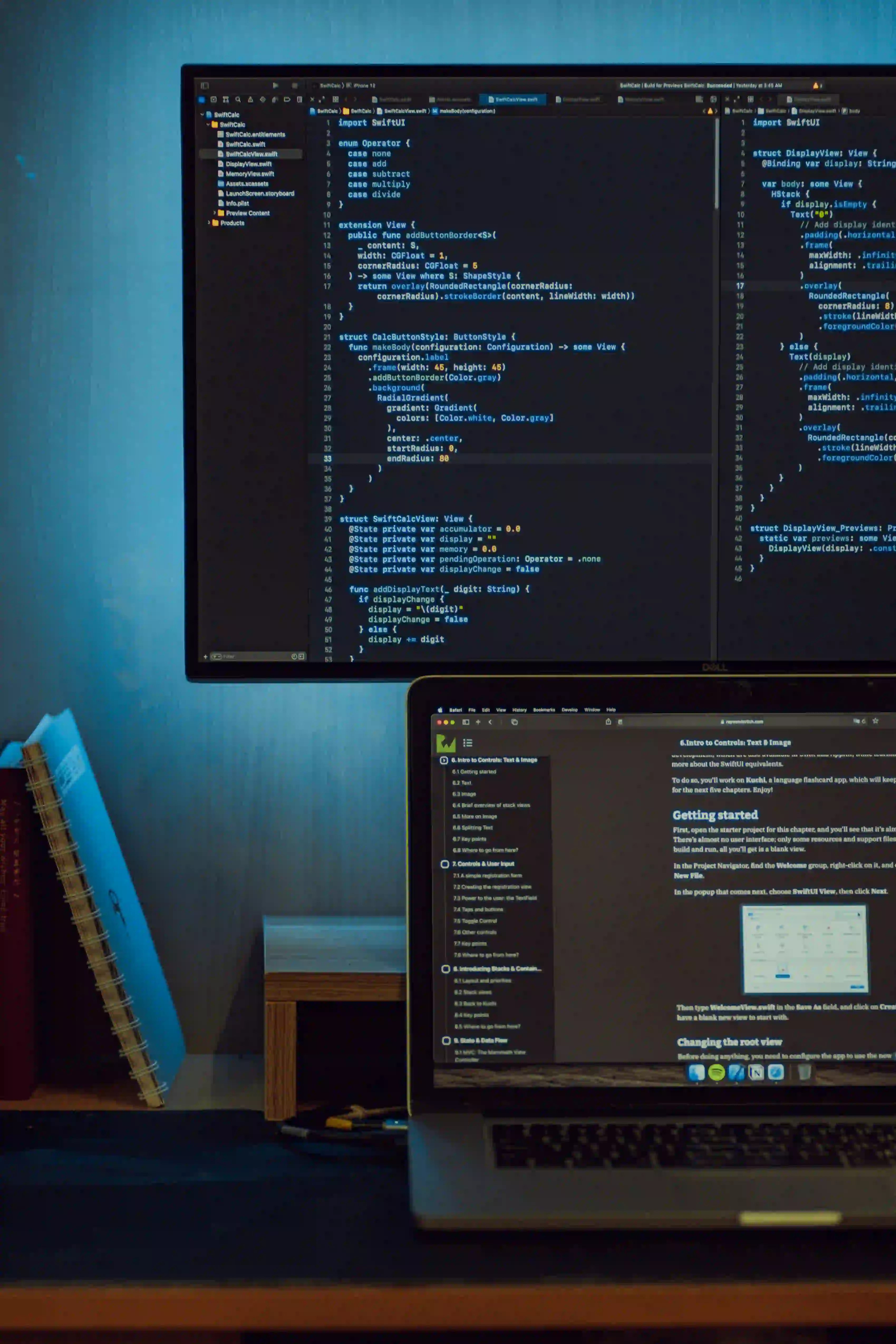
Handling Checkbox Processing in Spring MVC
When building a web application with Spring MVC, handling checkboxes in forms is a common and essential task. Checkboxes allow users to select one or more options from a set of choices. In this blog post, we will discuss how to handle checkbox processing in a Spring MVC application. We'll cover how to bind checkbox values to a model attribute, retrieve selected checkbox values, and process them in the backend. Let's dive in!
1. Creating a Form with Checkboxes
First, let's create a form with checkboxes in a JSP page. We'll use the <form:checkbox>
tag provided by Spring MVC to render checkboxes with their corresponding model attributes.
<form:form method="post" modelAttribute="checkboxForm">
<form:checkbox path="selectedValues" value="option1"/> Option 1
<form:checkbox path="selectedValues" value="option2"/> Option 2
<form:checkbox path="selectedValues" value="option3"/> Option 3
<input type="submit" value="Submit"/>
</form:form>
In this example, we have a form that binds to a model attribute checkboxForm
and renders three checkboxes for options 1, 2, and 3. The path="selectedValues"
attribute binds the selected checkbox values to the selectedValues
property of the model attribute.
2. Model Attribute and Controller Setup
Next, let's define the model attribute and the corresponding controller to handle form submission and process the selected checkbox values.
Model Attribute
public class CheckboxForm {
private List<String> selectedValues;
// Getters and setters
}
In the CheckboxForm
class, we have a selectedValues
property of type List<String>
to store the selected checkbox values.
Controller
@Controller
public class CheckboxController {
@RequestMapping(value = "/submitForm", method = RequestMethod.POST)
public String submitForm(@ModelAttribute("checkboxForm") CheckboxForm checkboxForm) {
List<String> selectedValues = checkboxForm.getSelectedValues();
// Process the selected checkbox values
// ...
return "result";
}
}
In the controller, we have a method submitForm
that handles the form submission. The @ModelAttribute("checkboxForm")
annotation binds the form data to the CheckboxForm
object. We can then retrieve the selected checkbox values from the CheckboxForm
object and process them as needed.
3. Retrieving and Processing Selected Checkbox Values
Now that we have the form and the controller set up, let's see how to retrieve and process the selected checkbox values from the CheckboxForm
object.
List<String> selectedValues = checkboxForm.getSelectedValues();
// Process the selected checkbox values
// ...
In the submitForm
method of the controller, we retrieve the selected checkbox values from the CheckboxForm
object using the getSelectedValues()
method. We can then perform any necessary processing based on the selected values.
Final Considerations
In this blog post, we discussed how to handle checkbox processing in a Spring MVC application. We created a form with checkboxes, defined a model attribute to store the selected values, and implemented a controller to handle form submission and process the selected checkbox values. By following these steps, you can effectively work with checkboxes in your Spring MVC web applications.
For further learning, you can explore more about Spring MVC and its form handling capabilities.
Thank you for reading! If you have any questions or thoughts on this topic, feel free to share them in the comments below.