Preventing SQL Injection in Java
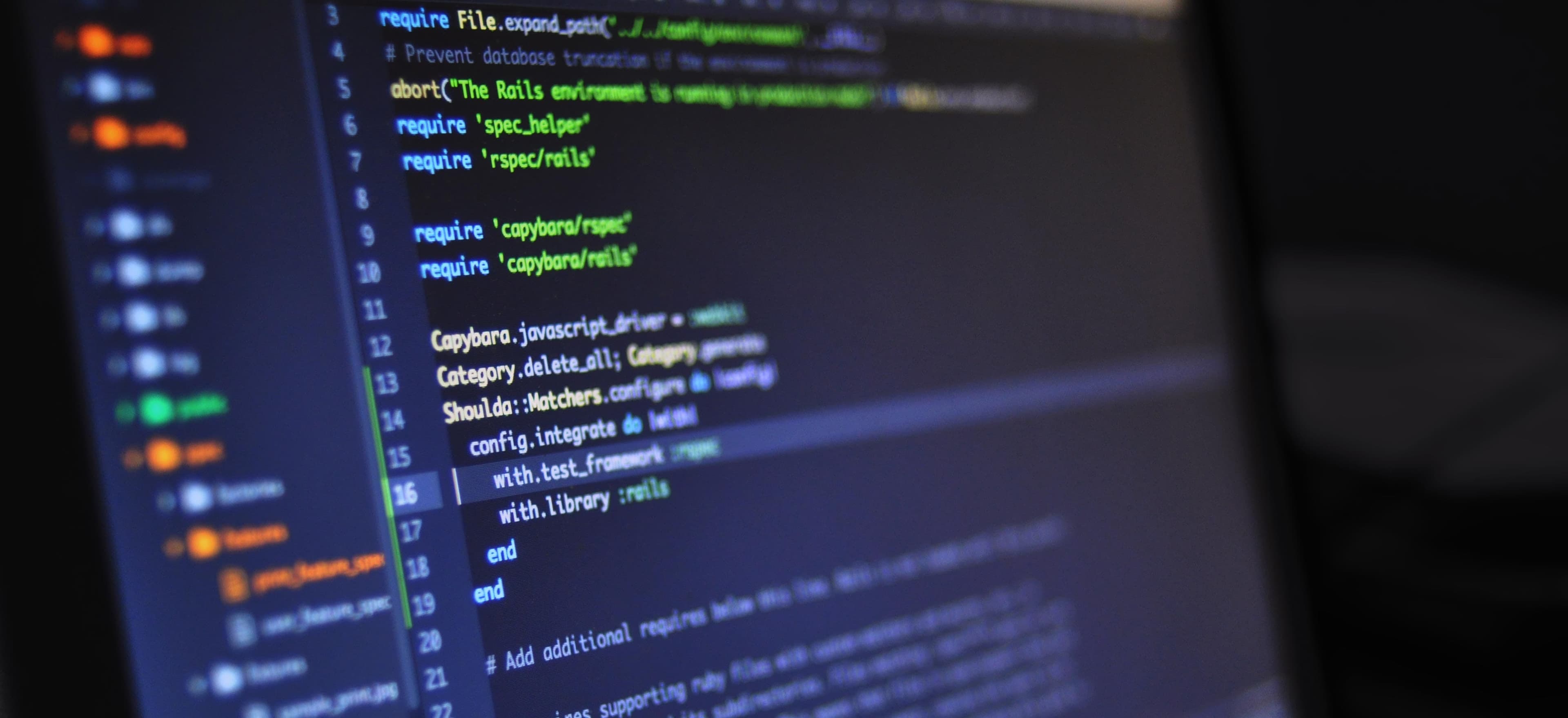
- Published on
Preventing SQL Injection in Java
SQL injection is a common security vulnerability in web applications that occurs when an attacker is able to insert malicious SQL code into a query. This can lead to unauthorized access, data manipulation, and potentially devastating consequences for your application and its users.
In this article, we will explore how to prevent SQL injection in Java, using best practices and tools to ensure the security of your application.
Parameterized Queries
One of the most effective ways to prevent SQL injection is to use parameterized queries. This involves using prepared statements with parameter placeholders, which are then bound to specific values. Let's look at an example using JDBC (Java Database Connectivity):
String username = request.getParameter("username");
String password = request.getParameter("password");
String sql = "SELECT * FROM users WHERE username = ? AND password = ?";
try (Connection connection = DriverManager.getConnection(url, user, password);
PreparedStatement statement = connection.prepareStatement(sql)) {
statement.setString(1, username);
statement.setString(2, password);
try (ResultSet resultSet = statement.executeQuery()) {
// Process the result set
}
} catch (SQLException e) {
// Handle SQL exception
}
In this example, we use PreparedStatement
to create a parameterized query with placeholders (?
). Then, we use the setString
method to bind the values to the placeholders. This way, the SQL query is constructed separately from the parameters, preventing any possibility of SQL injection.
Input Validation
Another crucial step in preventing SQL injection is input validation. It's essential to validate and sanitize any user input before using it in a SQL query. This includes enforcing data types, length limits, and format checks.
String username = request.getParameter("username");
if (username.matches("[a-zA-Z0-9]+")) {
// Proceed with the query
} else {
// Handle invalid input
}
In this example, we validate the username input using a regular expression to ensure it only contains alphanumeric characters. If the input doesn't meet the criteria, it should be deemed invalid and handled accordingly.
Use Object-Relational Mapping (ORM) Libraries
Object-Relational Mapping (ORM) libraries such as Hibernate and JPA provide a higher level of abstraction for database interactions, which can inherently mitigate the risk of SQL injection. These libraries handle parameterization and escaping of values, reducing the likelihood of introducing vulnerabilities.
Here's an example of using JPA to perform a parameterized query:
String username = request.getParameter("username");
String password = request.getParameter("password");
EntityManager em = // obtain entity manager
CriteriaBuilder cb = em.getCriteriaBuilder();
CriteriaQuery<User> cq = cb.createQuery(User.class);
Root<User> user = cq.from(User.class);
cq.select(user).where(cb.equal(user.get("username"), username), cb.equal(user.get("password"), password));
List<User> result = em.createQuery(cq).getResultList();
In this code snippet, we utilize JPA's Criteria API to construct a parameterized query without directly writing SQL. The framework takes care of handling the parameters securely, reducing the risk of SQL injection.
Escaping Special Characters
When it's not feasible to use parameterized queries, and direct SQL string manipulation is necessary, it's crucial to escape special characters to prevent injection attacks. Most database engines provide functions for escaping characters, and many libraries offer methods to sanitize input for different databases.
String username = request.getParameter("username");
String password = request.getParameter("password");
// Use library-specific method to escape special characters
String sql = "SELECT * FROM users WHERE username = '" + escape(username) + "' AND password = '" + escape(password) + "'";
In this example, we assume a hypothetical escape
function provided by your database library. This function ensures that any special characters in the input are properly escaped to prevent them from being interpreted as part of the SQL query.
Limiting Database Permissions
Another layer of defense against SQL injection is to limit the permissions of the database user used by your Java application. By following the principle of least privilege, you can reduce the potential impact of a successful injection attack. Only grant the necessary permissions for the application to perform its intended operations.
A Final Look
Preventing SQL injection is a critical aspect of developing secure and robust Java applications. By utilizing parameterized queries, input validation, ORM libraries, character escaping, and limiting database permissions, you can significantly reduce the risk of SQL injection attacks.
Remember, security is an ongoing process, so always stay informed about the latest best practices and tools for safeguarding your Java applications against evolving threats.
By incorporating these techniques into your Java development workflow, you can build applications that not only perform effectively but also maintain the highest standards of security and integrity.
Incorporating these best practices into your Java application will not only make it more robust but also ensure the security of your users' data.
For additional in-depth information on SQL injection and best practices for mitigating it, I recommend checking out the OWASP SQL Injection Prevention Cheat Sheet and the Java Secure Coding Guidelines provided by Oracle.
Keep coding securely!
The above post demonstrates how developers can prevent SQL injection in Java applications, covering a range of best practices and tools to ensure the security of the code. From utilizing parameterized queries to employing input validation and ORM libraries, this comprehensive guide provides essential insights for safeguarding Java applications against SQL injection attacks.