Enhancing Big Data Analytics with Apache Arrow
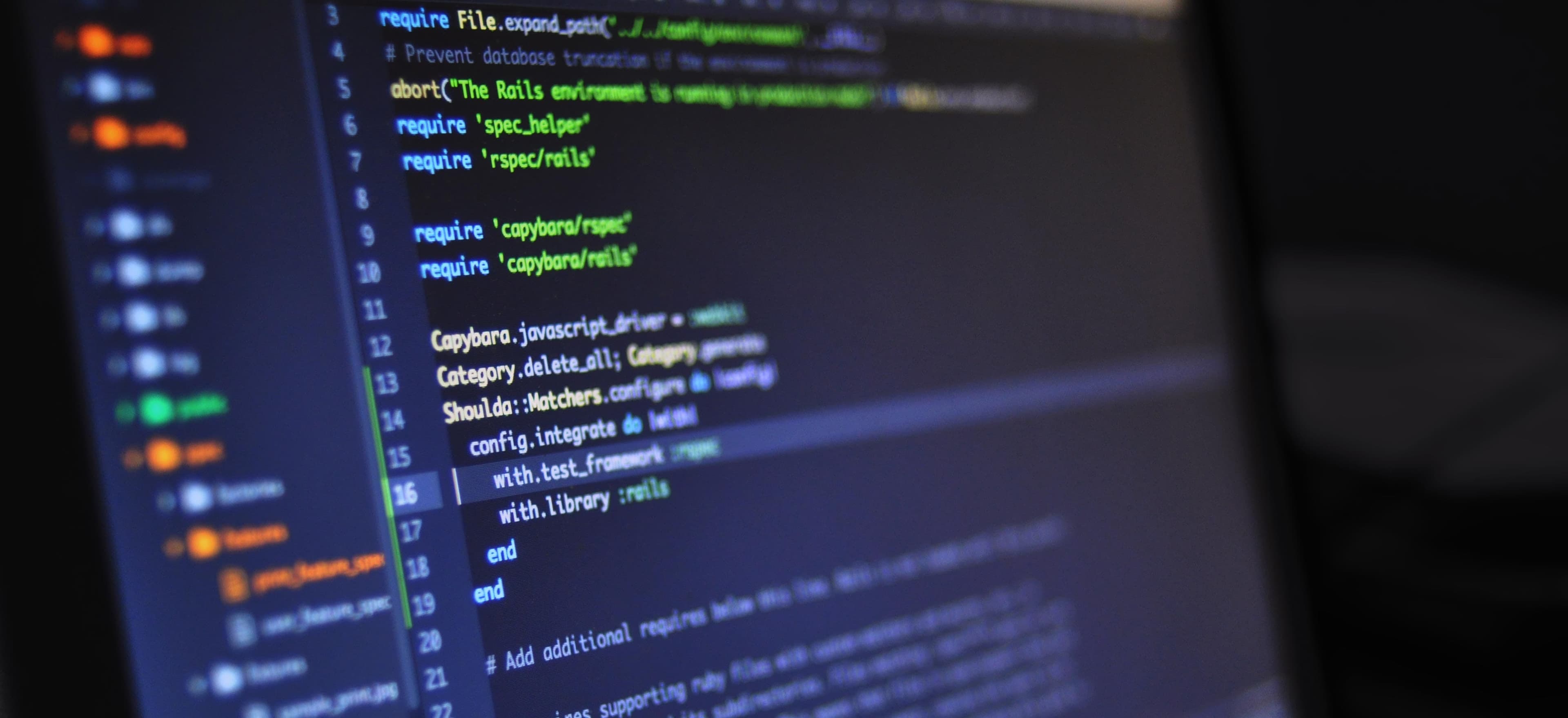
- Published on
Enhancing Big Data Analytics with Apache Arrow
In the world of big data analytics, efficiency is key. Processing large volumes of data requires tools capable of handling the workload without sacrificing speed or performance. Apache Arrow is a powerful in-memory columnar data format that serves as a foundation for accelerating analytic systems. In this article, we'll explore how Apache Arrow improves big data analytics, its key features, and how you can leverage it in your Java applications.
What is Apache Arrow?
Apache Arrow is a cross-language development platform for in-memory data. It specifies a standardized language-independent columnar memory format for flat and hierarchical data, organized for efficient analytical operations on modern hardware. By representing data in a columnar format, Arrow enables efficient data interchange across systems and languages. This not only reduces the overhead of converting data between different formats but also improves the performance of analytical operations significantly.
Key Features of Apache Arrow
1. In-Memory Representation
Apache Arrow provides in-memory representation of data, optimized for analytical processing. By organizing data in a columnar format, Arrow minimizes memory usage and maximizes CPU cache efficiency, thereby reducing the time and resources required for processing large datasets.
2. Cross-Language Support
Arrow is designed to provide seamless interoperability across various programming languages. It supports popular languages such as Java, C++, Python, and more, making it a versatile choice for data processing applications that involve multiple language ecosystems.
3. Zero-Copy Interoperability
One of the standout features of Apache Arrow is its zero-copy interop, allowing different systems and applications to share data without incurring additional memory overhead. This capability streamlines data exchange between components, leading to improved performance and reduced resource consumption.
4. Vectorized Processing
Arrow enables vectorized processing of data, which results in operations being performed on whole arrays of data at once, as opposed to element by element. This approach leverages modern CPU architectures and SIMD (Single Instruction, Multiple Data) instructions for efficient parallel processing.
5. GPU Acceleration
Apache Arrow includes support for GPU acceleration, which can significantly boost the performance of data-intensive tasks such as machine learning and scientific computing. By utilizing the parallel processing capabilities of GPUs, Arrow extends its potential for high-speed data processing.
Leveraging Apache Arrow in Java
Now that we understand the benefits of Apache Arrow, let's explore how we can leverage its capabilities in Java applications. Apache Arrow provides a dedicated Java library that enables seamless integration and utilization of Arrow's features within Java-based projects.
Setting Up Apache Arrow Java Library
To integrate Apache Arrow into a Java project, you can add the following Maven dependency to your pom.xml
file:
<dependency>
<groupId>org.apache.arrow</groupId>
<artifactId>arrow-vector</artifactId>
<version>5.0.0</version>
</dependency>
Replace the version with the latest stable release of the Apache Arrow Java library.
Working with Arrow Data Structures
Apache Arrow Java library offers various data structures, including VectorSchemaRoot
, Field
, VectorLoader
, and more, for managing and manipulating Arrow-formatted data. These structures provide a high-level interface for working with Arrow data, enabling seamless integration into existing Java data processing pipelines.
Example: Creating an Arrow Vector
Let's take a simple example of creating an Arrow IntVector
within a Java application:
import org.apache.arrow.vector.IntVector;
import org.apache.arrow.memory.RootAllocator;
// Create a root allocator
try (RootAllocator allocator = new RootAllocator(Long.MAX_VALUE)) {
// Create an IntVector with a capacity of 100
IntVector intVector = new IntVector("exampleIntVector", allocator);
intVector.allocateNew(100);
// Set values into the vector
for (int i = 0; i < 100; i++) {
intVector.set(i, i * 10);
}
// Do something with the populated vector
// ...
}
In this example, we use the Apache Arrow Java library to create an IntVector
, allocate memory for it, populate it with data, and perform subsequent operations. The code snippet demonstrates how Apache Arrow simplifies the manipulation of in-memory data structures within Java applications, fostering efficient data processing.
Integrating Arrow with Data Processing Frameworks
Apache Arrow can seamlessly integrate with popular Java-based data processing frameworks such as Apache Spark and Apache Flink. Leveraging Arrow within these frameworks enables enhanced interoperability, improved performance, and streamlined data processing workflows.
Example: Using Arrow in Apache Spark
import org.apache.spark.sql.SparkSession;
import org.apache.arrow.memory.RootAllocator;
import org.apache.arrow.adapter.spark.VectorContainer;
import org.apache.arrow.adapter.spark.VectorSchemaRoot;
import org.apache.arrow.vector.ipc.message.ArrowRecordBatch;
import org.apache.arrow.adapter.spark.SparkMemoryUtils;
SparkSession spark = SparkSession.builder()
.appName("ArrowIntegration")
.getOrCreate();
// Assume dataFrame is the input DataFrame
// Convert dataFrame to Arrow data
VectorSchemaRoot vectorSchemaRoot = SparkMemoryUtils.toArrow((Dataset<Row>) dataFrame);
VectorContainer vectorContainer = new VectorContainer(vectorSchemaRoot);
RootAllocator allocator = new RootAllocator(Long.MAX_VALUE);
// Perform operations using Arrow data
// ...
// Convert Arrow data back to DataFrame if needed
Dataset<Row> resultDataFrame = SparkMemoryUtils.fromArrow(vectorContainer.getValueVectors(), vectorContainer.getSchema());
// Perform further processing with resultDataFrame
In this example, we demonstrate how Apache Arrow seamlessly integrates with Apache Spark to convert data between native Spark DataFrames and Arrow in-memory format, enabling efficient data processing and seamless interoperability between the two frameworks.
Optimizing Data Serialization with Arrow
Apache Arrow's efficient in-memory representation can also significantly improve data serialization and deserialization tasks, especially when dealing with large volumes of data. Leveraging Arrow for serialization can lead to reduced overhead and improved throughput in data-intensive Java applications.
To Wrap Things Up
Apache Arrow stands as a pivotal technology for accelerating big data analytics by providing a language-independent, in-memory columnar data format. Its features, including cross-language support, zero-copy interop, vectorized processing, GPU acceleration, and seamless integration with Java, make it an invaluable asset for data processing applications.
By embracing Apache Arrow within Java applications, developers can unlock significant performance gains, improved interoperability, and streamlined data processing workflows. Whether it's enhancing data processing pipelines, integrating with popular data processing frameworks, or optimizing data serialization, Apache Arrow proves to be a game-changer in the realm of big data analytics, empowering developers to harness the full potential of modern hardware and architectures.
As big data continues to evolve and expand, Apache Arrow remains at the forefront, driving efficiencies and performance enhancements across diverse analytical systems and use cases.
In conclusion, Apache Arrow serves as a catalyst for innovation and optimization within the realm of big data analytics, and its seamless integration with Java opens new vistas for accelerating data processing applications, unlocking unprecedented efficiencies and performance gains.
For more information about Apache Arrow, you can visit the official Apache Arrow website and explore the rich documentation and resources available.
Try Apache Arrow in your Java projects and experience the transformative power of a columnar, in-memory data format that's tailored for modern data processing demands.