Optimizing Maven Build Performance
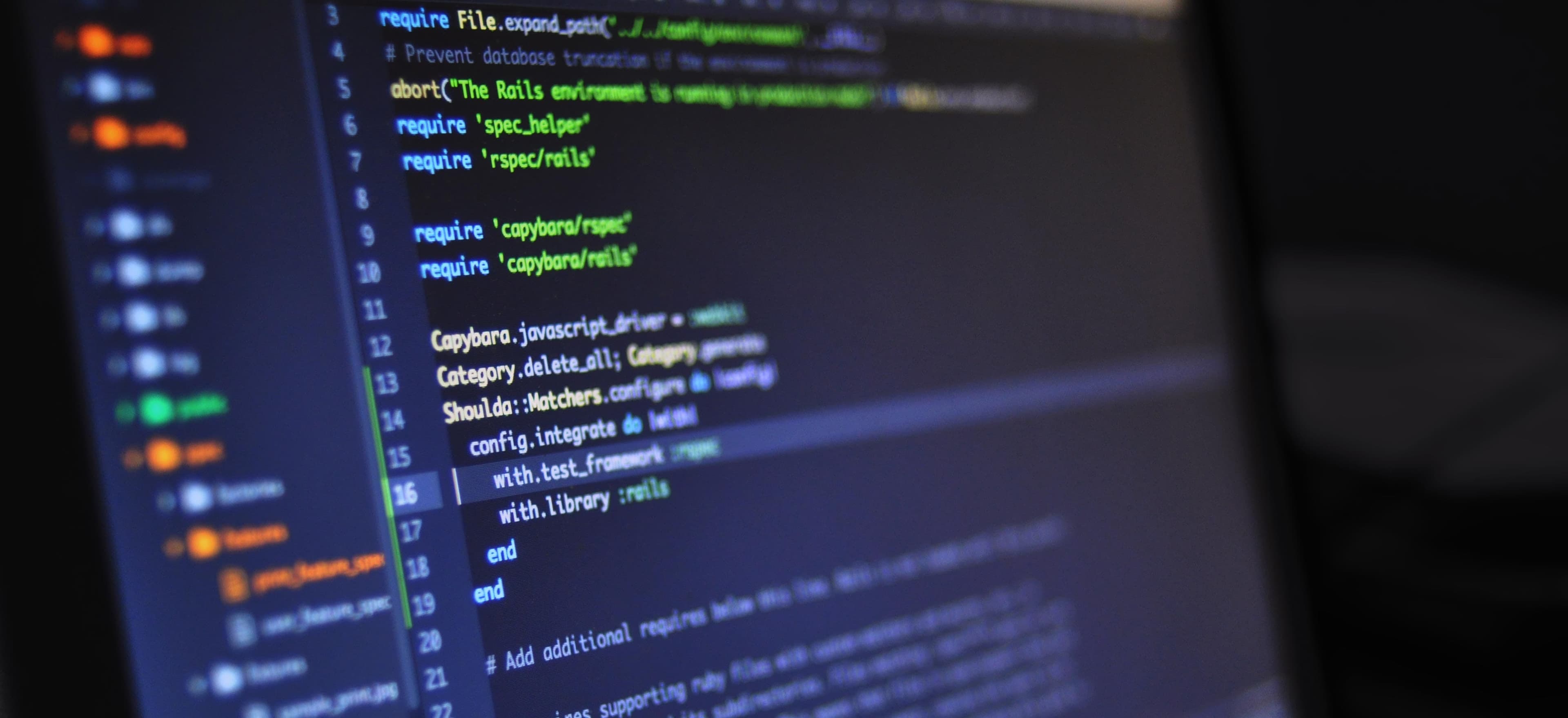
- Published on
Optimizing Maven Build Performance
Maven is a powerful build automation tool that is widely used in the Java ecosystem to manage and build projects. However, as projects grow in size and complexity, Maven build times can become a significant pain point for developers. In this article, we will explore techniques and best practices to optimize Maven build performance, ensuring that your build processes run efficiently and swiftly.
1. Use Maven Wrapper
The Maven Wrapper is a tool that allows you to execute Maven builds without having Maven installed on the system. It provides a convenient way to ensure that the correct version of Maven is used for building the project, eliminating version mismatches and ensuring consistency across different developer environments.
To integrate the Maven Wrapper into your project, execute the following command in the project's root directory:
mvn -N io.takari:maven:0.7.7:wrapper
This will create the necessary wrapper files in the project, and developers can use mvnw
(for Unix-based systems) or mvnw.cmd
(for Windows) to execute Maven builds without having to install Maven separately.
2. Parallel Builds
Maven supports parallel builds, allowing multiple modules to be built concurrently, thus reducing overall build times. To enable parallel builds, you can set the <modules>
element in the <build>
section of the parent pom.xml
:
<build>
<modules>
<module>module1</module>
<module>module2</module>
<!-- Other modules -->
</modules>
</build>
By specifying the modules to build in parallel, you can take advantage of multi-core processors and speed up the build process significantly.
3. Profile-based Configuration
Maven allows the use of profiles to customize build configurations for different environments. This can be leveraged to optimize build performance by specifying different settings for development, testing, and production builds.
For example, you can define a profile specifically for faster local development builds by excluding certain plugins or tasks that are not essential for day-to-day development:
<profiles>
<profile>
<id>development</id>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<skipMain>true</skipMain>
</configuration>
</plugin>
<!-- Other plugins to exclude -->
</plugins>
</build>
</profile>
</profiles>
By activating the development
profile for local development, you can skip certain time-consuming tasks and speed up build times significantly.
4. Dependency Management
Efficient dependency management is crucial for optimizing Maven build performance. Here are some best practices to consider:
a. Dependency Scope
Specify the appropriate dependency scope to prevent unnecessary dependencies from being included in the build. For example, if a dependency is only required for compilation and not at runtime, it should be marked with a provided
scope:
<dependency>
<groupId>...</groupId>
<artifactId>...</artifactId>
<version>...</version>
<scope>provided</scope>
</dependency>
b. Dependency Exclusions
Exclude transitive dependencies that are not needed for the project to reduce unnecessary bloat in the build:
<dependency>
<groupId>org.example</groupId>
<artifactId>example-artifact</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>org.unwanted</groupId>
<artifactId>unwanted-dependency</artifactId>
</exclusion>
</exclusions>
</dependency>
By carefully managing dependencies, you can streamline the build process and reduce unnecessary overhead.
5. Incremental Builds
Maven supports incremental builds, which allow it to recompile only the necessary modules and classes that have changed since the last build. This can significantly reduce build times, especially for large projects with many source files.
To enable incremental builds, ensure that the <sourceDirectory>
and <testSourceDirectory>
elements are properly configured within the project's pom.xml
:
<build>
<sourceDirectory>src/main/java</sourceDirectory>
<testSourceDirectory>src/test/java</testSourceDirectory>
</build>
Additionally, you can configure the maven-compiler-plugin
to enable incremental compilation:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<useIncrementalCompilation>true</useIncrementalCompilation>
</configuration>
</plugin>
</plugins>
</build>
By enabling incremental builds, Maven can intelligently recompile only the necessary parts of the project, leading to faster build times during development cycles.
6. Externalizing Configuration
Externalizing configuration settings, such as plugin configurations and properties, can improve build performance by allowing for quicker iterative changes without the need for full project recompilation.
One way to achieve this is by using external property files or environment variables to supply configurable values, reducing the need to modify and rebuild the project for minor configuration tweaks.
Lessons Learned
Optimizing Maven build performance is crucial for maintaining developer productivity and project efficiency. By leveraging techniques such as parallel builds, profile-based configuration, efficient dependency management, incremental builds, and externalizing configuration, you can streamline your build processes and ensure swift, efficient builds for your Java projects.
By implementing these best practices, you can reduce build times, improve developer satisfaction, and ultimately deliver high-quality software more effectively.
For more information on Maven build optimization, you can refer to the official Maven documentation and Apache Maven Performance.