Resolving Coreference in Texts with Stanford CoreNLP
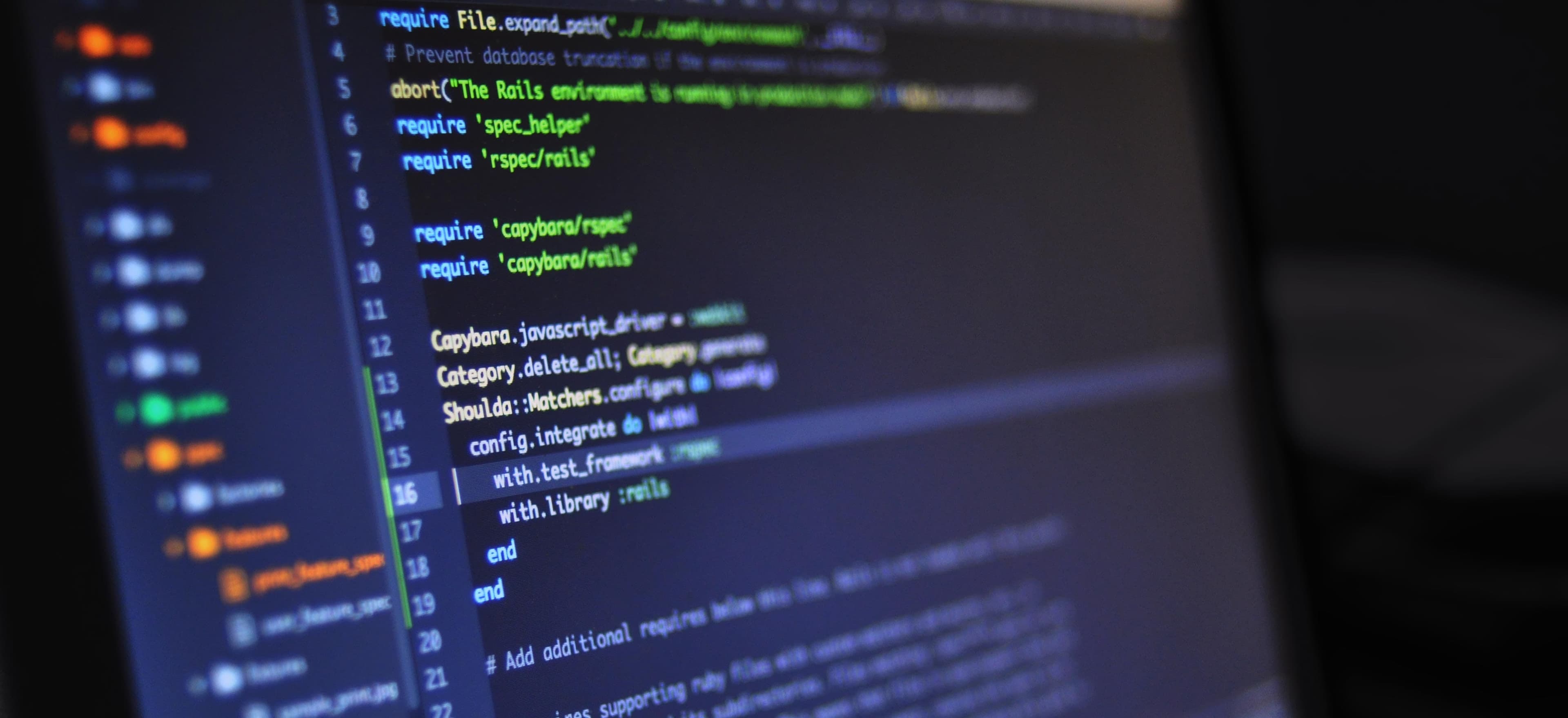
- Published on
Understanding Coreference Resolution with Stanford CoreNLP
When dealing with natural language processing (NLP) tasks, one common challenge is resolving coreference in texts. Coreference resolution involves identifying words or phrases that refer to the same entity. For example, in the sentence "The cat chased its tail because it was bored," the words "its" and "it" both refer to "the cat."
In this blog post, we will explore how to use Stanford CoreNLP, a popular Java library for NLP tasks, to perform coreference resolution. We will cover the basics of coreference resolution, how Stanford CoreNLP can help us tackle this task, and provide practical examples to demonstrate its usage.
What is Coreference Resolution?
Coreference resolution is the task of determining when two or more expressions in a text refer to the same entity. This is crucial for understanding the content and meaning of a document, as it helps in creating a coherent representation of the text.
For instance, consider the sentence: "John said he would come to the party."
Here, "he" refers to "John." Resolving this coreference helps in understanding that it is John who would come to the party.
Using Stanford CoreNLP for Coreference Resolution
Stanford CoreNLP is a robust natural language processing toolkit developed by the Stanford NLP Group. It provides a suite of tools for many NLP tasks, including part-of-speech (POS) tagging, named entity recognition (NER), and coreference resolution.
To get started with coreference resolution using Stanford CoreNLP in your Java project, you can add the following Maven dependency to your pom.xml
:
<dependency>
<groupId>edu.stanford.nlp</groupId>
<artifactId>stanford-corenlp</artifactId>
<version>4.3.0</version>
</dependency>
After adding the dependency, you can utilize the CoreNLP library to perform coreference resolution within your Java application.
Let's delve into an example to showcase how Stanford CoreNLP can be used for coreference resolution.
Example of Coreference Resolution with Stanford CoreNLP
Suppose we have the following text: "Barack Obama was born in Hawaii. He served as the 44th president of the United States. Obama's presidency began in 2009."
We want to identify and resolve the coreferences in this passage. Using Stanford CoreNLP, we can achieve this by defining a pipeline and processing the text. Here's how it can be done:
import edu.stanford.nlp.coref.CorefCoreAnnotations;
import edu.stanford.nlp.coref.data.CorefChain;
import edu.stanford.nlp.ling.CoreAnnotations;
import edu.stanford.nlp.ling.CoreLabel;
import edu.stanford.nlp.pipeline.Annotation;
import edu.stanford.nlp.pipeline.StanfordCoreNLP;
import edu.stanford.nlp.util.CoreMap;
import java.util.List;
import java.util.Map;
import java.util.Properties;
public class CoreferenceResolutionExample {
public static void main(String[] args) {
// Set up the CoreNLP pipeline
Properties props = new Properties();
props.setProperty("annotators", "tokenize, ssplit, pos, lemma, ner, parse, coref");
StanfordCoreNLP pipeline = new StanfordCoreNLP(props);
// Create an empty Annotation just with the given text
Annotation document = new Annotation("Barack Obama was born in Hawaii. He served as the 44th president of the United States. Obama's presidency began in 2009.");
// Run all Annotators on this text
pipeline.annotate(document);
// Get the coref chain annotation
Map<Integer, CorefChain> corefChains = document.get(CorefCoreAnnotations.CorefChainAnnotation.class);
// Iterate over the coreference chains
for (Map.Entry<Integer, CorefChain> entry : corefChains.entrySet()) {
System.out.println("Chain " + entry.getKey() + ":");
CorefChain corefChain = entry.getValue();
List<CorefChain.CorefMention> mentions = corefChain.getMentionsInTextualOrder();
CorefChain.CorefMention representative = corefChain.getRepresentativeMention();
System.out.println("Representative: " + representative.mentionSpan);
for (CorefChain.CorefMention mention : mentions) {
if (!mention.mentionSpan.equals(representative.mentionSpan)) {
System.out.println(" " + mention.mentionSpan);
}
}
}
}
}
In this example, we create a Stanford CoreNLP pipeline with the required annotators, process the text, and retrieve the coreference chains. We then iterate over the chains and print out the representative mentions along with their corresponding mentions in the text.
Closing Remarks
In this post, we delved into the concept of coreference resolution and how Stanford CoreNLP can be used to tackle this NLP task. We showcased a practical example of leveraging Stanford CoreNLP to identify and resolve coreferences in a given text.
Understanding coreference in texts is essential for various NLP applications, including information extraction, question answering systems, and summarization. By incorporating coreference resolution into our NLP pipelines, we can extract more meaningful insights from textual data.
Incorporating Stanford CoreNLP's coreference resolution capabilities empowers Java developers to build sophisticated NLP applications that can comprehend and analyze text more effectively. Whether you're working on chatbots, document analysis, or any other NLP-related task, knowing how to leverage tools like Stanford CoreNLP for coreference resolution can elevate the quality and depth of your applications.
Now that you have a foundational understanding of coreference resolution and how to utilize Stanford CoreNLP for this task, you can further explore and integrate this knowledge into your NLP projects.
Continue to explore more about coreference resolution, NLP, and Stanford CoreNLP to enhance your proficiency in natural language processing and build powerful and intelligent Java applications.