Detecting Memory Leaks in Spring Web Apps
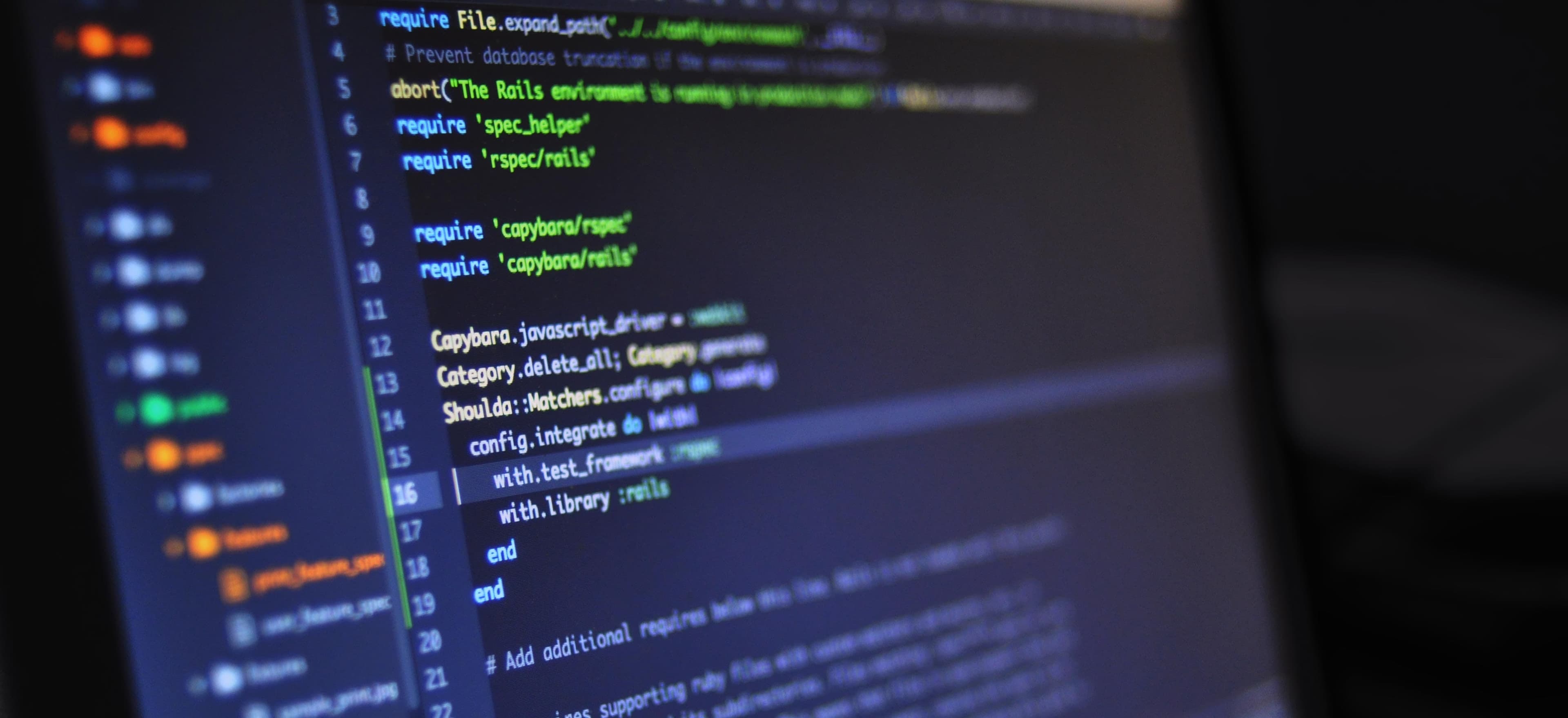
- Published on
Detecting Memory Leaks in Spring Web Apps
Memory leaks are a common issue in Java web applications, including those built using the Spring framework. These leaks can lead to performance degradation and even application crashes if not addressed promptly. In this article, we will explore the common causes of memory leaks in Spring web apps and discuss ways to detect and prevent them.
Causes of Memory Leaks in Spring Web Apps
Memory leaks in Spring web apps can be caused by various factors, including but not limited to:
-
Unclosed Resources: Failing to properly close database connections, file handles, or network sockets can lead to memory leaks.
-
Inefficient Caching: Improper usage of caching mechanisms, such as the
@Cacheable
annotation in Spring, can result in memory leaks if cached objects are not managed correctly. -
Static References: Holding onto references to objects for longer than necessary, especially in static contexts, can prevent the garbage collector from reclaiming memory.
-
Improper Session Management: In web applications, failing to manage session objects properly can lead to memory leaks, especially when dealing with long-lived sessions.
Now that we have identified the potential causes of memory leaks in Spring web apps, let's discuss how to detect these issues.
Detecting Memory Leaks
1. Monitoring Heap Memory
One of the most effective ways to detect memory leaks in a Spring web app is to monitor the application's heap memory usage. Tools like JVisualVM, which comes with the JDK, can be used to monitor heap memory usage in real time. By observing the heap memory over an extended period, you can identify abnormal patterns such as a steady increase in memory usage, which could indicate a memory leak.
2. Using Profiling Tools
Profiling tools such as YourKit and JProfiler can provide in-depth insights into memory usage and help identify potential memory leaks in Spring web apps. These tools can identify objects that are consuming excessive memory and analyze their references to pinpoint any leaks.
3. Heap Dump Analysis
Taking heap dumps at regular intervals and analyzing them can reveal valuable information about memory usage and potential leaks. Tools like Eclipse MAT (Memory Analyzer Tool) can be used to analyze heap dumps and identify objects that are causing memory leaks.
Preventing Memory Leaks
Now that we have discussed how to detect memory leaks, let's explore some preventive measures to minimize the risk of memory leaks in Spring web apps.
1. Proper Resource Management
Ensure that all resources, such as database connections and file handles, are properly closed after use. Using try-with-resources or try-finally blocks can help in ensuring resource closure even in the event of exceptions.
try (Connection connection = dataSource.getConnection()) {
// Use the connection
} catch (SQLException e) {
// Handle the exception
}
2. Efficient Caching Strategies
When using caching mechanisms in Spring, make sure to configure cache eviction policies and consider the memory impact of cached objects. Avoid caching large or long-lived objects that could potentially lead to memory leaks.
3. Avoiding Static References
Be mindful of holding references to objects in static contexts, as they can persist for the entire duration of the application and prevent the garbage collector from reclaiming memory. Use dependency injection and non-static methods whenever possible.
4. Session Management Best Practices
In web applications, implement session management best practices to ensure that session objects are not kept alive longer than necessary. Consider using frameworks like Spring Session to manage session-related operations effectively.
By implementing these preventive measures, you can significantly reduce the likelihood of memory leaks in your Spring web apps.
The Last Word
Memory leaks in Spring web apps can have detrimental effects on application performance and stability. By monitoring heap memory, using profiling tools, and analyzing heap dumps, you can effectively detect memory leaks in your application. Additionally, adopting proper resource management, efficient caching strategies, and session management best practices can help prevent memory leaks from occurring in the first place.
Detecting and preventing memory leaks is crucial for maintaining the reliability and performance of Spring web apps. By following the best practices outlined in this article, you can minimize the risk of memory leaks and ensure smooth operation of your applications.
Remember, proactive detection and prevention are key to maintaining healthy memory management in Spring web apps.
Here is a great resource for further understanding and addressing memory leaks in Java applications.
If you're interested in exploring more about performance optimization in Spring applications, check out this link.
Happy coding!