Solving Special Presentation Requirements in Grails Domain Classes
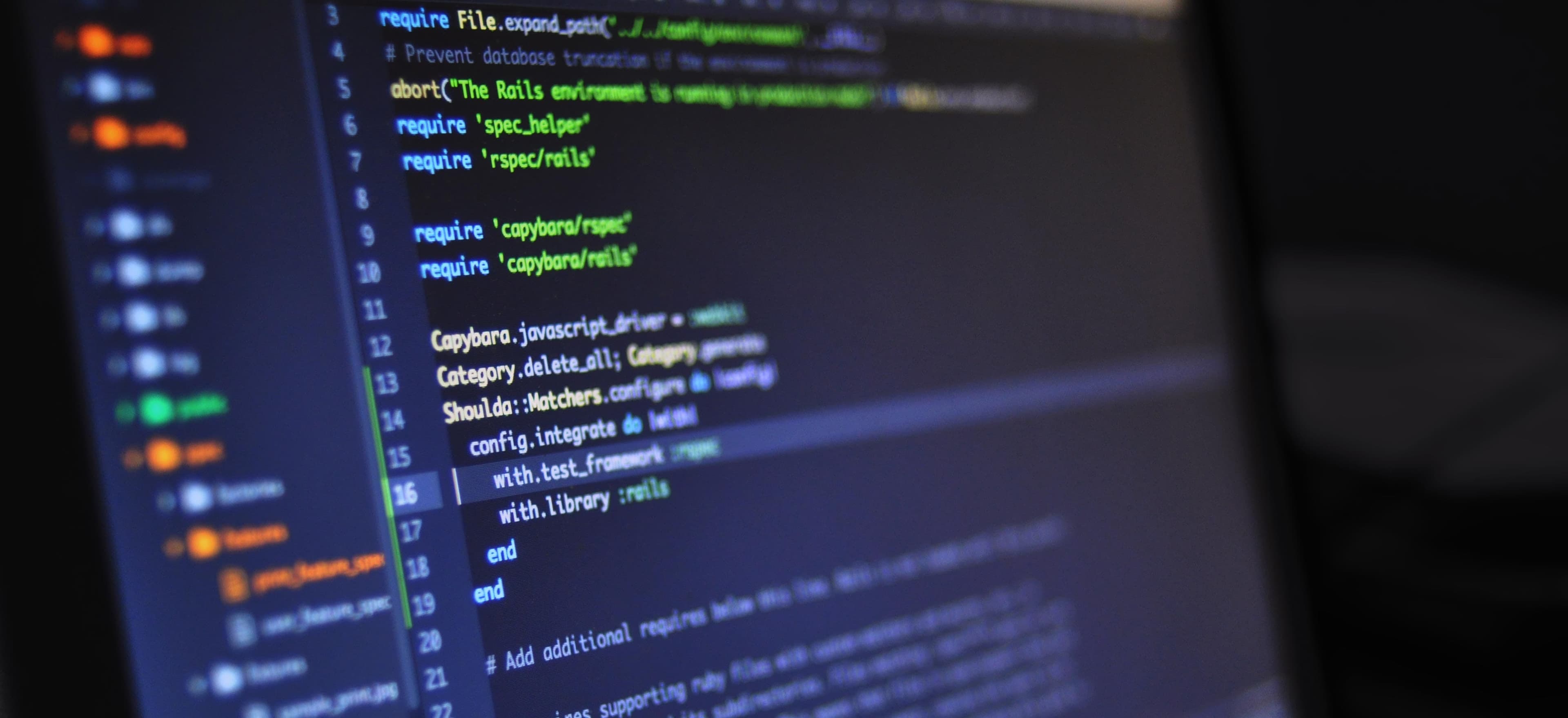
- Published on
Solving Special Presentation Requirements in Grails Domain Classes
When working with Grails, a widely used web application framework based on the Groovy programming language and the Java Virtual Machine (JVM), you might come across special presentation requirements for domain classes. These requirements could range from formatting dates, customizing string representations, or any other specific formatting needs for your domain model. In this article, we will explore how to address these special presentation requirements in Grails domain classes. We will cover various techniques and best practices to achieve custom representations while maintaining the integrity of your domain model.
Understanding the Special Presentation Requirements
Before we delve into the implementation, it's crucial to understand the special presentation requirements specific to your domain classes. Is there a need to display dates in a certain format? Do you require specific string representations for certain properties? Identifying these requirements will guide us in selecting the most appropriate approach for solving them.
Leveraging the String
method in the Domain Class
Grails domain classes are Groovy classes, and as such, they inherit all the methods available to Groovy objects. One of these methods is the String
method, which allows you to customize the string representation of an object when it's converted to a string. By overriding this method in your domain class, you can provide a custom presentation for instances of that class.
Let's consider a simple Product
domain class with a releaseDate
property, and we want to format the releaseDate
in a specific way when it's converted to a string. We can achieve this by overriding the String
method in the Product
class.
class Product {
String name
Date releaseDate
String toString() {
"${name} - Released on: ${releaseDate.format('dd/MM/yyyy')}"
}
}
In this example, we have overridden the String
method to customize the string representation of the Product
instance. When the toString
method is called, it will return a string in the specified format, combining the product name with the formatted release date.
Using Grails' format
Method
Grails provides a convenient way to format objects using the format
method, which is available for date objects. This method allows you to specify the desired format for the date representation. By using the format
method directly in the GSP (Groovy Server Pages) views, you can achieve the required presentation without modifying the domain class itself.
Let's consider the same Product
class with the releaseDate
property, and we want to display the formatted releaseDate
in a GSP view. We can leverage the format
method to achieve this.
class Product {
String name
Date releaseDate
}
In the GSP view:
<p>${product.name} - Released on: ${product.releaseDate.format('dd/MM/yyyy')}</p>
In this example, we utilize the format
method directly in the GSP view to display the formatted releaseDate
. This approach keeps the domain class clean and places the responsibility of presentation on the view layer.
Implementing Custom Formatting Methods
For more complex presentation requirements, you might need to implement custom formatting methods within your domain class. These methods can encapsulate the logic for generating specific representations of your domain object properties, providing a clean and modular approach to handling special presentation requirements.
Let's enhance our Product
class by introducing a custom formatting method for the releaseDate
property.
class Product {
String name
Date releaseDate
String formattedReleaseDate() {
releaseDate.format('dd/MM/yyyy')
}
}
In this example, we have added a formattedReleaseDate
method to the Product
class, which encapsulates the logic for formatting the releaseDate
. This method can be called from the view layer to obtain the formatted representation of the releaseDate
.
Leveraging Grails' Data Binding and Type Conversion
Grails provides powerful data binding and type conversion capabilities, which can be utilized to handle special presentation requirements. By defining custom property editors or type converters, you can influence the way data is presented and parsed in your domain classes.
For instance, let's say we want to handle custom date formatting during data binding for our Product
class. We can create a custom property editor to achieve this.
class Product {
String name
Date releaseDate
static constraints = {
releaseDate bindable: true
}
def releaseDatePropertyEditor = {
binder ->
binder.registerCustomEditor(
Date,
new CustomDatePropertyEditor('dd/MM/yyyy')
)
}
}
In this example, we've defined a custom property editor for the releaseDate
property, specifying the desired date format. This ensures that when data is bound to the releaseDate
property, it will be formatted according to the custom editor configuration.
Final Considerations
In this article, we explored various techniques for addressing special presentation requirements in Grails domain classes. By leveraging methods like toString
, format
, custom formatting methods, and Grails' data binding capabilities, you can achieve custom representations while keeping your domain classes clean and focused on their core responsibilities. Understanding the specific presentation needs of your domain model and choosing the most suitable approach will enable you to create a clear and maintainable codebase for your Grails application.
By applying these techniques, you can enhance the user experience and improve the overall presentation of your application, while maintaining the integrity of your domain model. As you continue to work with Grails, being mindful of these special presentation requirements and effectively addressing them will contribute to the overall quality and usability of your application.
As always, feel free to leave your comments and share your experiences with handling special presentation requirements in Grails domain classes. Happy coding!