Managing Complex Associations in Hibernate
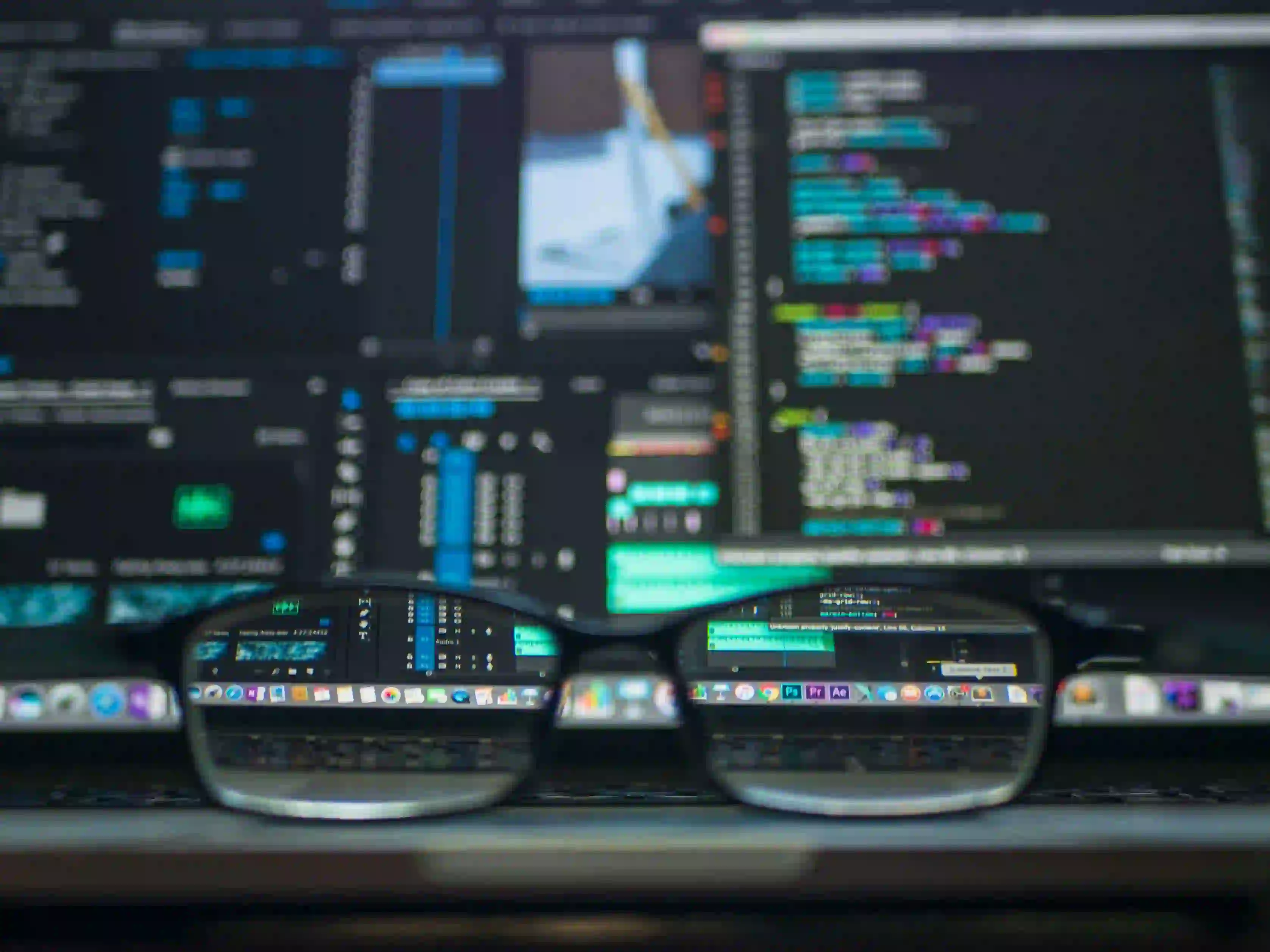
Understanding Complex Associations in Hibernate
When dealing with relational databases, managing complex associations between entities is a common challenge. Hibernate, a popular object-relational mapping (ORM) framework for Java, provides powerful tools to handle these complexities. In this post, we will explore how to effectively manage complex associations in Hibernate, including one-to-one, one-to-many, and many-to-many relationships. We will also cover best practices and potential pitfalls to avoid.
One-to-One Relationships
Example Scenario
Consider a scenario where we have two entities: Employee
and Address
. Each employee has one address, and each address is associated with only one employee.
Mapping in Hibernate
@Entity
public class Employee {
@Id
private Long id;
// Other fields
@OneToOne(cascade = CascadeType.ALL)
@JoinColumn(name = "address_id")
private Address address;
// Getters and setters
}
@Entity
public class Address {
@Id
private Long id;
// Other fields
// Getters and setters
}
In this example, we use the @OneToOne
annotation to establish the relationship between Employee
and Address
. The cascade = CascadeType.ALL
attribute ensures that any operations performed on an employee will cascade to its associated address.
One-to-Many Relationships
Example Scenario
Let’s consider an example where an Order
can have multiple LineItems
. Each LineItem
represents a product within that order.
Mapping in Hibernate
@Entity
public class Order {
@Id
private Long id;
// Other fields
@OneToMany(cascade = CascadeType.ALL, mappedBy = "order")
private List<LineItem> lineItems = new ArrayList<>();
// Getters and setters
}
@Entity
public class LineItem {
@Id
private Long id;
// Other fields
@ManyToOne
@JoinColumn(name = "order_id")
private Order order;
// Getters and setters
}
In this example, we use the @OneToMany
and @ManyToOne
annotations to establish the one-to-many relationship between Order
and LineItem
. The mappedBy
attribute in the @OneToMany
annotation specifies the field in the LineItem
class that owns the relationship.
Many-to-Many Relationships
Example Scenario
Imagine a scenario where Student
entities can enroll in multiple Course
entities, and each Course
can have multiple students.
Mapping in Hibernate
@Entity
public class Student {
@Id
private Long id;
// Other fields
@ManyToMany(cascade = { CascadeType.ALL })
@JoinTable(
name = "student_course",
joinColumns = { @JoinColumn(name = "student_id") },
inverseJoinColumns = { @JoinColumn(name = "course_id") }
)
private Set<Course> courses = new HashSet<>();
// Getters and setters
}
@Entity
public class Course {
@Id
private Long id;
// Other fields
@ManyToMany(mappedBy = "courses")
private Set<Student> students = new HashSet<>();
// Getters and setters
}
In this example, we use the @ManyToMany
annotation to establish the many-to-many relationship between Student
and Course
. The @JoinTable
annotation is used to define the mapping table that holds the foreign key references to both entities.
Best Practices and Pitfalls to Avoid
Best Practices
- Understand the Entity Relationships: Before defining the associations, have a clear understanding of the relationships between entities in your domain model.
- Use Cascade Types Wisely: Cascade types should be used judiciously to prevent unintended side effects. Consider the impact of cascading operations on the associated entities.
- Indexing and Fetch Strategies: For performance optimization, consider indexing columns involved in associations and carefully choose fetch strategies based on your application’s use cases.
Pitfalls to Avoid
- N+1 Query Problem: Be mindful of the N+1 query problem when fetching entities with associations. Consider using fetch joins or batch fetching to alleviate this issue.
- Circular References: Avoid circular references between entities, as they can lead to infinite loops during serialization and deserialization.
Wrapping Up
In this post, we explored how to manage complex associations in Hibernate, covering one-to-one, one-to-many, and many-to-many relationships. By understanding the mapping strategies and best practices, you can effectively model and manage complex associations within your Hibernate-based applications. As with any ORM framework, it’s essential to weigh the trade-offs and choose the most suitable association mapping based on your specific application requirements.
As you delve into Hibernate and explore its powerful association management capabilities, always remember to keep your domain model clear and your mappings optimized for performance. Now, armed with this knowledge, go forth and master the art of managing complex associations with Hibernate!