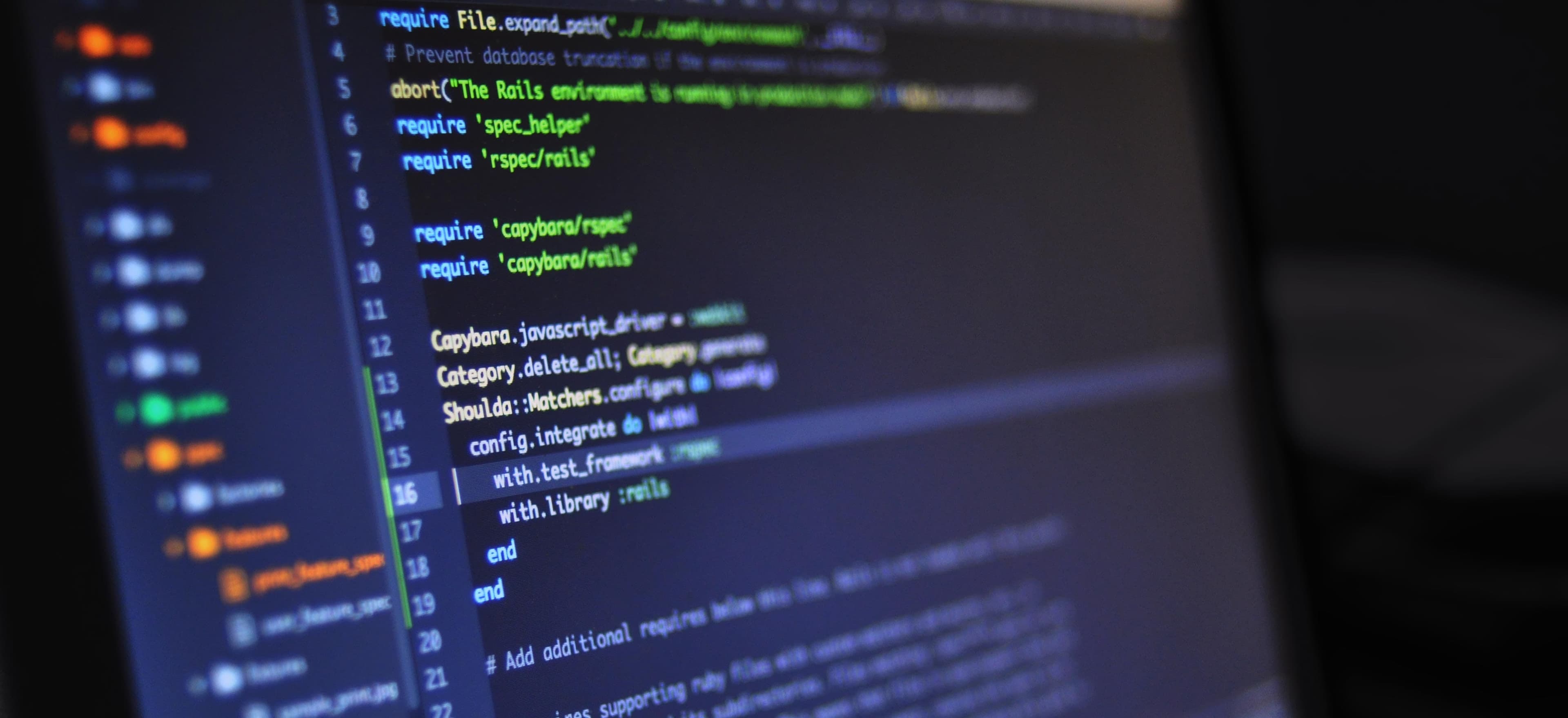
- Published on
Creating a Context Menu with ActionMode Callback in Android
In Android, a context menu provides actions that affect a specific item or context frame in the user interface. The Context Menu is typically invoked by long-pressing a view, such as a list item or an image, and it provides options relevant to the selected item. However, when dealing with multiple item selections, the ActionMode callback becomes essential to handle the contextual action bar and its associated actions.
Understanding ActionMode Callback
ActionMode is a contextual mode of the user interface, which appears when a selection is made. It allows the user to perform customized actions on the selected items, usually displayed as a contextual action bar at the top of the screen. ActionMode.Callback is an interface that provides lifecycle callbacks for the ActionMode. It includes the creation, preparation, and selection action handling methods.
Implementing Context Menu with ActionMode Callback
To illustrate the implementation of a Context Menu with ActionMode Callback, we can consider a scenario where a user long-presses an item in a RecyclerView to select multiple items and then performs action(s) on the selected items using the contextual action bar.
Steps to Implement:
-
Define the Context Menu in RecyclerView Adapter:
public class MyAdapter extends RecyclerView.Adapter<MyAdapter.MyViewHolder> { // ... other methods @Override public void onBindViewHolder(@NonNull MyViewHolder holder, int position) { // Register the context menu for the RecyclerView item holder.itemView.setOnLongClickListener(view -> { holder.itemView.showContextMenu(); return true; }); } }
Here, we register a long-click listener on each item in the RecyclerView to show the context menu when long-pressed.
-
Handle Context Menu Actions:
public class MyActivity extends AppCompatActivity { // ... other methods @Override public void onCreate(Bundle savedInstanceState) { // ... other initialization registerForContextMenu(recyclerView); } @Override public void onCreateContextMenu(ContextMenu menu, View v, ContextMenu.ContextMenuInfo menuInfo) { super.onCreateContextMenu(menu, v, menuInfo); // Inflate the context menu from a menu resource getMenuInflater().inflate(R.menu.context_menu, menu); } @Override public boolean onContextItemSelected(MenuItem item) { // Handle the selected item from the context menu switch (item.getItemId()) { // Handle different menu item selections case R.id.action_delete: // Perform delete action return true; // Handle other actions } return super.onContextItemSelected(item); } }
In this step, we register the RecyclerView for the context menu and then handle the context menu actions in the activity.
-
Implement ActionMode.Callback:
public class MyActivity extends AppCompatActivity implements ActionMode.Callback { // ... other methods private ActionMode actionMode; // ... other methods private void startActionMode() { if (actionMode == null) { actionMode = startSupportActionMode(this); } } @Override public boolean onCreateActionMode(ActionMode mode, Menu menu) { // Inflate the menu resource for the contextual action bar getMenuInflater().inflate(R.menu.contextual_action_menu, menu); return true; } @Override public boolean onPrepareActionMode(ActionMode mode, Menu menu) { // Additional preparation if needed return false; } @Override public boolean onActionItemClicked(ActionMode mode, MenuItem item) { // Handle action item clicks switch (item.getItemId()) { // Handle different action items case R.id.action_share: // Perform share action return true; // Handle other action items } return false; } @Override public void onDestroyActionMode(ActionMode mode) { // Reset any selections or states when action mode is closed actionMode = null; } }
Here, we implement the ActionMode.Callback interface in the activity and define the lifecycle callbacks for the ActionMode.
Summary
In this blog post, we discussed the implementation of a Context Menu with ActionMode Callback in Android. We explored the steps involved in defining the Context Menu in a RecyclerView Adapter, handling Context Menu actions, and implementing the ActionMode.Callback for contextual action bar handling. By following these steps, you can create a seamless user experience for selecting and performing actions on multiple items in your Android app.
By effectively using the Context Menu and ActionMode Callback, you can enhance the usability of your app and provide users with intuitive ways to interact with the content. Whether it's managing multiple items in a list or enabling context-specific actions, these techniques play a crucial role in delivering a polished and engaging user interface.
I hope this guide has provided you with valuable insights into implementing Context Menu with ActionMode Callback in Android, and I encourage you to experiment with these concepts in your own Android projects.
For further reference and deeper insights, you can explore the official Android developer documentation on Context Menu and ActionMode.
Feel free to share your thoughts, experiences, or any additional tips related to implementing Context Menu with ActionMode Callback in Android!
Happy coding!