Fixing Deadlock Issues in Java Concurrency
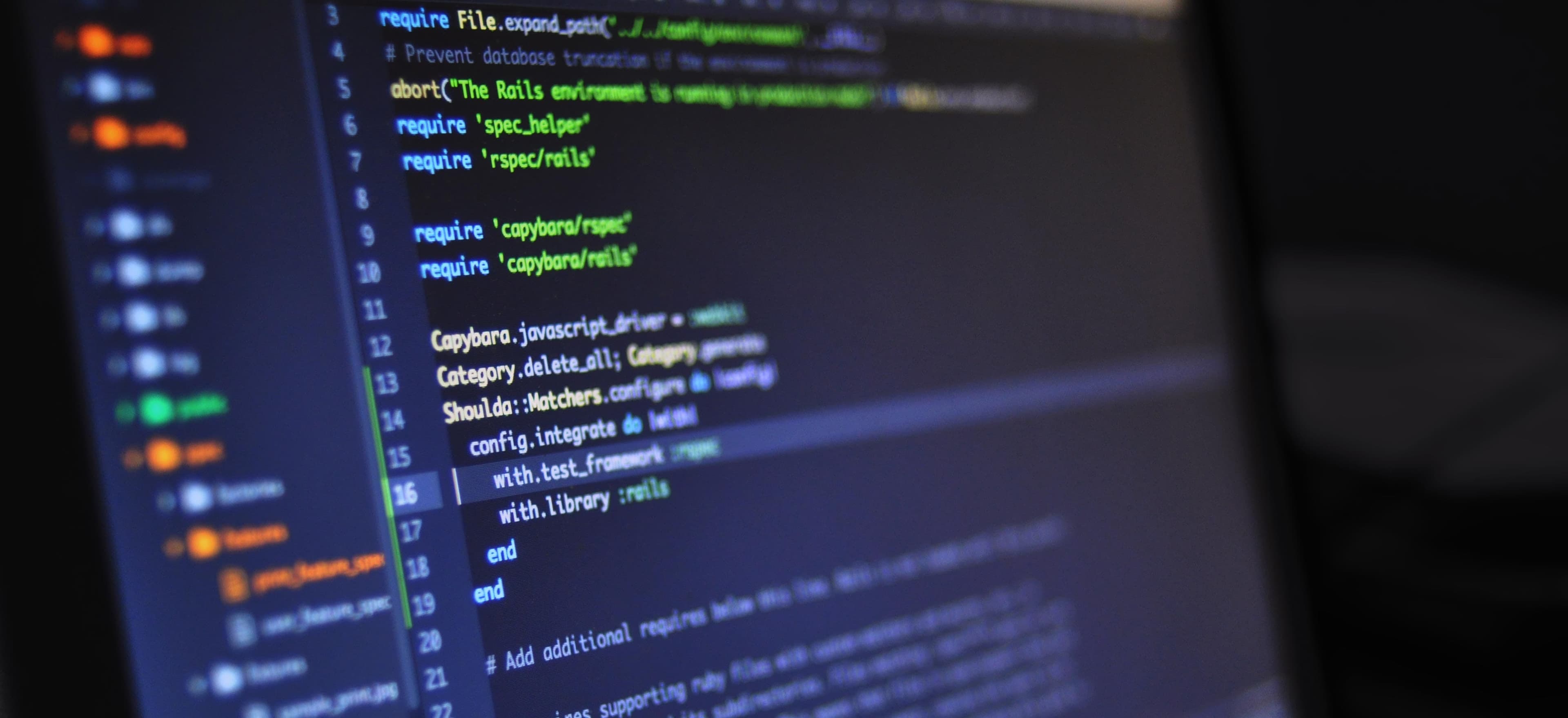
- Published on
Fixing Deadlock Issues in Java Concurrency
Deadlocks are a common issue in parallel programming, especially in Java concurrency. They occur when two or more threads are blocked forever, waiting for each other to release the resources they need. Addressing deadlocks is crucial for a stable, responsive application. In this post, we'll explore some strategies for identifying and mitigating deadlock issues in Java concurrency.
Understanding Deadlocks
Before diving into solutions, let's revisit the conditions that must be present for a deadlock to occur:
- Mutual Exclusion: At least one resource must be held in a non-sharable mode.
- Hold and Wait: A thread must hold a resource and be waiting for another.
- No Preemption: Resources cannot be forcibly taken from the threads holding them.
- Circular Wait: There must exist a circular chain of two or more threads, each waiting for a resource held by the next thread in the chain.
Identifying Deadlocks
The first step in resolving deadlocks is identifying when and where they occur. Java provides several tools to help identify and diagnose deadlock issues:
-
Thread Dump Analysis: Using tools like
jstack
to capture and analyze thread dumps to identify blocked threads and potential deadlock scenarios. -
VisualVM: Profiling tools like VisualVM can help analyze the state of threads and monitor locks and conditions, aiding in identifying potential deadlock situations.
Mitigating Deadlocks
Now, let's explore some strategies to mitigate and prevent deadlocks in Java concurrency:
1. Avoid Nested Locks
Nested locks can lead to deadlocks when not handled carefully. It's crucial to acquire locks in a consistent order to avoid circular dependencies.
synchronized void method1() {
// Critical section
synchronized (resourceX) {
// Do something with resourceX
synchronized (resourceY) {
// Do something with resourceY
}
}
}
2. Use tryLock
Instead of synchronized
Blocks
The tryLock
method from the java.util.concurrent.locks.Lock
interface allows threads to try to acquire a lock and do something else if the lock isn't available, thus preventing potential deadlock situations.
Lock lockX = new ReentrantLock();
Lock lockY = new ReentrantLock();
if (lockX.tryLock()) {
try {
if (lockY.tryLock()) {
try {
// Critical section
} finally {
lockY.unlock();
}
} else {
// Handle the case where lockY is not available
}
} finally {
lockX.unlock();
}
} else {
// Handle the case where lockX is not available
}
3. Using Thread.join
with Timeouts
When using join
to wait for a thread to terminate, using timeouts can ensure that the waiting thread doesn't block indefinitely, preventing potential deadlock situations.
Thread t = new Thread(() -> {
// Do some work
});
t.start();
try {
t.join(1000); // Wait for the thread to die or timeout after 1 second
} catch (InterruptedException e) {
// Handle the interruption
}
4. Resource Ordering
Establish a global ordering on resources and ensure that threads always acquire resources in the same order. This can prevent circular wait conditions.
5. Using java.util.concurrent
Utilities
Utilize higher-level concurrency utilities provided by the java.util.concurrent
package, such as Executor
, Semaphore
, and CountDownLatch
, which inherently handle concurrency and synchronization, reducing the chances of deadlocks.
6. Deadlock Detection and Recovery
Implement mechanisms to detect and recover from deadlocks, such as periodically checking for deadlock conditions and restarting affected threads or processes.
My Closing Thoughts on the Matter
In conclusion, deadlocks in Java concurrency can be a challenging issue to tackle, but with a solid understanding of the root causes and potential solutions, they can be effectively mitigated. By following best practices, avoiding nested locks, utilizing advanced concurrency utilities, and implementing deadlock detection and recovery mechanisms, the risk of deadlocks in Java applications can be significantly reduced.
By embracing these strategies, developers can build more reliable and responsive concurrent applications, ensuring a smoother user experience and fewer unexpected issues related to thread management.
For further reading on Java concurrency and deadlock mitigation, you may find the following resources helpful:
- Java Concurrency in Practice - A comprehensive guide to Java concurrency principles and best practices.
- Oracle's Java Tutorials on Concurrency - Official documentation on Java concurrency by Oracle, covering a wide range of topics related to multithreading and concurrency.
Remember, proactive design and thoughtful consideration of concurrency issues can go a long way in preventing and resolving deadlock problems in Java applications.
Checkout our other articles