Debugging Java Applications: 5 Essential Tools
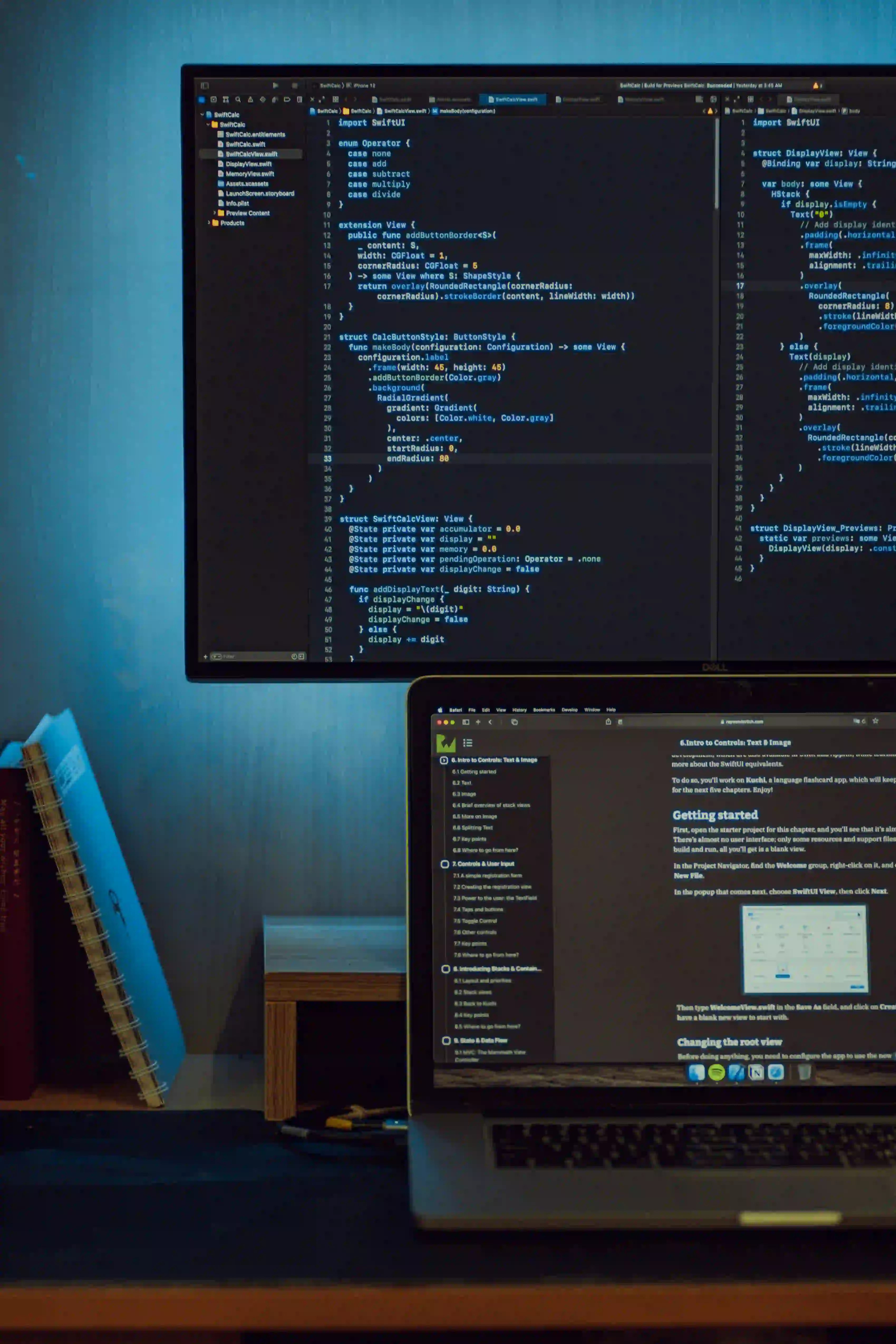
Debugging Java Applications: 5 Essential Tools
Debugging is an essential aspect of the software development process. In the world of Java development, having the right tools for debugging applications can streamline the process and make it more efficient. In this article, we will explore five essential tools for debugging Java applications. These tools are indispensable for identifying and fixing issues in Java code, making the development process smoother and more productive.
1. IntelliJ IDEA
IntelliJ IDEA is a popular integrated development environment (IDE) for Java developers. It comes with a powerful debugger that provides features such as breakpoints, step-by-step execution, variable inspection, and expression evaluation. The user-friendly interface of IntelliJ IDEA makes it easy to navigate through the code and identify bugs effectively. Additionally, IntelliJ IDEA integrates with build tools like Maven and Gradle, making it seamless to debug Java applications within the IDE.
Example:
public class DebugExample {
public static void main(String[] args) {
int x = 5;
int y = 10;
int result = x + y;
System.out.println("Result: " + result);
}
}
In the above code, you can set a breakpoint at the System.out.println
line to inspect the value of result
and understand how the variables x
and y
are being used.
2. Eclipse
Eclipse is another popular IDE among Java developers that provides a robust set of debugging tools. It offers features like breakpoints, watch expressions, and a detailed stack trace to track the flow of the program during execution. Eclipse also allows for remote debugging, enabling developers to debug applications running on different machines or environments.
3. JDB (Java Debugger)
JDB is a command-line debugger that comes with the Java Development Kit (JDK). While it may not have the visual appeal of an IDE, JDB is a powerful tool for debugging Java applications, especially in server environments where GUI-based tools may not be available. With JDB, developers can set breakpoints, examine variables, and control the execution flow of the program directly from the command line.
4. VisualVM
VisualVM is a visual tool that integrates with the Java Virtual Machine (JVM) and provides insights into the runtime behavior of Java applications. While it is primarily used for monitoring and profiling, VisualVM also offers a powerful debugging feature that allows developers to inspect heap dumps, analyze threads, and troubleshoot performance issues in real-time.
5. Apache JMeter
Apache JMeter is primarily known as a performance testing tool, but it also offers debugging capabilities for Java applications. With its sampler components and debugging controllers, JMeter can be utilized to simulate and debug various aspects of Java applications, making it a valuable tool for diagnosing performance-related issues and bottlenecks.
Final Considerations
Having the right set of debugging tools is crucial for every Java developer. Whether it's the feature-rich IntelliJ IDEA, the versatile Eclipse IDE, the command-line power of JDB, the runtime insights from VisualVM, or the performance testing and debugging capabilities of Apache JMeter, each tool plays a vital role in identifying and resolving issues in Java applications. By leveraging these essential tools, developers can debug their Java code effectively and deliver high-quality software solutions.
In conclusion, debugging is a critical skill for Java developers, and having the right tools at your disposal can make all the difference in the development process. With the tools mentioned in this article, developers can streamline their debugging workflow, identify issues efficiently, and ultimately build more robust and reliable Java applications. So, whether you prefer the convenience of an IDE or the flexibility of command-line tools, there is a debugging tool out there to suit your preferences and requirements.
Remember, effective debugging not only improves the quality of your code but also enhances your overall development experience. So, embrace these essential debugging tools and elevate your Java programming proficiency!