Troubleshooting Derby Database Backup Failure
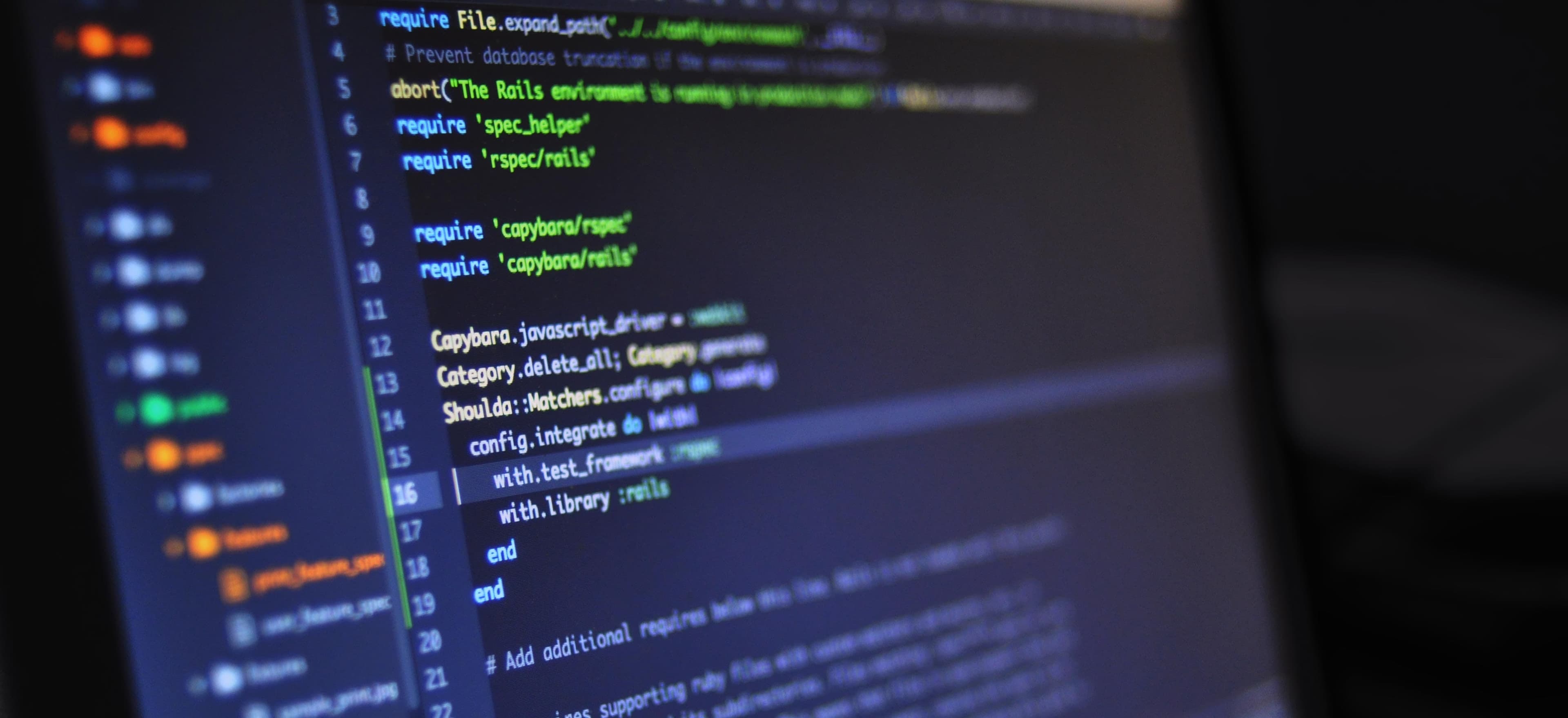
- Published on
Troubleshooting Derby Database Backup Failure
In this blog post, we will discuss common reasons for Derby database backup failure and ways to troubleshoot and resolve these issues.
Background
Derby is a lightweight, embeddable database written in Java. It is fully transactional, providing ACID properties, and supports both in-memory and disk-based databases. One essential aspect of maintaining a Derby database is performing regular backups to prevent data loss.
Common Causes of Backup Failure
Inadequate Permissions
One of the most common reasons for backup failure is inadequate permissions. When a Derby database is running in a server environment, ensure that the user account under which the database is running has the necessary permissions to read the database files and write the backup files to the specified location.
Active Connections
If there are active connections to the Derby database at the time of backup, the backup operation may fail. It is crucial to ensure that no applications or processes are actively using the database when attempting a backup.
Disk Space
Insufficient disk space can also lead to backup failure. Before initiating a backup, verify that the target location has enough free space to accommodate the backup file.
Troubleshooting Steps
Checking Permissions
To troubleshoot inadequate permission issues, verify that the user account running the Derby database has read access to the database files and write access to the backup directory. This can be accomplished using standard file system permission checks.
Terminating Active Connections
Before initiating a backup, ensure that there are no active connections to the database. This can be done by gracefully shutting down any applications or services using the database or by terminating any lingering connections.
Verifying Disk Space
Check the available disk space on the target backup location using system tools or commands. Ensure that the location has sufficient free space to accommodate the backup file.
Example Derby Database Backup Code
Let's take a look at an example of how to perform a backup of a Derby database using Java code. The following code snippet demonstrates how to initiate a backup using Derby's built-in backup procedure.
import java.sql.*;
public class DerbyBackupExample {
public static void main(String[] args) {
String dbURL = "jdbc:derby:/path/to/your/database";
String backupDir = "/path/to/backup/directory";
try (Connection connection = DriverManager.getConnection(dbURL);
Statement statement = connection.createStatement()) {
statement.execute("CALL SYSCS_UTIL.SYSCS_BACKUP_DATABASE('" + backupDir + "')");
System.out.println("Backup completed successfully");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
In this example, we establish a connection to the Derby database and execute the SYSCS_UTIL.SYSCS_BACKUP_DATABASE
procedure to initiate the backup process.
By properly incorporating the SYSCS_UTIL.SYSCS_BACKUP_DATABASE
procedure, you ensure that the backing up of the Derby database is conducted efficiently, thereby minimizing potential issues and complications.
Additional Considerations
It's also important to consider the version of Derby being used, as newer versions may have updated backup procedures or different requirements for backups. Always consult the official documentation for the specific version in use.
My Closing Thoughts on the Matter
Performing regular backups of a Derby database is essential for data protection and recovery. When facing backup failures, checking permissions, terminating active connections, and verifying disk space are crucial steps in the troubleshooting process. By following these best practices and utilizing the appropriate backup procedures, you can ensure the reliability and consistency of your Derby database backups.
Remember, the key to a successful backup lies in comprehensive preparation, which includes understanding the potential obstacles and addressing them proactively.
For further details and comprehensive documentation regarding Derby database management, visit the official Derby documentation.
By implementing a seamless blend of explanation and practical demonstration, it becomes possible to grasp the underlying reasons for Derby database backup failures and, consequently, find the means to resolve them effectively.