Understanding Hazelcast's Distributed Data Structures
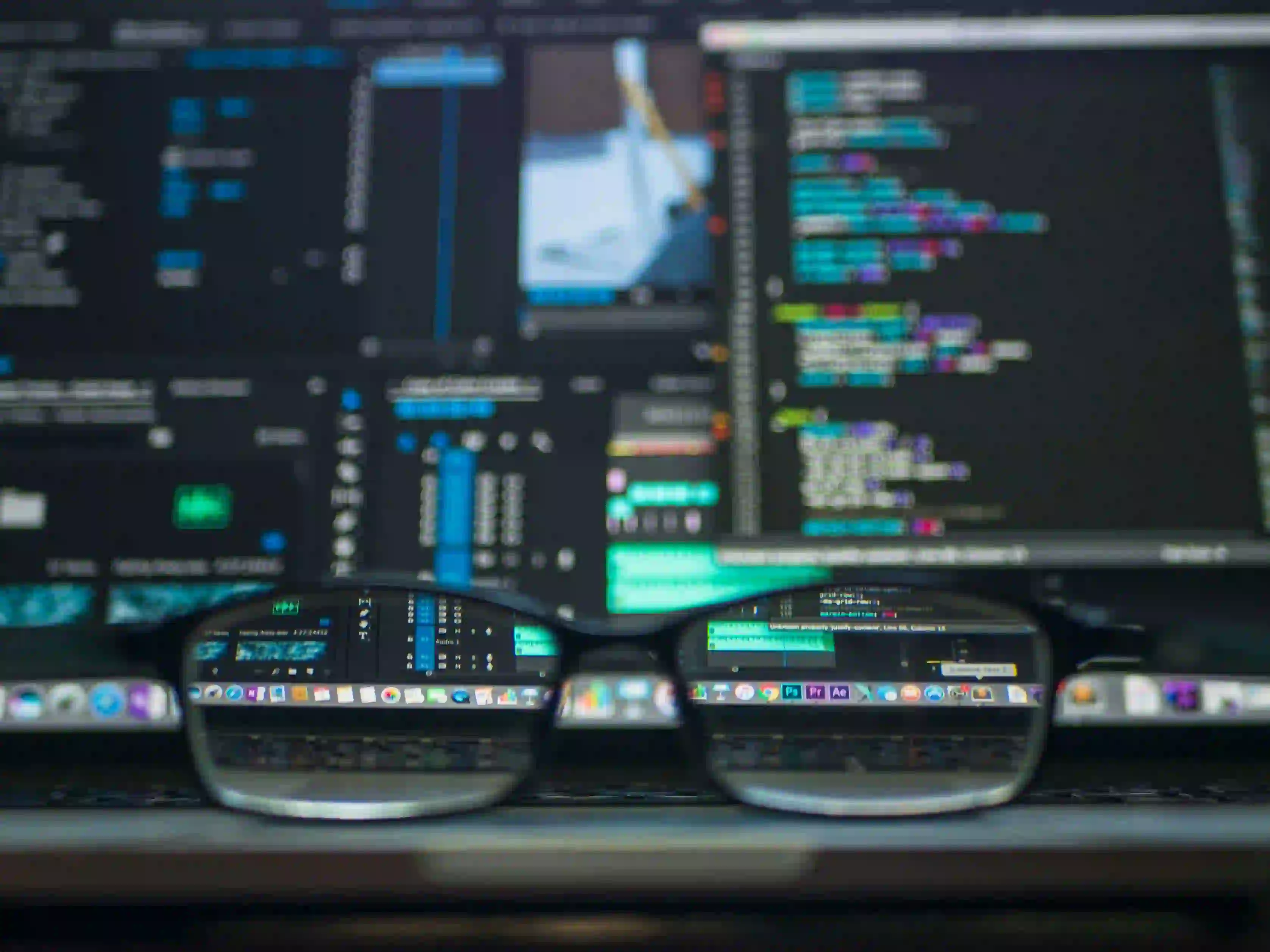
Getting Started with Hazelcast's Distributed Data Structures
When designing and developing applications, especially in the realm of distributed systems, managing data across multiple nodes can be a challenging task. This is where distributed data structures come into play, offering a way to handle data in a distributed manner without compromising on performance or reliability.
In this article, we will explore the world of Hazelcast's distributed data structures, understanding what they are, how they work, and how they can benefit your Java applications.
What are Distributed Data Structures?
Distributed data structures are specialized data structures that are designed to be used in distributed environments, where data is spread across multiple nodes. This enables applications to perform computations, store and retrieve data efficiently, and maintain consistency in distributed systems.
Hazelcast, an in-memory data grid platform, provides a wide range of distributed data structures that can be used in Java applications. These data structures include maps, queues, lists, sets, and more, all of which are designed to be distributed and provide high performance and scalability.
Benefits of Using Hazelcast's Distributed Data Structures
1. Scalability
Hazelcast's distributed data structures offer seamless scalability, allowing you to add or remove nodes from the cluster without impacting the overall performance or availability of the data. This makes it easier to handle increasing workloads and growing datasets without having to re-architect the entire system.
2. Performance
By distributing data across multiple nodes, Hazelcast's distributed data structures can significantly improve application performance by parallelizing operations and reducing network latency. This makes them ideal for high-throughput, low-latency use cases where performance is critical.
3. Fault Tolerance
One of the key advantages of using distributed data structures is their built-in fault tolerance. Hazelcast's data structures automatically handle node failures and ensure that data remains available and consistent, even in the face of network partitions or node outages.
Working with Hazelcast's Distributed Map
Let's dive into a practical example by exploring how to work with Hazelcast's distributed map in a Java application. The distributed map is a key-value data structure that provides a distributed implementation of the java.util.Map
interface, allowing you to store and retrieve key-value pairs across the Hazelcast cluster.
Setting Up Hazelcast
Before getting started with the distributed map, you will need to set up Hazelcast in your Java application. You can do this by adding the Hazelcast dependency to your project's pom.xml
if you are using Maven:
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast</artifactId>
<version>{latest_version}</version>
</dependency>
or to your build.gradle
file if you are using Gradle:
implementation group: 'com.hazelcast', name: 'hazelcast', version: '{latest_version}'
Initializing the Hazelcast Cluster
To initialize the Hazelcast cluster and create a distributed map, you can use the following code snippet:
import com.hazelcast.config.Config;
import com.hazelcast.core.Hazelcast;
import com.hazelcast.core.HazelcastInstance;
import java.util.Map;
public class DistributedMapExample {
public static void main(String[] args) {
Config config = new Config();
HazelcastInstance hazelcastInstance = Hazelcast.newHazelcastInstance(config);
Map<String, String> distributedMap = hazelcastInstance.getMap("my-distributed-map");
// Perform operations on the distributed map
// ...
}
}
In this code snippet, we are creating a Hazelcast instance and obtaining a reference to the distributed map named "my-distributed-map". This map can now be used to store and retrieve key-value pairs across the cluster.
Performing Operations on the Distributed Map
Once the distributed map is initialized, you can perform various operations such as putting, getting, and removing key-value pairs. Here’s a simple example:
// Put a key-value pair into the distributed map
distributedMap.put("key1", "value1");
// Retrieve the value associated with a key from the distributed map
String value = distributedMap.get("key1");
// Remove a key-value pair from the distributed map
String removedValue = distributedMap.remove("key1");
By using Hazelcast's distributed map, you can store and retrieve data across the cluster seamlessly, without having to worry about the underlying distribution and synchronization mechanisms.
Closing Remarks
In conclusion, Hazelcast's distributed data structures are a powerful addition to any Java developer's toolkit when it comes to building distributed systems. They offer scalability, performance, and fault tolerance, making them well-suited for a wide range of distributed applications.
In this article, we have only scratched the surface of what is possible with Hazelcast's distributed data structures. To delve deeper into this topic and explore more advanced use cases, I recommend referring to the official Hazelcast documentation.
So, the next time you find yourself working on a distributed Java application, consider leveraging the capabilities of Hazelcast's distributed data structures to simplify and streamline your data management needs.
Start exploring Hazelcast's distributed data structures today and unlock the full potential of your distributed Java applications!